How to Count Occurrences of a Character Inside a String in C#
-
Count Occurrences of a Character in a String With the Linq Method in
C#
-
Count Occurrences of a Character in a String With the
String.Split()
Method inC#
-
Count Occurrences of a Character in a String With the
foreach
Loop inC#
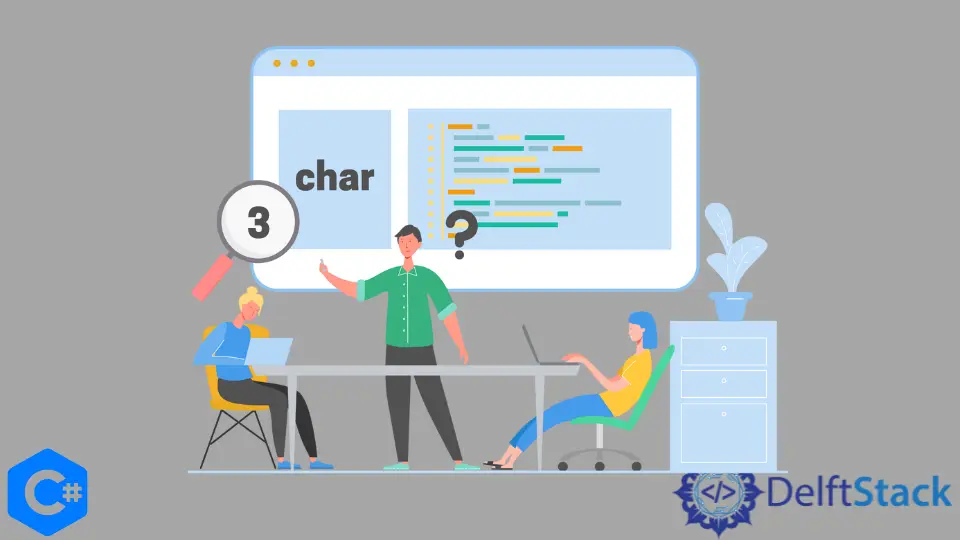
This tutorial will introduce the methods to get the number of occurrences of a character in a string variable in C#.
Count Occurrences of a Character in a String With the Linq Method in C#
Linq
integrates SQL functionalities on data structures in C#. The following code example shows us how we can get the number of occurrences of a character in a string with the Linq method in C#.
using System;
using System.Linq;
namespace count_occurrences_of_a_char_in_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = source.Count(f => f == 'o');
Console.WriteLine(count);
}
}
}
Output:
2
In the above code, we calculated the number of occurrences of the character o
in the string variable source
with the Linq method in C#.
Count Occurrences of a Character in a String With the String.Split()
Method in C#
The String.Split()
method splits a string into multiple substrings based on a separator in C#. The String.Split(x)
method would return 1 string more than the number of occurrences of x
in the string. We can count the number of strings returned by the String.Split()
method and subtract 1 from it to get the number of occurrences of the character inside the main-string. The following code example shows us how we can count the number of occurrences of a character in a string variable with the String.Split()
method in C#.
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = source.Split('o').Length - 1;
Console.WriteLine(count);
}
}
}
Output:
2
In the above code, we calculated the number of occurrences of the character o
in the string variable source
with the String.Split()
function in C#.
Count Occurrences of a Character in a String With the foreach
Loop in C#
The foreach
loop is used to iterate through a data structure in C#. We can use the foreach
loop to iterate through each character of our string variable and check whether the character matches our desired character with an if
statement in C#. The following code example shows us how we can count the number of occurrences of a character inside a string with the foreach
loop in C#.
using System;
using System.Linq;
namespace get_first_char_of_string {
class Program {
static void Main(string[] args) {
string source = "/once/upon/a/time/";
int count = 0;
foreach (char c in source) {
if (c == 'o') {
count++;
}
}
Console.WriteLine(count);
}
}
}
Output:
2
In the above code, we calculated the number of occurrences of the character o
in the string variable source
with the foreach
loop in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#