How to Check Whether a File Exists in C#
- Understanding the File.Exists() Method
- Checking for File Existence with Full Paths
- Handling Exceptions with File.Exists()
- Conclusion
- FAQ
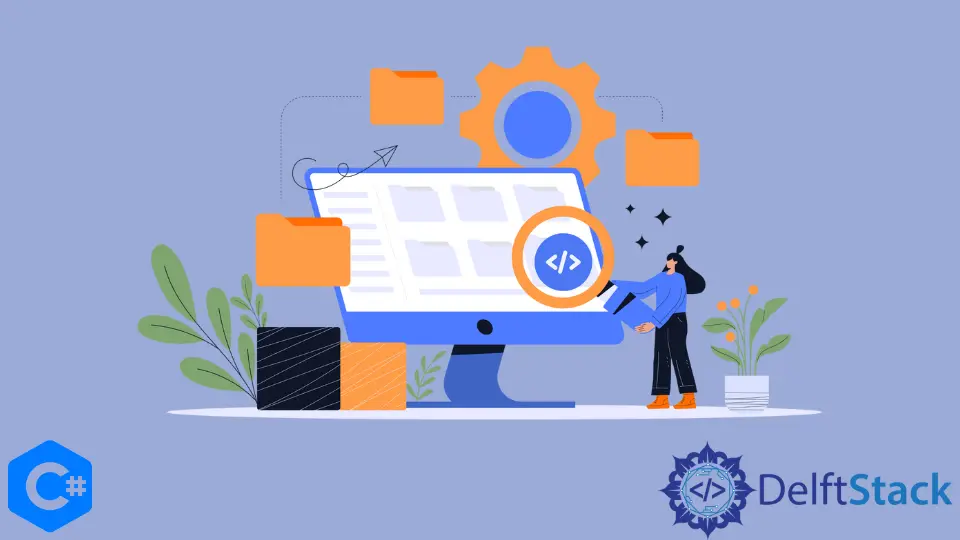
When working with files in C#, it’s crucial to know whether a specific file exists before attempting to read from or write to it. This not only prevents runtime errors but also ensures that your application functions smoothly. The File.Exists()
method is a straightforward way to check for a file’s existence at a given path. By understanding how to utilize this function effectively, you can enhance the robustness of your applications.
In this article, we’ll explore how to check for file existence in C#, including practical code examples and explanations to help you grasp the concept fully.
Understanding the File.Exists() Method
The File.Exists()
method is part of the System.IO
namespace in C#. This method takes a string parameter that specifies the path of the file you want to check. If the file exists, the method returns true
; otherwise, it returns false
. This simple yet powerful method is essential for file handling in C# applications.
Here’s a basic example of how to use the File.Exists()
method:
using System;
using System.IO;
class Program
{
static void Main()
{
string path = "example.txt";
bool fileExists = File.Exists(path);
Console.WriteLine($"Does the file exist? {fileExists}");
}
}
Output:
Does the file exist? False
In this code snippet, we first specify the file path as example.txt
. The File.Exists()
method checks if this file exists and assigns the result to the fileExists
variable. Finally, we print the result to the console. If the file does not exist, it will output False
.
Understanding how to implement this method is vital for file management tasks. It allows developers to handle scenarios where a file might not be present, thus avoiding exceptions that could crash the application.
Checking for File Existence with Full Paths
Using a relative path can sometimes lead to confusion, especially if your application’s working directory changes. Therefore, it’s often better to use a full path when checking for a file’s existence. This ensures that you are looking in the correct location.
Here’s how to do it:
using System;
using System.IO;
class Program
{
static void Main()
{
string fullPath = @"C:\Users\YourUsername\Documents\example.txt";
bool fileExists = File.Exists(fullPath);
Console.WriteLine($"Does the file exist at the specified path? {fileExists}");
}
}
Output:
Does the file exist at the specified path? False
In this example, we specify the full path to example.txt
. The File.Exists()
method checks if the file is located at this exact location. By using a full path, you eliminate any ambiguity that might arise from the current working directory, ensuring that your application behaves predictably.
Handling Exceptions with File.Exists()
While the File.Exists()
method is quite reliable, it’s essential to be aware of potential exceptions that could arise from file handling operations. For instance, if the path provided is invalid or if your application lacks the necessary permissions, you might encounter issues.
To handle these scenarios gracefully, you can wrap your code in a try-catch block. Here’s an example:
using System;
using System.IO;
class Program
{
static void Main()
{
string path = @"C:\InvalidPath\example.txt";
try
{
bool fileExists = File.Exists(path);
Console.WriteLine($"Does the file exist? {fileExists}");
}
catch (UnauthorizedAccessException)
{
Console.WriteLine("You do not have permission to access this file.");
}
catch (DirectoryNotFoundException)
{
Console.WriteLine("The specified directory was not found.");
}
}
}
Output:
The specified directory was not found.
In this code, we attempt to check for the existence of a file in an invalid directory. By using a try-catch block, we can catch specific exceptions like UnauthorizedAccessException
and DirectoryNotFoundException
, allowing us to provide meaningful feedback to users. This approach enhances the robustness of your file handling logic.
Conclusion
Checking whether a file exists in C# is a fundamental skill for developers. The File.Exists()
method provides a simple yet effective way to perform this task. By understanding how to use this method with both relative and full paths, as well as handling exceptions, you can ensure that your applications run smoothly and efficiently. Remember that effective file management is key to building reliable applications, so make sure to incorporate these practices into your coding routine.
FAQ
-
How does the File.Exists() method work?
The File.Exists() method checks if a file exists at the specified path and returns true or false accordingly. -
Can I use relative paths with File.Exists()?
Yes, you can use relative paths, but using full paths is often more reliable to avoid confusion. -
What should I do if the file doesn’t exist?
You can handle the situation by informing the user, creating the file, or taking alternative actions based on your application’s requirements. -
What exceptions can I handle when using File.Exists()?
You can handle exceptions like UnauthorizedAccessException and DirectoryNotFoundException to manage permission issues or invalid paths. -
Is File.Exists() a blocking call?
Yes, the File.Exists() method is a blocking call, meaning it will halt the execution of your program until it completes the check.
using the File.Exists() method. This article provides practical code examples and detailed explanations to help you master file management in your applications. Discover the importance of using full paths and handling exceptions effectively for robust coding practices.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn