The typename Keyword in C++
- Qualified and Unqualified Names in C++
- Dependent and Non-Dependent Names in C++
-
Using the
typename
Keyword in C++
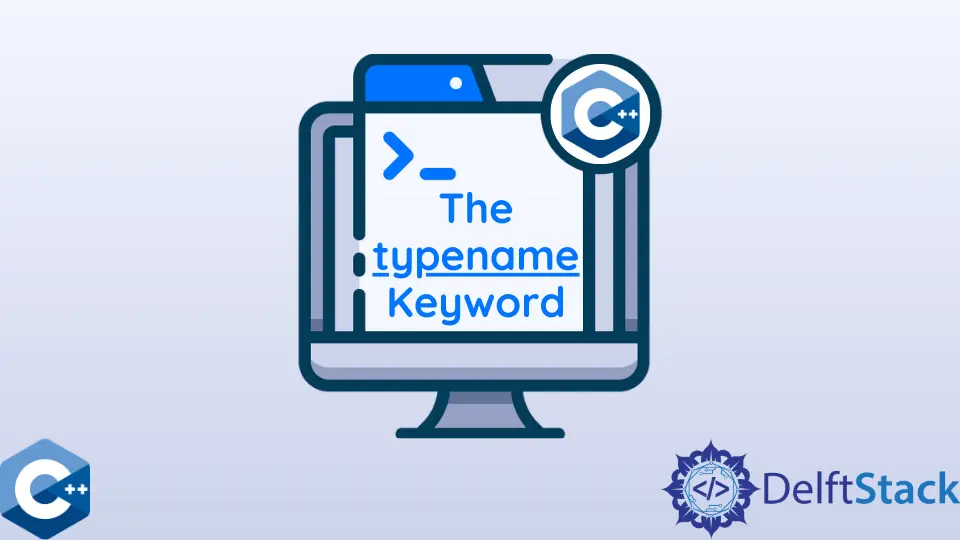
This article will discuss the keyword typename
in C++.
To understand the significance of the keyword typename
, we need to understand the key concepts of qualified
and dependent
names.
Qualified and Unqualified Names in C++
A qualified name specifies a scope. Let’s see an example to understand it better.
#include <bits/stdc++.h>
int main() { std::cout << "Hello world!" << std::endl; }
The references to cout
and endl
are qualified names here. But if we bring both of them into the scope with a using
declaration, i.e., using namespace std
and just used cout
by itself, they would become unqualified
names because they lack the std::
.
Dependent and Non-Dependent Names in C++
Dependent names are the names that depend upon a template
parameter.
Let’s look at a sample code to understand it better.
template <class T>
class MyClass {
int i;
vector<int> vi;
vector<int>::iterator vitr;
T t;
vector<T> vt;
vector<T>::iterator viter;
}
The first three declarations are known as non-dependent names because their type is known at the time of template declaration.
Whereas if we look at the second set of declarations, T
, vector<T>
, and vector<T>::iterator
are dependent names because their type is not known until the point of instantiation as they depend on the template parameter T
.
Using the typename
Keyword in C++
As a general rule, the typename
keyword is mandatory to use before a qualified or dependent name that refers to a type.
So, the keyword typename
is introduced to specify that the identifier that follows is a type rather than a static member variable.
class A {
public:
typedef int myPtr;
};
template <class T>
class myClass {
public:
typename T::SubType* myPtr;
}
In the above code, the typename
keyword tells the compiler that SubType
is a type of class T
, meaning that pointer myptr
is a type of T::SubType
.
Without the typename
keyword, SubType
will be considered a static member, and the compiler will evaluate it as multiplication of SubType
of type T
with the pointer myptr
.
T::SubType * myptr //compiler will think it's multiplication
If we don’t use the keyword typename
in the above code, it will lead to a compile-time error because the compiler doesn’t know whether T::SubType
will refer to a type name or a static member of T
.