C# Binary Literals
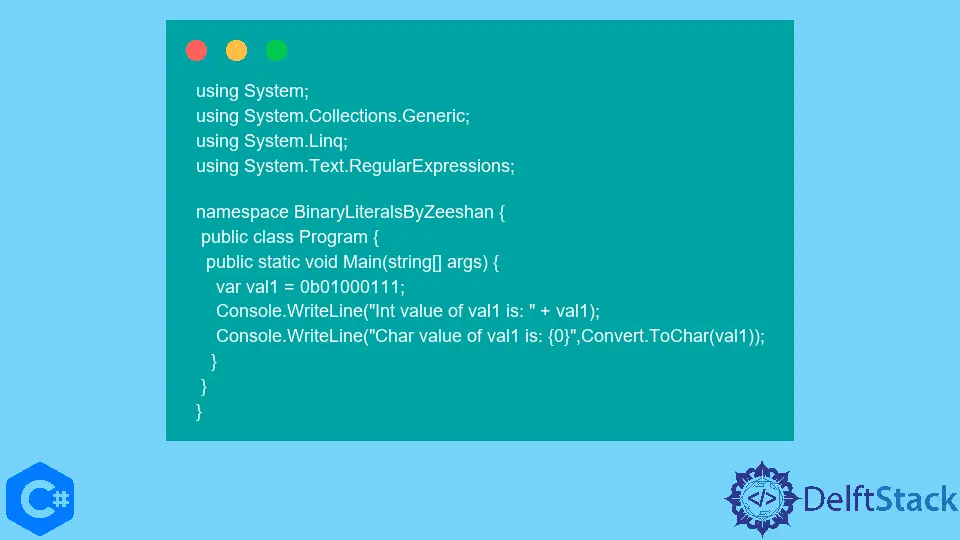
Programming languages evolve, and C# is no exception. One of the exciting features introduced in C# 7.0 is the ability to use binary literals. This enhancement allows developers to express binary numbers directly in their code, making it easier to work with bitwise operations and low-level programming.
In this article, we will explore how to write binary literals in C#, providing you with practical examples and insights. Whether you’re a seasoned developer or just starting, understanding binary literals can enhance your coding efficiency and clarity. Let’s dive into the world of binary literals in C# and see how they can simplify your programming tasks.
Understanding Binary Literals
Binary literals are a way to represent numbers in base-2 format, which consists only of two digits: 0 and 1. In C#, binary literals are prefixed with 0b
or 0B
, making them easy to identify. For instance, the binary number 1010
can be represented as 0b1010
in C#. This feature is particularly useful when working with bitwise operations, as it allows developers to see the exact bit patterns they are manipulating.
Using binary literals can make your code more readable, especially when dealing with flags or configurations that rely on specific bits. Instead of converting binary to decimal and back again, you can write your numbers in binary directly, which reduces the chances of errors and improves code clarity.
Writing Binary Literals in C#
To write binary literals in C#, you simply need to use the 0b
prefix followed by your binary number. Let’s look at some examples to illustrate this.
Example 1: Basic Binary Literal
Here’s a simple example of defining a binary literal in C#.
int binaryValue = 0b1010;
Console.WriteLine(binaryValue);
Output:
10
In this example, we define an integer variable binaryValue
and assign it the binary literal 0b1010
. When we print this value, it outputs 10
, which is the decimal equivalent of the binary number.
Example 2: Using Binary Literals with Bitwise Operations
Binary literals become even more powerful when combined with bitwise operations. Here’s an example demonstrating this.
int a = 0b1100; // 12 in decimal
int b = 0b1010; // 10 in decimal
int result = a & b;
Console.WriteLine(result);
Output:
8
In this case, we define two binary literals, a
and b
, and perform a bitwise AND operation. The result of 0b1100 & 0b1010
is 0b1000
, which is 8
in decimal. This showcases how binary literals can simplify bitwise operations, making your code more intuitive.
Example 3: Combining Multiple Binary Literals
You can also combine multiple binary literals for more complex operations. Here’s how it works.
int flags = 0b0010 | 0b1000; // Combining flags
Console.WriteLine(flags);
Output:
10
In this example, we use the bitwise OR operator (|
) to combine two binary literals. The result is 0b1010
, which equals 10
in decimal. This is particularly useful when managing multiple flags in applications, as it allows you to set or clear specific bits easily.
Conclusion
Binary literals in C# offer a powerful way to work with binary numbers directly in your code. By using the 0b
prefix, you can enhance the readability and maintainability of your programs, particularly when dealing with bitwise operations. As you integrate binary literals into your coding practices, you will likely find that they simplify your logic and reduce the likelihood of errors. Embracing this feature can lead to more efficient and clear code, ultimately improving your programming experience in C#.
FAQ
-
What is a binary literal in C#?
A binary literal in C# is a way to represent numbers in base-2 format, prefixed with0b
or0B
. -
When was binary literals introduced in C#?
Binary literals were introduced in C# 7.0. -
How do you perform bitwise operations using binary literals?
You can perform bitwise operations like AND, OR, and XOR on binary literals just like any other integer values. -
Can binary literals be used for floating-point numbers?
No, binary literals in C# are only applicable for integral types likeint
,long
,byte
, etc.
- Why should I use binary literals?
Using binary literals enhances code readability and reduces the chances of errors when dealing with bitwise operations.
#. Learn about the benefits of binary literals, see practical examples, and understand how they can simplify bitwise operations in your C# programming. Whether you’re a beginner or an experienced developer, this guide will enhance your coding skills.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn