How to Fix the Undefined Reference to Main Error in C++
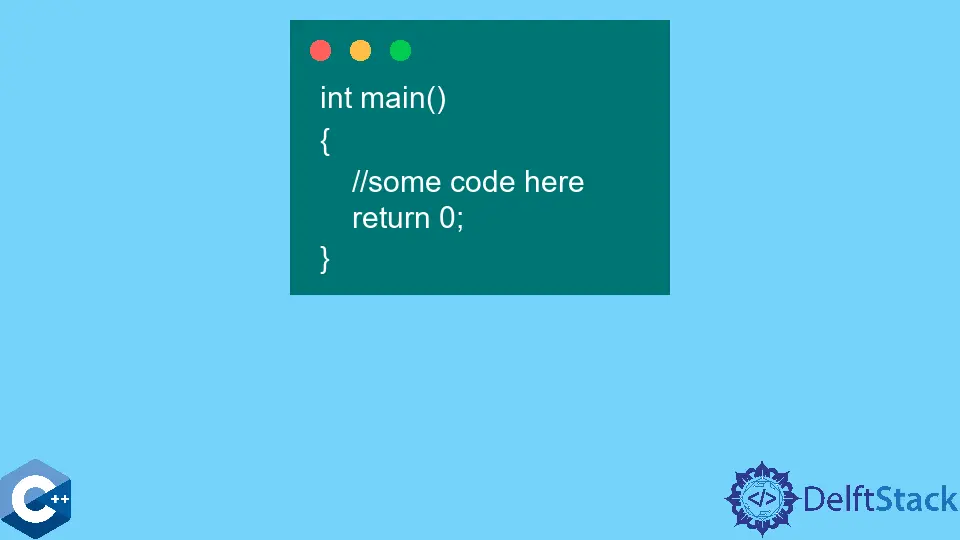
This quick tutorial will briefly discuss one of the most common and equally critical errors in C++ programming, i.e., Undefined Reference to main()
.
First, we will briefly discuss different errors that can occur while coding in C++. Then, we will explain the causes and fixes for the Undefined Reference
error.
Types of Errors in C++
Like any other programming language, the C++ codes may be subjected to errors or bugs for multiple reasons. These errors are broadly classified into the following error categories:
- Syntax Errors
- Run-time Errors
- Logical Error
- Linker Error
Syntax Errors are the errors that occur due to violations in the rules of C++ or any syntaxes. Run-time Errors occur when there is no issue in the program syntactically but is detected at the time of execution and lead to a program crash.
Logical Errors occur when we are not getting our desired results or output, which means there is some mistake in the logic of our program. Linker Errors are errors when the program is compiled successfully and tries to link some other objects with our main
object file; thus, the executable is not generated.
Examples are any wrong prototype of the function defined, any incorrect header file included, etc.
Fix the Undefined Reference to main()
Error in C++
This error is most frequently occurring in C++ and is equally critical, especially for new programmers. This type of linker error can affect the running of the program.
These errors mainly occur when the program is compiled successfully and is at the linking phase trying to link other object files with the main
object.
The Undefined Reference
error occurs when we have not included the main()
function in our code. Usually, with multi-file projects, programmers often forget to include the main()
function.
The main()
function is the driver function and serves as the entry point for every program. Therefore, if the main()
function definition is missing, the program generates the error Undefined Reference to main()
.
The syntax for this function is as follows:
int main() {
// some code here
return 0;
}
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn