How to Change Cursor in C#
- Method 1: Using Windows Forms
- Method 2: Using WPF (Windows Presentation Foundation)
- Method 3: Asynchronous Operations
- Conclusion
- FAQ
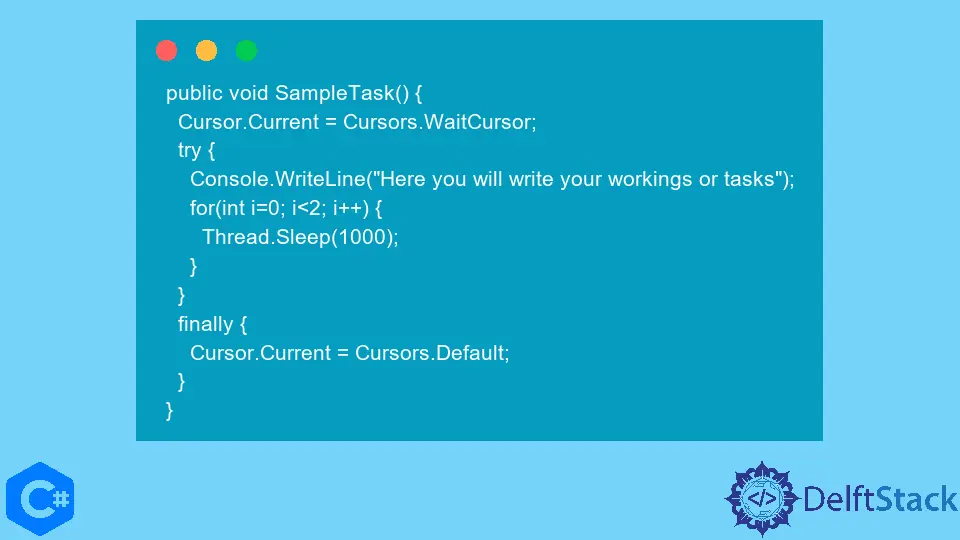
Changing the cursor in a C# application can enhance user experience significantly. Whether you are developing a desktop application or a web application, providing visual feedback through cursor changes is essential.
In this article, we will focus on how to change the cursor to a wait cursor using C#. This is particularly useful when performing long-running tasks, as it informs users that they should wait for the operation to complete. You will learn step-by-step methods to implement this, complete with code examples and detailed explanations. Let’s dive into the world of C# and see how easy it is to change the cursor!
Method 1: Using Windows Forms
If you’re working with a Windows Forms application, changing the cursor is quite straightforward. The Cursor.Current
property allows you to set the current cursor for the application. Here’s how you can implement this:
using System;
using System.Drawing;
using System.Windows.Forms;
public class CursorExample : Form
{
public CursorExample()
{
this.Load += new EventHandler(Form_Load);
}
private void Form_Load(object sender, EventArgs e)
{
Cursor.Current = Cursors.WaitCursor;
// Simulate a long-running task
System.Threading.Thread.Sleep(5000);
Cursor.Current = Cursors.Default;
}
[STAThread]
public static void Main()
{
Application.EnableVisualStyles();
Application.Run(new CursorExample());
}
}
Output:
The cursor changes to a wait cursor for 5 seconds before reverting to the default cursor.
In this code snippet, we create a simple Windows Form application. When the form loads, we set the cursor to a wait cursor. The System.Threading.Thread.Sleep(5000)
simulates a long-running task for 5 seconds. After this period, we revert the cursor back to the default. This method provides a clear indication to users that an operation is in progress, improving the overall user experience.
Method 2: Using WPF (Windows Presentation Foundation)
For those working with WPF applications, the approach is slightly different but equally effective. The Mouse.OverrideCursor
property can be used to set a wait cursor. Here’s an example:
using System;
using System.Threading;
using System.Windows;
namespace CursorExampleWPF
{
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
Loaded += MainWindow_Loaded;
}
private void MainWindow_Loaded(object sender, RoutedEventArgs e)
{
Mouse.OverrideCursor = Cursors.Wait;
// Simulate a long-running task
Thread.Sleep(5000);
Mouse.OverrideCursor = null;
}
}
}
Output:
The cursor changes to a wait cursor for 5 seconds before reverting to the default cursor.
In this WPF example, we utilize the Mouse.OverrideCursor
property to change the cursor to a wait cursor. Similar to the Windows Forms example, we simulate a long-running task with Thread.Sleep(5000)
. After the task, we set the Mouse.OverrideCursor
back to null
, which restores the default cursor. This method is particularly useful in WPF applications, allowing for a seamless user experience during lengthy operations.
Method 3: Asynchronous Operations
If your application performs tasks asynchronously, you can combine cursor changes with asynchronous programming for a smoother experience. Here’s how you can implement this in a Windows Forms application:
using System;
using System.Threading.Tasks;
using System.Windows.Forms;
public class AsyncCursorExample : Form
{
public AsyncCursorExample()
{
this.Load += new EventHandler(Form_Load);
}
private async void Form_Load(object sender, EventArgs e)
{
Cursor.Current = Cursors.WaitCursor;
await Task.Delay(5000); // Simulate a long-running task
Cursor.Current = Cursors.Default;
}
[STAThread]
public static void Main()
{
Application.EnableVisualStyles();
Application.Run(new AsyncCursorExample());
}
}
Output:
The cursor changes to a wait cursor for 5 seconds before reverting to the default cursor.
In this asynchronous example, we use await Task.Delay(5000)
instead of blocking the UI thread with Thread.Sleep
. This allows the application to remain responsive while waiting. When the form loads, the cursor changes to a wait cursor, and after the delay, it reverts back to the default. This method is particularly advantageous in modern applications, as it keeps the user interface responsive and user-friendly.
Conclusion
Changing the cursor in C# applications is a simple yet effective way to enhance user experience. Whether you’re using Windows Forms or WPF, the methods outlined in this article provide straightforward solutions for implementing a wait cursor during long-running tasks. By giving users visual feedback, you can help them understand that an operation is in progress, reducing frustration and improving overall satisfaction. Remember to choose the method that best fits your application’s architecture, and happy coding!
FAQ
-
How do I change the cursor in a WPF application?
You can use the Mouse.OverrideCursor property to change the cursor in a WPF application. -
Can I change the cursor back to default after a task?
Yes, you can revert the cursor to its default state by setting the cursor property back to null or Cursors.Default. -
What is the purpose of using a wait cursor?
A wait cursor indicates to users that a task is in progress, helping to manage their expectations and improve user experience. -
Is it possible to change the cursor dynamically?
Yes, you can change the cursor dynamically based on application events or user actions. -
How can I ensure my application remains responsive while changing the cursor?
Using asynchronous programming, such as async/await, allows your application to remain responsive while performing long-running tasks.
#. You will learn effective methods for implementing this in both Windows Forms and WPF applications. Enhance user experience by providing visual feedback during long-running tasks. Discover code examples and detailed explanations that make it easy to implement cursor changes in your C# applications.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn