Array of Lists in C#
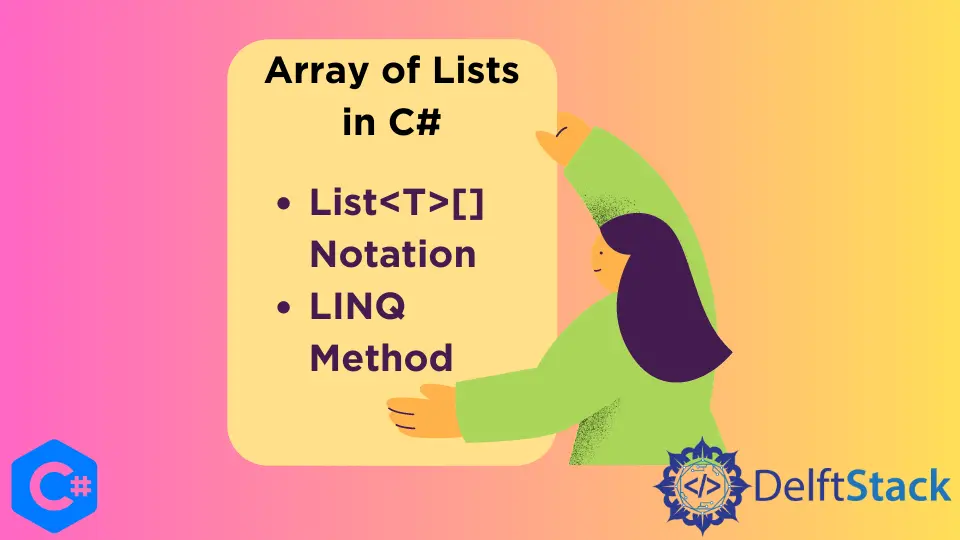
This tutorial will discuss the methods to create an array of lists in C#.
Array of Lists With the List<T>[]
Notation in C#
The List<T>[]
notation can be used to declare an array of lists of type T
in C#. Keep in mind that this will only declare an array of null references. We still have to initialize each list at each index of the List<T>[]
with the new
keyword.
using System;
using System.Collections.Generic;
namespace array_of_lists {
class Program {
static void Main(string[] args) {
List<int>[] arrayList = new List<int>[3];
arrayList[0] = new List<int> { 1, 2, 3 };
arrayList[1] = new List<int> { 4, 5, 6 };
arrayList[2] = new List<int> { 7, 8, 9 };
foreach (var list in arrayList) {
foreach (var element in list) {
Console.WriteLine(element);
}
}
}
}
}
Output:
1
2
3
4
5
6
7
8
9
In the above code, we declared and initialized the array of lists arrayList
containing integer values with the List<T>[]
notation and new
keyword in C#. The above approach is ok for small arrays. But, if we have a large array, this approach can become very labor-intensive and can take a lot of code. This approach is only suitable for arrays with a smaller length.
Array of Lists With the LINQ Method in C#
The LINQ is used for integrating query functionality with the data structures in C#. We can use LINQ to declare and initialize an array of lists in C#.
using System;
using System.Collections.Generic;
namespace array_of_lists {
class Program {
static void Main(string[] args) {
List<int>[] arrayList = new List<int> [3].Select(item => new List<int> { 1, 2, 3 }).ToArray();
foreach (var list in arrayList) {
foreach (var element in list) {
Console.WriteLine(element);
}
}
}
}
}
Output:
1
2
3
1
2
3
1
2
3
In the above code, we declared and initialized the array of lists arrayList
containing integer values with the List<T>[]
notation and new
keyword in C#. This method is less labor-intensive than the previous example for larger arrays, so it is more suitable for arrays with a larger length.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn