The Use of += in C#
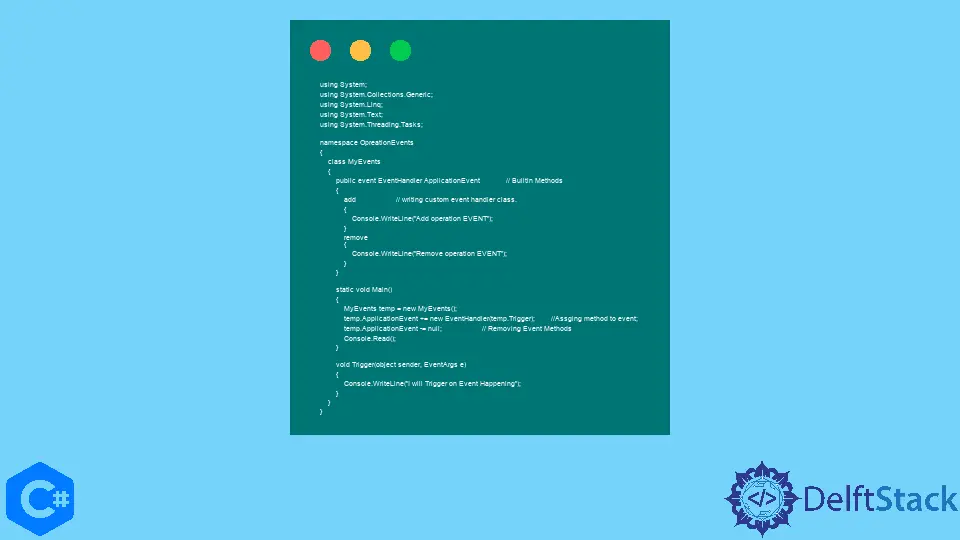
This guide will learn about the +=
operator in C#. +=
in C# is different from in other programming languages.
It can also be used as the assignment operator, just like all other languages. We’ll see what its other use in C#.
Let’s dive in.
Use the +=
as an EventHandler
in C#
In the C# language, the operator +=
specifies the method supposed to be called in response to an event.
These types of methods are also known as event handlers. An EventHandler
is a class in C# featuring built-in methods.
In the code below, we have made a custom event handler class. Take a look.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace OpreationEvents {
class MyEvents {
public event EventHandler ApplicationEvent // Builtin Methods
{
add // writing custom event handler class.
{
Console.WriteLine("Add operation EVENT");
}
remove {
Console.WriteLine("Remove operation EVENT");
}
}
static void Main() {
MyEvents temp = new MyEvents();
temp.ApplicationEvent += new EventHandler(temp.Trigger); // Assging method to event;
temp.ApplicationEvent -= null; // Removing Event Methods
Console.Read();
}
void Trigger(object sender, EventArgs e) {
Console.WriteLine("I will Trigger on Event Happening");
}
}
}
The void
method trigger()
is assigned to the event in the above code. It will trigger the trigger()
function whenever that event occurs.
We can also use the -=
operator to remove the operation event. It’s the opposite of what we have learned in the +=
operator.
Output:
Add operation EVENT
Remove operation EVENT
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn