The switch Statements in C++
-
the
switch-case
Statement in C++ - Rules and Properties in Using Switch Case in C++
-
Difference Between
switch
Statements andif-else
Statements in C++
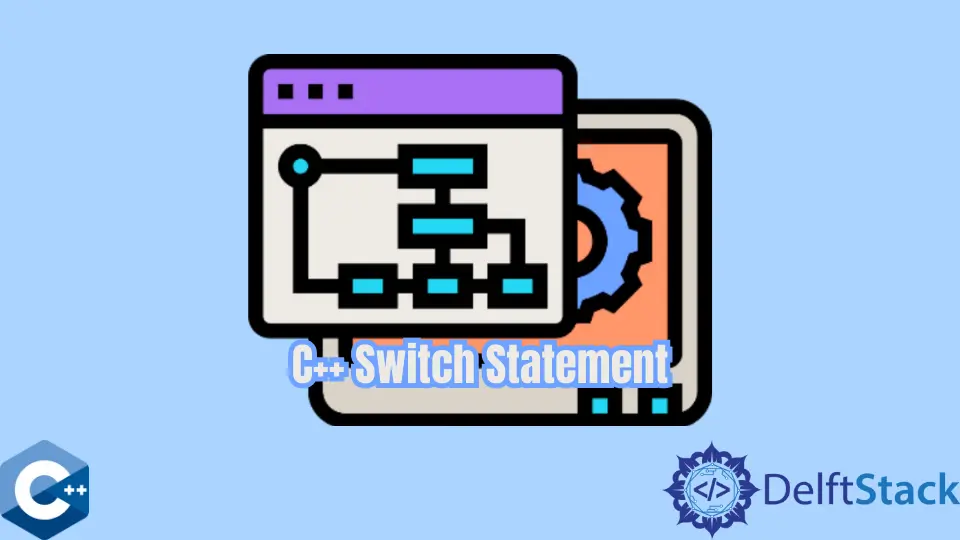
In this article, we will learn about switch
statements in C++ and their special properties.
the switch-case
Statement in C++
The switch-case
evaluates the expression, based on its value, and is tested against a list of constant values present in case
statements to perform different actions based on different cases
.
Like if-else
, switch statements are control flow statements because they can alter a program’s general flow because it allows an expression or a variable to control the flow via a multi-way branching.
Syntax:
switch(variable / expression)
{
case constant-expression 1:
statements;
case constant-expression 2:
statements;
}
Example code:
#include <iostream>
using namespace std;
int main() {
int day = 3;
switch (day) {
case 1:
cout << "Monday\n";
case 2:
cout << "Tuesday\n";
case 3:
cout << "Wednesday\n";
case 4:
cout << "Thursday\n";
case 5:
cout << "Friday\n";
case 6:
cout << "Saturday\n";
case 7:
cout << "Sunday\n";
}
}
Output:
Wednesday
Thursday
Friday
Saturday
Sunday
The value of variable day = 3
is evaluated and matched with the respective case statement that is case 3
, and the statement it contains that is "Wednesday"
is printed, but still we get the remaining strings because once the control goes to case 3
, everything from there till the end of the switch block, all the statements are printed/evaluated.
That’s why the statements from cases
4,5,6,7 are printed.
To avoid this, we have to modify our switch-case
structure.
Syntax - Modified switch-case
:
switch(variable / expression)
{
case constant-expression 1:
statements;
break; (optional)
case constant-expression 2:
statements;
break; (optional)
default: //default statment optional
statements;
break;
}
The break
keyword is utilized to stop the execution of the switch block and come out of it. Once the condition/case is matched, we evaluate the statements, break the flow, and come out of the switch block.
The default
keyword specifies statements executed when no case matches.
Example code:
#include <iostream>
using namespace std;
int main() {
int day = 3;
switch (day) {
case 1:
cout << "Monday\n";
break;
case 2:
cout << "Tuesday\n";
break;
case 3:
cout << "Wednesday\n";
break;
case 4:
cout << "Thursday\n";
break;
case 5:
cout << "Friday\n";
break;
case 6:
cout << "Saturday\n";
break;
case 7:
cout << "Sunday\n";
break;
default:
cout << "Wrong option entereted\n";
break;
}
}
Output:
Wednesday
In this next example, we can also switch with char
. We’ll see an example code that checks whether a character is a vowel or a consonant.
Example code:
#include <iostream>
using namespace std;
int main() {
char x = 'u';
switch (x) {
case 'a':
cout << "Vowel";
break;
case 'e':
cout << "Vowel";
break;
case 'i':
cout << "Vowel";
break;
case 'o':
cout << "Vowel";
break;
case 'u':
cout << "Vowel";
break;
default:
cout << "Consonant";
break;
}
}
Output:
Vowel
Rules and Properties in Using Switch Case in C++
-
The expression used inside the
switch
statement should be aconstant value
. Else it will be considered invalid.Here we can see that constant and variable expressions provided they are assigned with fixed values, can be used.
switch (1 + 2 + 3 * 4 + 5) switch (a + b + c * d)
-
default
andbreak
are optional. Even if theswitch-case
doesn’t have them, it will run without any problem. -
Nesting of
switch
statements is valid means we have can switch statements inside another one. Though it’s not a good programming practice, we can still utilize them.#include <iostream> using namespace std; int main() { int ID = 1234; int password = 000; switch (ID) { case 1234: cout << "checking info......\n"; switch (password) { case 000: cout << "Login successful\n"; break; default: cout << "Password don't match please check it\n"; break; } break; default: printf("incorrect ID"); break; } }
Output:
checking info...... Login successful
-
The expression used inside the
switch
statement must be an integral type meaning it should beint
,char
, orenum
. Else we get a compile error.#include <iostream> using namespace std; int main() { float x = 12.34; switch (x) { case 1.1: cout << "Yes"; break; case 12.34: cout << "NO"; break; } }
Output:
[Error] switch quantity not an integer
-
The
default
block can be inside theswitch
but only executed when no cases are matched.#include <iostream> using namespace std; int main() { int x = 45; switch (x) { default: cout << "It's not 1 or 2"; break; case 1: cout << "It's a 1"; break; case 2: cout << "It's a 2"; break; } }
Output:
It's not 1 or 2
-
The statements written above the case are never executed. Once the
switch
statement is evaluated, the control shifts to either the matching case or thedefault
block if present.#include <iostream> using namespace std; int main() { int x = 45; switch (x) { cout << "Tony is Iron Man\n"; case 1: cout << "It's a 1"; break; case 2: cout << "It's a 2"; break; default: cout << "It's not 1 or 2"; break; } }
Output:
It's not 1 or 2
-
Duplicate case labels are not allowed. If given, we will get a compile error.
#include <iostream> using namespace std; int main() { int x = 45; switch (x) { case 45: cout << "Iron Man"; break; case 40 + 5: cout << "tony stark"; break; default: cout << "It's not 1 or 2"; break; } }
Output:
[Error] duplicate case value
-
The case labels should also be constant. Else, we get a compile error.
#include <iostream> using namespace std; int main() { int x = 2; int arr[] = {100, 200, 300}; switch (x) { case arr[0]: cout << "Iron Man"; break; case arr[1]: cout << "tony stark"; break; case arr[2]: cout << "It's not 1 or 2"; break; } }
Output:
[Error] the value of 'arr' is not usable in a constant expression
-
We can group all the
case
statements performing the same task.#include <iostream> using namespace std; int main() { char x = 'A'; switch (x) { case 'a': case 'e': case 'i': case 'o': case 'u': case 'A': case 'E': case 'I': case 'O': case 'U': cout << "Vowel"; break; default: cout << "consonant"; } }
Difference Between switch
Statements and if-else
Statements in C++
When we have many if-else
statements, the compiler must check all of them until a valid match is found. While in switch-case
statements are used if we only want a certain block of code to run, if a certain condition is met.
The following example checks a character if it is an alphabet or not.
Example code:
char x = '+';
if (x == 'A')
....
else if (x == 'B')...
else if (x == 'C')...
....else cout
<< "Not an alphabet"
Since +
is not an alphabet, it takes much longer to reach the last because it must first examine every previous condition. If implemented using switch-case
, it uses a lookup table or a hash list when the number of cases/conditions is very large.
This means all items get the same access time. So, when we have many cases/conditions
, the switch
is preferred over if-else
as it is faster.