How to Convert String to JSON Object in C#
- Understanding JSON and C#
- Using JObject.Parse() to Convert String to JSON Object
- Handling Exceptions During Parsing
- Accessing Nested JSON Objects
- Modifying JSON Objects
- Conclusion
- FAQ
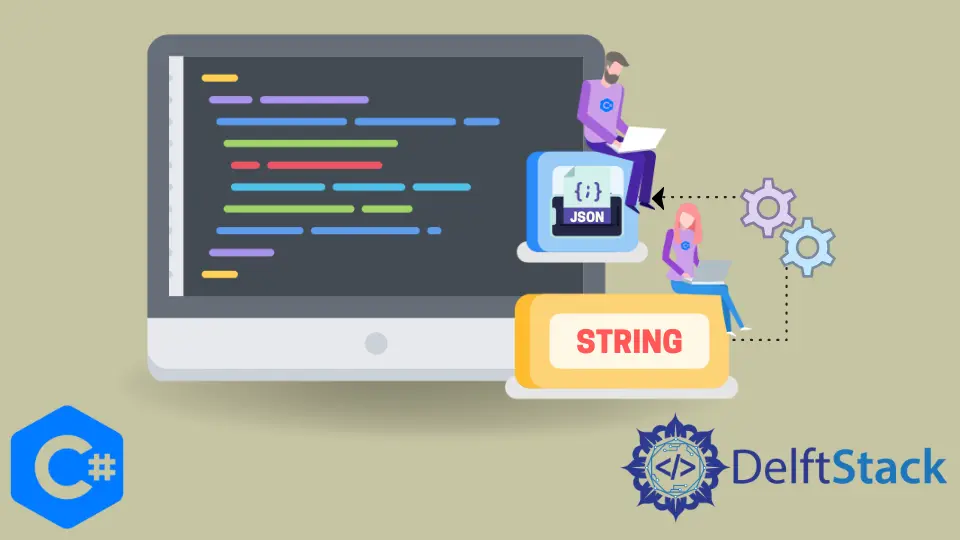
When working with C#, developers often encounter scenarios where they need to convert strings into JSON objects. This is particularly useful when dealing with APIs, configuration files, or any data interchange format. One of the most straightforward ways to perform this conversion in C# is by using the JObject.Parse()
function from the popular Newtonsoft.Json library, also known as Json.NET. This function allows you to easily parse a JSON-formatted string and convert it into a JObject, which can then be manipulated or accessed in your application.
In this article, we will explore the steps to convert a string to a JSON object in C#, along with practical examples to help you understand the process better.
Understanding JSON and C#
JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write. It is also easy for machines to parse and generate. In C#, JSON is often used for data serialization and deserialization. The Newtonsoft.Json library provides a robust framework for working with JSON data in C#. By using JObject.Parse()
, you can convert a JSON string into a JObject, which represents a JSON object in C#. This opens up a world of possibilities for data manipulation, enabling you to access properties, iterate through arrays, and much more.
Using JObject.Parse() to Convert String to JSON Object
To convert a string to a JSON object in C#, you first need to ensure that you have the Newtonsoft.Json library installed. You can do this via NuGet Package Manager in Visual Studio. Once you have the library set up, you can use the JObject.Parse()
method to convert your string into a JSON object.
Here’s a simple example:
using Newtonsoft.Json.Linq;
class Program
{
static void Main()
{
string jsonString = "{\"name\":\"John\", \"age\":30, \"city\":\"New York\"}";
JObject jsonObject = JObject.Parse(jsonString);
Console.WriteLine(jsonObject["name"]);
Console.WriteLine(jsonObject["age"]);
Console.WriteLine(jsonObject["city"]);
}
}
Output:
John
30
New York
In this example, we define a JSON string that contains basic information about a person. The JObject.Parse()
method takes this string and converts it into a JObject. We can then access the properties of the JObject using the indexer syntax, allowing us to retrieve values like the name, age, and city of the individual.
Handling Exceptions During Parsing
When converting a string to a JSON object, it’s important to handle potential exceptions that may arise, especially if the string is not formatted correctly. The JObject.Parse()
method throws a JsonReaderException
if the input string is not valid JSON. To manage this, you can implement a try-catch block around your parsing code.
Here’s how you can do this:
using Newtonsoft.Json.Linq;
class Program
{
static void Main()
{
string jsonString = "{\"name\":\"John\", \"age\":30, \"city\":\"New York\"}";
try
{
JObject jsonObject = JObject.Parse(jsonString);
Console.WriteLine(jsonObject["name"]);
}
catch (JsonReaderException ex)
{
Console.WriteLine("Invalid JSON string: " + ex.Message);
}
}
}
Output:
John
In this example, we wrap the JObject.Parse()
call in a try-catch block. If the JSON string is invalid, the catch block will handle the exception, and we can print an error message. This is a good practice to ensure that your application does not crash due to unexpected input.
Accessing Nested JSON Objects
JSON often contains nested structures, and accessing these nested objects is straightforward with JObject. You can use the indexer to navigate through the hierarchy of the JSON object. Here’s an example illustrating how to work with nested JSON:
using Newtonsoft.Json.Linq;
class Program
{
static void Main()
{
string jsonString = "{\"person\":{\"name\":\"John\", \"age\":30}, \"city\":\"New York\"}";
JObject jsonObject = JObject.Parse(jsonString);
Console.WriteLine(jsonObject["person"]["name"]);
Console.WriteLine(jsonObject["person"]["age"]);
Console.WriteLine(jsonObject["city"]);
}
}
Output:
John
30
New York
In this example, the JSON string contains a nested object under the “person” key. By using multiple indexers, we can easily access the nested properties. This flexibility allows for greater manipulation of complex JSON structures.
Modifying JSON Objects
Once you have converted a string to a JSON object, you may want to modify its contents. The JObject class provides methods to add, remove, or change properties. Here’s a practical example:
using Newtonsoft.Json.Linq;
class Program
{
static void Main()
{
string jsonString = "{\"name\":\"John\", \"age\":30}";
JObject jsonObject = JObject.Parse(jsonString);
jsonObject["age"] = 31; // Update age
jsonObject["city"] = "New York"; // Add new property
Console.WriteLine(jsonObject.ToString());
}
}
Output:
{
"name": "John",
"age": 31,
"city": "New York"
}
In this case, we first parse the JSON string into a JObject. We then update the age property and add a new property called “city”. Finally, we print the modified JObject, which reflects the changes we made. This capability is particularly useful when you need to update data before sending it back to a server or saving it locally.
Conclusion
Converting a string to a JSON object in C# is a straightforward task, especially with the help of the Newtonsoft.Json library. By utilizing the JObject.Parse()
method, you can easily parse JSON strings, handle exceptions, access nested structures, and modify JSON objects as needed. Understanding these concepts will significantly enhance your ability to work with JSON data in your C# applications, making data interchange more efficient and manageable.
FAQ
- What is JSON?
JSON stands for JavaScript Object Notation, a lightweight format for data interchange that is easy to read and write.
-
Why use JObject.Parse() in C#?
JObject.Parse() allows for easy conversion of JSON strings into JSON objects, enabling manipulation and access to data in structured formats. -
How do I handle invalid JSON strings in C#?
You can use a try-catch block around the JObject.Parse() method to catch exceptions like JsonReaderException, which indicates that the string is not valid JSON. -
Can I modify a JSON object after parsing it?
Yes, once you have parsed a JSON string into a JObject, you can easily modify its properties by using the indexer syntax. -
What library do I need to use for JSON manipulation in C#?
The Newtonsoft.Json library, also known as Json.NET, is the most commonly used library for JSON manipulation in C#.
using the JObject.Parse() function. This comprehensive guide covers parsing, exception handling, accessing nested objects, and modifying JSON data with practical examples. Perfect for developers looking to enhance their skills in JSON manipulation with C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#