How to Convert Array to List in C#
-
Convert an Array to a List With the
Array.ToList()
Method Inside Linq inC#
-
Convert an Array to a List With the
List.AddRange()
Method inC#
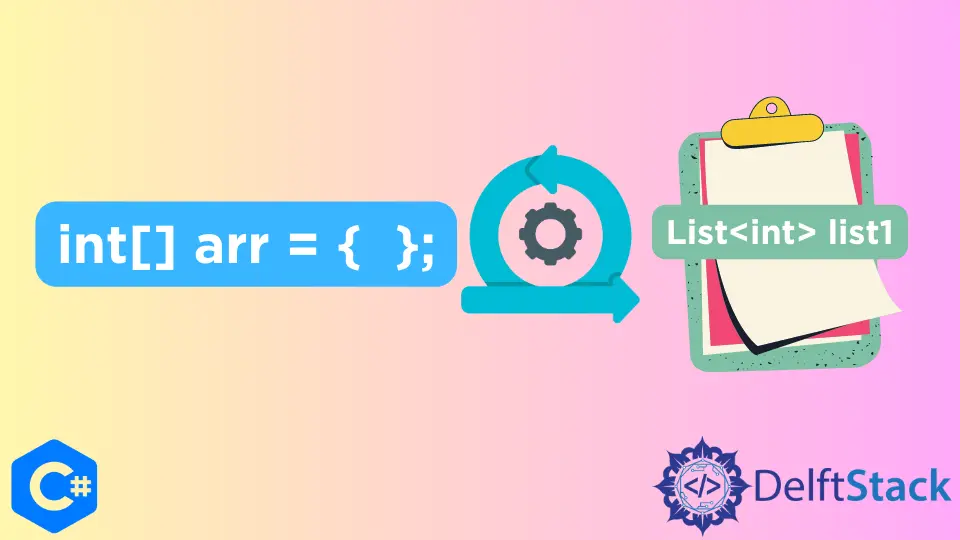
This tutorial will discuss methods to convert an array to a list in C#.
Convert an Array to a List With the Array.ToList()
Method Inside Linq in C#
The Linq or language integrated query is used for fast text manipulation in C#. The Array.ToList()
method inside the Linq can convert an array to a list. The Array.ToList()
method converts the calling array to a list and returns the result in a list data structure. The following code example shows us how to convert an array to a list with the Array.ToList()
method inside the Linq in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace convert_array_to_list {
class Program {
static void Main(string[] args) {
int[] arr = { 10, 12, 13 };
List<int> lst = arr.ToList();
foreach (var element in lst) {
Console.WriteLine(element);
}
}
}
}
Output:
10
12
13
Convert an Array to a List With the List.AddRange()
Method in C#
The List.AddRange()
method is used to insert a range of values inside a list in C#. The List.AddRange()
inserts the element of any data structure inside the calling list. The following code example shows us how to convert an array to a list with the List.AddRange()
function in C#.
using System;
using System.Collections.Generic;
using System.Linq;
namespace convert_array_to_list {
class Program {
static void Main(string[] args) {
int[] arr = { 10, 20, 30 };
List<int> lst = new List<int>();
lst.AddRange(arr);
foreach (var element in arr) {
Console.WriteLine(element);
}
}
}
}
Output:
10
20
30
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Array
- How to Get the Length of an Array in C#
- How to Sort an Array in C#
- How to Sort an Array in Descending Order in C#
- How to Remove Element of an Array in C#
- How to Convert a String to a Byte Array in C#
- How to Adding Values to a C# Array