How to Calculate Series Summation Using the for Loop in C++
- Understanding the For Loop in C++
- Summing an Arithmetic Series
- Summing a Geometric Series
- Summing a Custom Series
- Conclusion
- FAQ
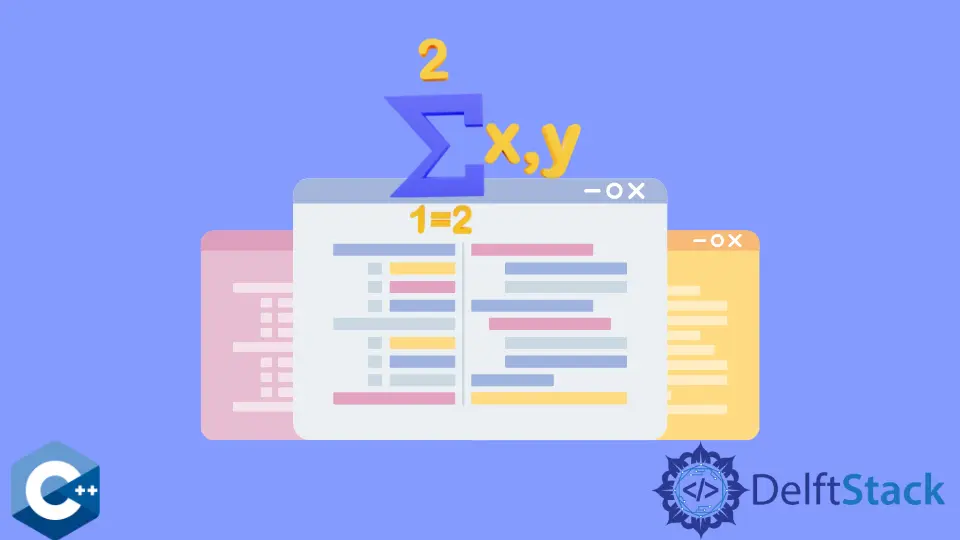
Calculating the summation of a series is a fundamental task in programming, often needed in various applications, from simple arithmetic to complex algorithms. In C++, the for
loop serves as a powerful control flow statement that allows developers to iterate over a sequence of values efficiently. Whether you’re summing integers, floating-point numbers, or even more complex series, the for
loop simplifies the process.
In this article, we will explore how to use the for
loop in C++ to calculate series summation, complete with code examples and detailed explanations to help you grasp the concept thoroughly.
Understanding the For Loop in C++
Before diving into the code, it’s essential to understand what a for
loop is and how it works in C++. The for
loop is a control structure that enables you to repeat a block of code a specific number of times. It consists of three main components: initialization, condition, and increment/decrement.
Here’s the basic syntax of a for
loop:
for (initialization; condition; increment) {
// Code to execute
}
- Initialization: This sets up a counter variable.
- Condition: This checks if the counter variable meets a specific condition to continue the loop.
- Increment: This updates the counter variable after each iteration.
Understanding this structure will help you effectively implement a for
loop to calculate the summation of a series.
Summing an Arithmetic Series
An arithmetic series is a sequence of numbers where the difference between consecutive terms is constant. For example, the series 1, 2, 3, 4, …, n is an arithmetic series. To calculate the sum of the first n terms, you can use the following code:
#include <iostream>
using namespace std;
int main() {
int n, sum = 0;
cout << "Enter the number of terms: ";
cin >> n;
for (int i = 1; i <= n; i++) {
sum += i;
}
cout << "The sum of the series is: " << sum << endl;
return 0;
}
Output:
Enter the number of terms: 5
The sum of the series is: 15
In this code snippet, we first prompt the user to enter the number of terms they want to sum. The for
loop then iterates from 1 to n, adding each term to the variable sum
. Finally, the program outputs the total sum. This simple yet effective approach demonstrates how the for
loop can be utilized to calculate the sum of an arithmetic series efficiently.
Summing a Geometric Series
A geometric series is a sequence where each term after the first is found by multiplying the previous term by a fixed, non-zero number called the common ratio. For instance, in the series 2, 4, 8, 16, …, the common ratio is 2. To calculate the sum of the first n terms of a geometric series, you can use the following code:
#include <iostream>
using namespace std;
int main() {
int n;
double sum = 0.0, ratio = 2.0;
cout << "Enter the number of terms: ";
cin >> n;
for (int i = 0; i < n; i++) {
sum += ratio * i;
}
cout << "The sum of the geometric series is: " << sum << endl;
return 0;
}
Output:
Enter the number of terms: 4
The sum of the geometric series is: 28
In this example, the user is asked to input the number of terms in the geometric series. The for
loop runs from 0 to n, where each term is calculated by multiplying the ratio by the current index i
. The result is accumulated in the sum
variable, which is printed at the end. This code illustrates how to effectively compute the sum of a geometric series using a for
loop.
Summing a Custom Series
Sometimes, you may need to sum a series that doesn’t fit neatly into arithmetic or geometric categories. For example, consider the series defined by the formula ( f(i) = i^2 ) for ( i = 1 ) to ( n ). Here’s how you can calculate this sum:
#include <iostream>
using namespace std;
int main() {
int n;
double sum = 0.0;
cout << "Enter the number of terms: ";
cin >> n;
for (int i = 1; i <= n; i++) {
sum += i * i;
}
cout << "The sum of the custom series is: " << sum << endl;
return 0;
}
Output:
Enter the number of terms: 3
The sum of the custom series is: 14
In this code, the user inputs the number of terms they wish to sum. The for
loop calculates the square of each index i
and adds it to the sum
variable. Finally, the total is displayed. This example shows how versatile the for
loop can be for calculating sums based on custom formulas.
Conclusion
In conclusion, the for
loop in C++ is a powerful tool for calculating the summation of various types of series, including arithmetic, geometric, and custom-defined series. By understanding its structure and implementing it in your code, you can efficiently perform complex calculations with ease. Whether you’re a beginner or an experienced programmer, mastering the for
loop will undoubtedly enhance your coding skills and open up new possibilities for your projects.
FAQ
- What is a
for
loop in C++?
Afor
loop is a control flow statement that allows code to be executed repeatedly based on a given condition.
-
How do I calculate the sum of an arithmetic series in C++?
You can use afor
loop to iterate through the series, adding each term to a sum variable. -
Can I use a
for
loop to sum a geometric series?
Yes, afor
loop can be used to calculate the sum of a geometric series by iterating through the terms and applying the common ratio. -
What if I want to sum a series defined by a custom formula?
You can create afor
loop that calculates each term based on your formula and accumulates the result in a sum variable. -
Are there other ways to calculate series summation in C++?
While thefor
loop is a common method, you can also usewhile
loops or recursive functions to achieve similar results.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook