Unary Negation Operator in C++
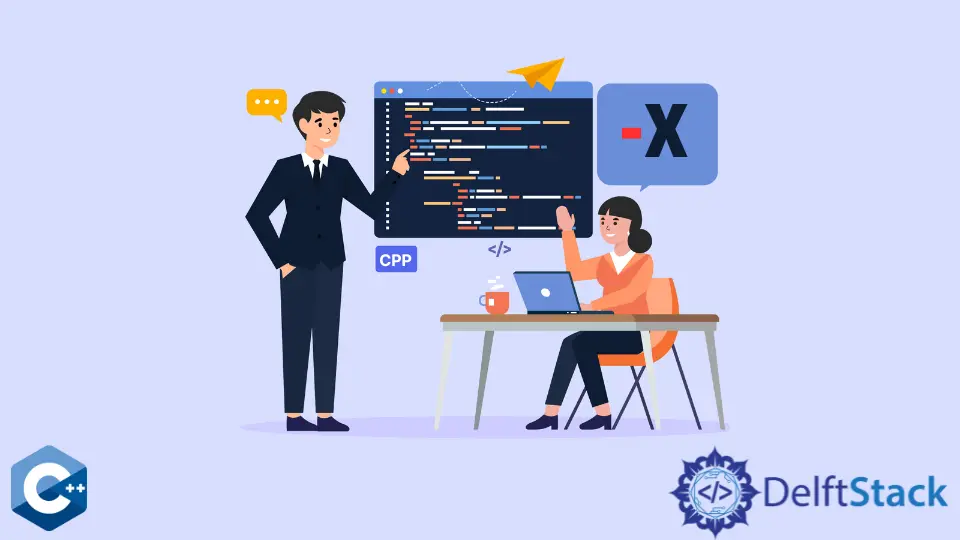
The unary minus operator is used to negate a number, and when it is used before a variable, it negates its value.
The unary minus operator represents the prefix -
sign in C++. You must put the -
sign before a number to negate it; for example, if you want to negate 5, you will type -5, and if you want to negate 4, you will type -4.
You can also use the unary minus operator before variables to negate their values. For example, if we have the variable x
of 2 and put -x
before it, its value will become -2, and if we had y
of 10 and put -y
before it, its value would become -10 as well.
Steps to Overload the Unary Minus Operator in C++
The following steps are required to overload the unary minus operator in C++:
-
Create an operand class inherited from the
basic_operand
class and overrides theunary_operator
function. -
Implement the
unary_operator
function in your operand class to return a negative value for any operation involving this operand. -
Include your operand class in your program by including it as one of the arguments when you create an expression object.
Example:
#include <iostream>
using namespace std;
int main() {
int x = 3;
int y = -x;
cout << "unary postive integer " << x << endl;
cout << "unary minus operator: " << y << endl;
return 0;
}
Click here to check the working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook