How to Check if an Object Is Null in C#
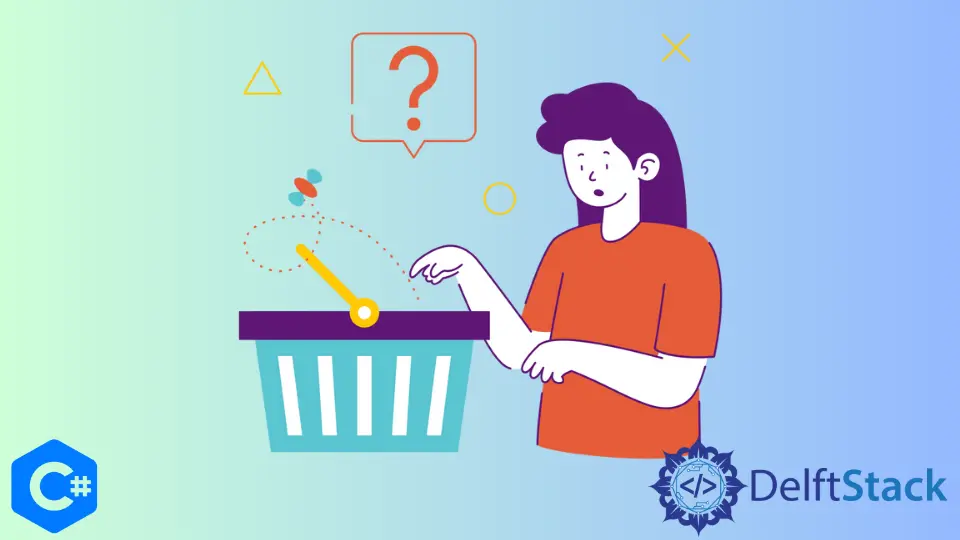
This tutorial will discuss methods to check whether an object is null or not in C#.
Check Null Object With the ==
Operator in C#
The binary operator ==
can check whether the value on the left side of the operator is equal to the value on the right side of the operator in C#. The following code example shows us how to check whether an object is null or not with the ==
operator in C#.
using System;
namespace check_null_object {
class Program {
static void Main(string[] args) {
string check = null;
if (check == null) {
Console.WriteLine("check is null");
} else {
Console.WriteLine("check is not null");
}
}
}
}
Output:
check is null
The above code checks whether the string variable check
is null
or not with the ==
binary operator in C#.
Check Null Object With the is
Keyword in C#
We can also use the is
keyword to check whether an object is null or not in C#. The is
keyword is used as an alternative of the binary operator ==
in C#. The following code example shows us how we can determine whether an object is null or not with the is
keyword in C#.
using System;
namespace check_null_object {
class Program {
static void Main(string[] args) {
string check = null;
if (check is null) {
Console.WriteLine("check is null");
} else {
Console.WriteLine("check is not null");
}
}
}
}
Output:
check is null
The above code checks whether the string variable check
is null
or not with the ==
binary operator in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn