How to Catch Multiple Exceptions in C#
-
Catch Multiple Exceptions With the
Exception
Class inC#
-
Catch Multiple Exceptions With the
if
Statement inC#
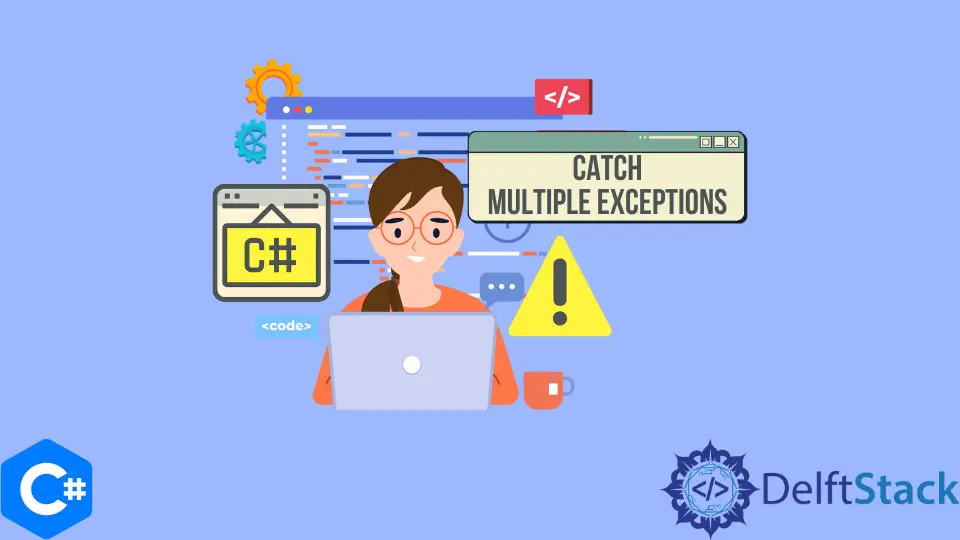
This tutorial will discuss the methods to catch multiple exceptions in C#.
Catch Multiple Exceptions With the Exception
Class in C#
The Exception
class is used to represent a general exception in C#. We can use the Exception
class inside the try...catch
clause to catch any type of exception thrown by the code. See the following example code.
using System;
namespace convert_int_to_bool {
class Program {
static void Main(string[] args) {
try {
int i = 1;
bool b = Convert.ToBoolean(i);
Console.WriteLine(b);
} catch (Exception e) {
Console.WriteLine("An Exception Occured");
}
}
}
}
Output:
True
In the above code, we catch any type of exception thrown by our code with the Exception
class in C#. This approach is generally discouraged because it does not give us enough information about the problem and how to troubleshoot it. We should always prefer specific exception types rather than this generic one.
Catch Multiple Exceptions With the if
Statement in C#
Using specific exceptions requires us to write a lot of code in the form of catch
clauses. We can use the if
statement to catch multiple types of exceptions with just one catch
clause in C#. See the following example code.
using System;
namespace convert_int_to_bool {
class Program {
static void Main(string[] args) {
try {
int i = 1;
bool b = Convert.ToBoolean(i);
Console.WriteLine(b);
} catch (Exception e) {
if (ex is FormatException || ex is OverflowException) {
Console.WriteLine("Format or Overflow Exception occured");
}
}
}
}
}
Output:
True
In the above code, we catch both FormatException
and OverflowException
exceptions with just one catch
clause using an if
statement in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn