C# Capitalize First Letter
-
Use the
ToUpper()
Method to Capitalize the First Letter of a String inC#
-
Use the
regex
Expressions to Capitalize the First Letter of a String inC#
-
Use the
ToCharArray()
to Capitalize the First Letter of a String inC#
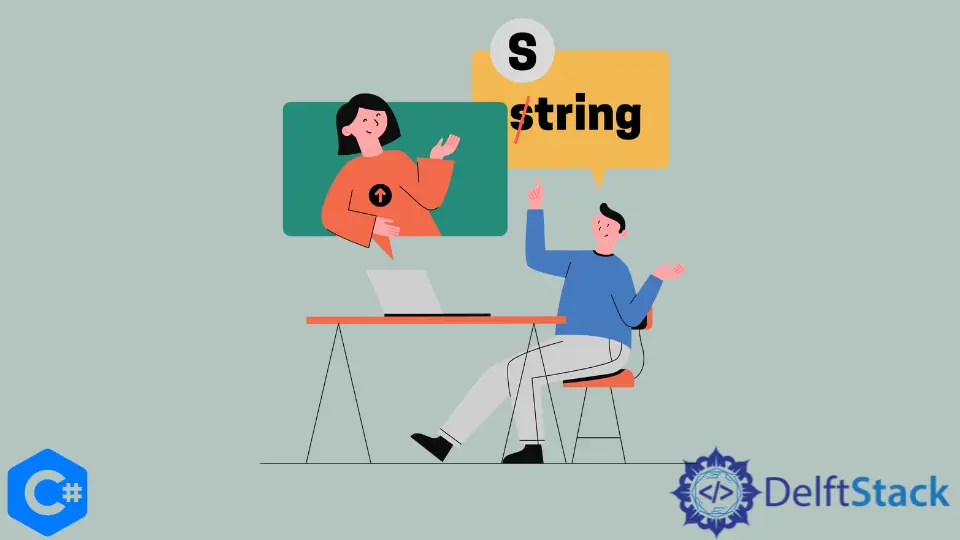
In this tutorial, we will look at multiple ways how to capitalize the first letter of a string in C#.
Use the ToUpper()
Method to Capitalize the First Letter of a String in C#
using System;
class Program {
static void Main() {
string str = "delftstack";
if (str.Length == 0) {
Console.WriteLine("Empty String");
} else if (str.Length == 1) {
Console.WriteLine(char.ToUpper(str[0]));
} else {
Console.WriteLine(char.ToUpper(str[0]) + str.Substring(1));
}
}
}
In this approach, we capitalize the first letter of string using the ToUpper()
method with corner cases like the string being empty or the string being length 1
.
Use the regex
Expressions to Capitalize the First Letter of a String in C#
using System;
using System.Text.RegularExpressions;
class Program {
static public string UpperCaseFirstChar(string text) {
return Regex.Replace(text, "^[a-z]", m => m.Value.ToUpper());
}
static void Main() {
string str = "delftstack";
Console.WriteLine(UpperCaseFirstChar(str));
}
}
Output:
Delftstack
In the above method, we use the regex expression to select the desired character and then replacement with its capitalized form.
Use the ToCharArray()
to Capitalize the First Letter of a String in C#
using System;
class Program {
public static string UpperCaseFirstChar(string s) {
if (string.IsNullOrEmpty(s)) {
return string.Empty;
}
char[] a = s.ToCharArray();
a[0] = char.ToUpper(a[0]);
return new string(a);
}
public static void Main() {
Console.WriteLine(UpperCaseFirstChar("delftstack"));
}
}
Output:
Delftstack
In this approach, we capitalize the first letter of string by capturing it in a character array and then replacing the first character with its capital counterpart. Once the replacement is performed, we form a new string using the modified character array.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn