How to Clear ListBox in C#
-
Clear ListBox With the
ListBox.Items.Clear()
Function inC#
-
Clear ListBox With the
DataSource = null
Approach inC#
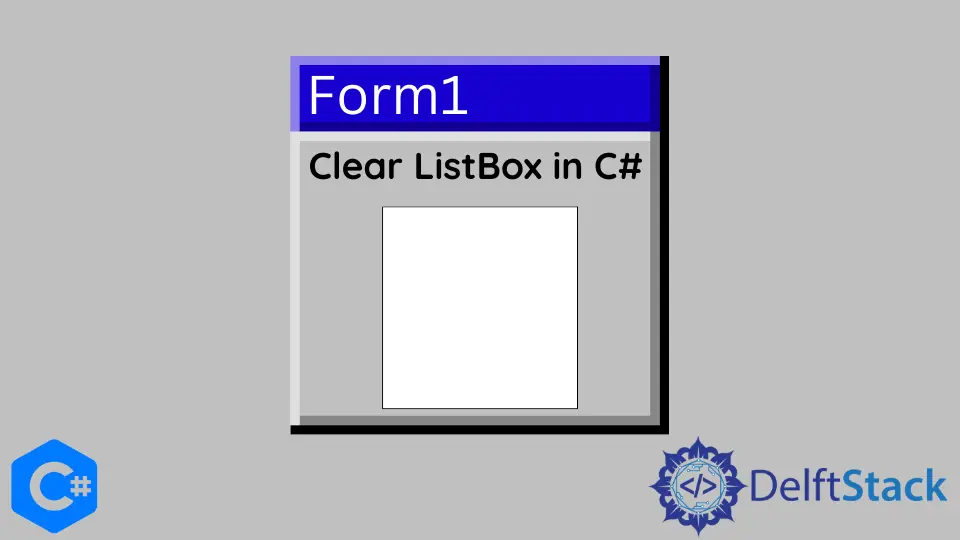
This tutorial will discuss the methods of clearing all the contents of a list box in C#.
Clear ListBox With the ListBox.Items.Clear()
Function in C#
The ListBox.Items.Clear()
function clears all the items inside a list box in C#. This function does not return anything, and all the information related to the deleted elements is lost. The following code example shows us how to empty a list box with the ListBox.Items.Clear()
function in C#.
private void emptylistbox(object sender, EventArgs e) {
listbox1.Items.Clear();
}
In the above code, we emptied the list box listbox1
with the listbox1.Items.Clear()
function in C#. Although this approach is good and works just fine with the simple list box. But if our list box is bound to a data source, this approach will not work and show an error. This error can be easily fixed, as shown in the next section.
Clear ListBox With the DataSource = null
Approach in C#
If our list box is bound to a data source, we can assign a null
value to the data source to empty our list box. But this is not a very good approach because we might need to use the same data source later in our code. The best solution for this would be to specify the ListBox.DataSource
property equal to null
to remove the data source and then use the ListBox.Items.Clear()
function to clear the previous items in the list box. The following code example shows us how we can empty a list box with the ListBox.DataSource
property in C#.
private void emptylistbox(object sender, EventArgs e) {
listbox1.DataSource = null;
listbox1.Items.Clear();
}
In the above code, we emptied the list box listbox1
with the the listbox1.DataSource = null
and listbox1.Items.Clear()
function in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn