The value_type in STL Containers in C++
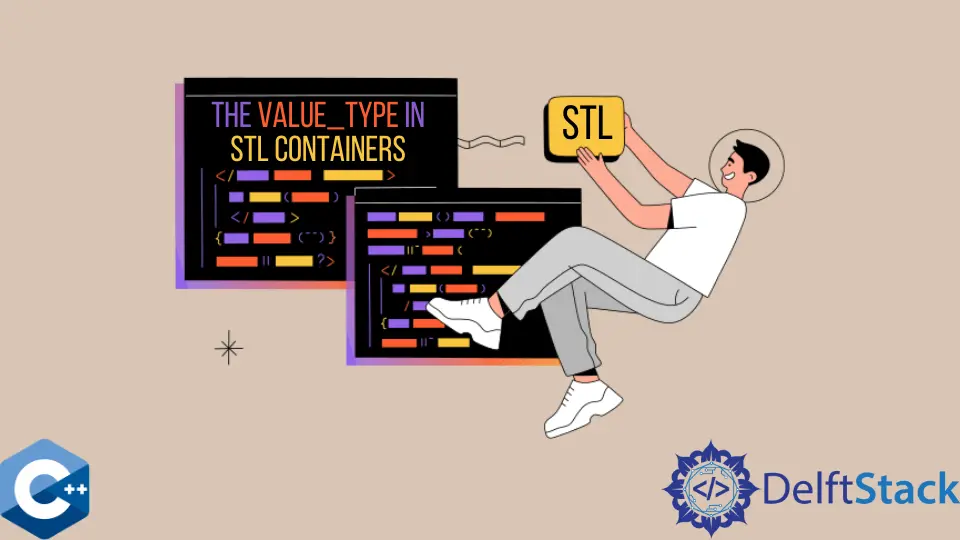
The STL is an abbreviation for the Standard Template Library. It is a set of data structures and algorithms used to implement generic containers and functions for the C++ programming language.
In C++, containers store data in a particular type of object. There are two containers: sequence containers, which hold objects in sequential order, and associative containers, which store objects using an associative key.
the value_type
in STL Containers in C++
We will be discussing STL value types today. The value type is one of the five standard template classes used as the type parameter when declaring a new container class or function template specialization.
STL containers provide us with various ways to store our data, and they do not offer any additional functionality other than what is provided by standard arrays.
They can store any object, but there are some restrictions on the value type of objects stored within them.
The STL container class defines the following types of containers: vector, list, set, map, and multiset.
Each container has its purpose and uses case. Vector is the fastest container, and it is also the most flexible container because it allows random access to its elements.
The list is slower than the vector, but it has better performance when inserting or removing items from the list’s beginning or middle; it also has better performance if you have many items.
The list is used for ordered sequences of elements that can be accessed by their position in the sequence (e.g., 1, 2, 3). The set is used for unordered collections of unique elements (e.g., 1, 2, 3).
The map is used for storing associations between keys and values (e.g., key = “1” and value = “2”).
Use the value_type
in STL Containers in C++
- The first step is to include the header file that defines the value type, e.g.,
vector using namespace std;
. - The second step is declaring a variable of that type and initializing it with a value, e.g.,
int x = 100;
. - The third step is displaying an object of the STL container class and inserting it with a new element or value, e.g.,
vector v;
. - The fourth step is assigning values to the STL container class elements.
#include <iostream>
#include <set>
using namespace std;
int main() {
set<int> numbers = {5, 20, 40, 50};
cout << "values: ";
for (auto &num : numbers) {
cout << num << ", ";
}
return 0;
}
Click here to check the live demo of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook