How to Specify 64-Bit Integer in C++
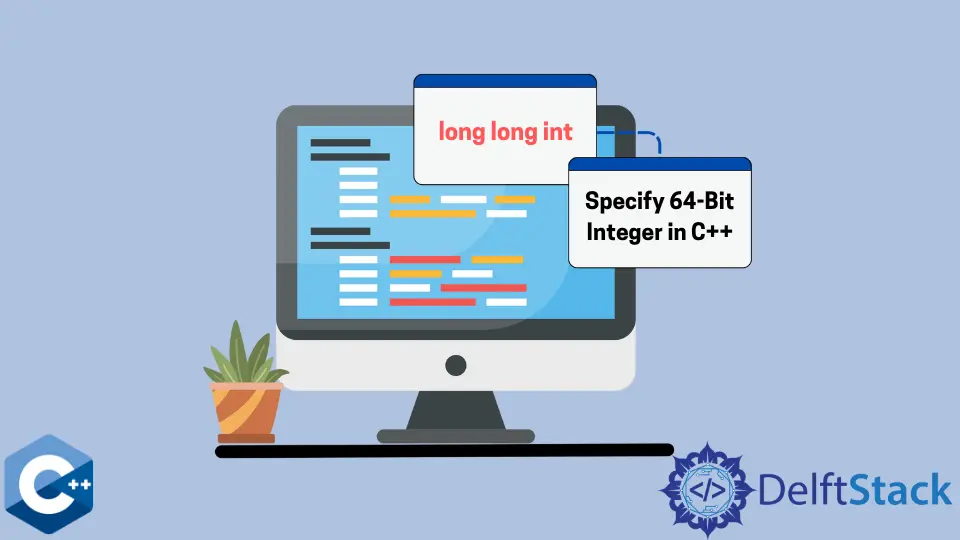
In today’s tutorial, we will discuss and learn about how we can specify 64-bit integers in C++. Further, we will compare the older methods when there were problems with using 64-bit integers.
Specify the 64-Bit Integer in C++
We have multiple choices to handle integers in C++. Starting from the int
data type to long int
and long long int
that has 64-bits, you can check the size using the following code:
#include <iostream>
using namespace std;
int main() {
long long int v;
cout << sizeof(v) << '\n';
return 0;
}
The output is 8
bytes or 64
bits. Let’s compare the maximum value that can be handled by long long int
using the following code:
#include <iostream>
using namespace std;
int main() {
long long int v = 9223372036854775807;
cout << sizeof(v) << '\n';
cout << v << '\n';
return 0;
}
The output is 9223372036854775807
, which is 8
bytes. However, we will get an error saying, integer constant is so large that it is unsigned
if you increase the value of v
.
It implies that 9223372036854775807
is the maximum range of positive values handled correctly. If you convert this number into hexadecimal, the value is 7FFFFFFFFFFFFFFF
.
It means we have all bits 1
except the sign bit. Similarly, the maximum range for negative integers is -9223372036854775808
.
Therefore, there seems to be no issue with a 64-bit integer; however, in the past, many processors were not capable of handling 64-bit integers. Thus, the same codes were giving the wrong output.
For example, there were error messages when we tried to store the following information. See code and related error messages:
long long int h2 = 0x4444000044444;
The error message was:
warning: overflow in implicit constant conversion
warning: integer constant is too large for its type
Besides the long long int
, there was another type available in stdint.h
, which is int64_t
. Again, if you check the size, you will get 8
bytes or 64
bits. However, the result is the same.
The discussion concludes that we had issues in the past while using 64-bit integers in C++. It was compiler and architecture dependent; however, this problem doesn’t exist now in the newer compiler versions.
One can safely handle 64-bit integers in long long int
or int64_t
. These types not only store value, but you can also perform mathematical operations, as far as a result is within 64 bits.