How to Remove Element From Vector in C++
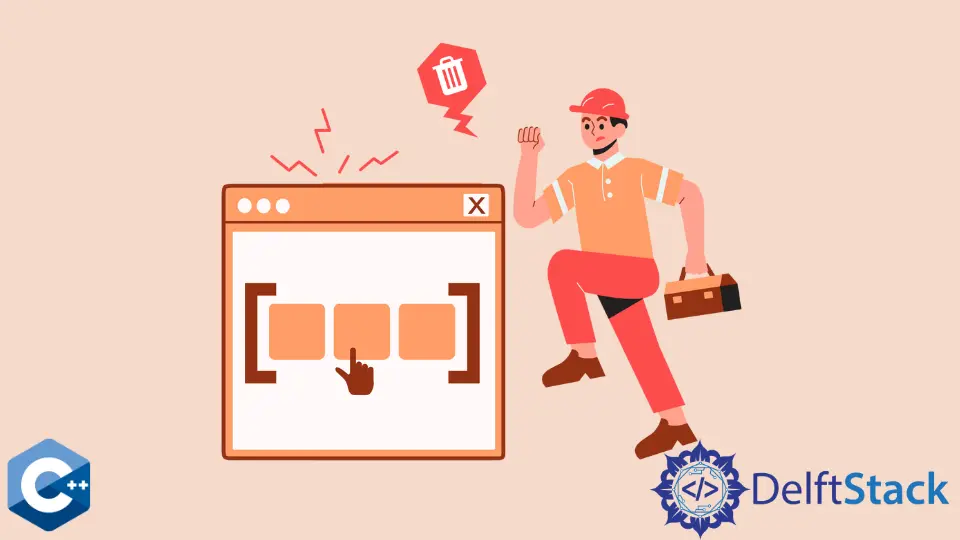
In C++, vectors are dynamic arrays that can grow or shrink in size, making them a popular choice for managing collections of data. However, there may come a time when you need to remove an element from a vector. Whether you’re trying to eliminate duplicates, filter out unwanted values, or simply manage your data more effectively, understanding how to remove elements from a vector is essential for any C++ developer.
In this article, we will explore various methods to remove elements from vectors in C++, complete with clear code examples and explanations. Let’s dive in!
Using the erase() Method
The erase()
method is one of the most straightforward ways to remove an element from a vector. This method allows you to specify the position of the element you want to remove. Here’s how it works:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.erase(numbers.begin() + 2); // Removes the element at index 2 (which is 3)
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Output:
1 2 4 5
The erase()
method takes an iterator as an argument, which points to the element you want to remove. In this example, we used numbers.begin() + 2
to get the iterator for the element at index 2. After calling erase()
, the element 3
is removed, and the vector now contains 1, 2, 4, 5
. This method is efficient for single removals but can be less efficient if you need to remove multiple elements, as it may require shifting elements in memory.
Using the remove() Function
Another effective way to remove elements from a vector is by using the remove()
algorithm from the <algorithm>
header, combined with the erase()
method. This approach is particularly useful when you want to remove all occurrences of a specific value. Here’s how to implement it:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 2, 4, 5};
numbers.erase(std::remove(numbers.begin(), numbers.end(), 2), numbers.end());
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Output:
1 3 4 5
In this example, we first call std::remove()
to shift all occurrences of 2
to the end of the vector and return an iterator pointing to the new logical end of the vector. Then, we use the erase()
method to remove the unwanted elements from the actual vector. This combination is efficient and elegant, allowing you to remove multiple elements in one go without the need for explicit loops.
Removing Elements by Value
If you need to remove elements based on a specific condition or value, you can use a loop to iterate through the vector and remove elements that match your criteria. This method gives you more control over the removal process. Here’s an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 3, 6};
for (auto it = numbers.begin(); it != numbers.end(); ) {
if (*it == 3) {
it = numbers.erase(it); // Erase returns the next iterator
} else {
++it; // Only increment if no erase
}
}
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Output:
1 2 4 5 6
In this code, we use an iterator to traverse the vector. When we find an element that equals 3
, we call erase()
to remove it and update the iterator to the next element. If the current element is not 3
, we simply increment the iterator. This method is flexible and allows for complex conditions, making it a powerful tool for managing vector contents.
Conclusion
Removing elements from a vector in C++ is a fundamental skill that enhances your ability to manage dynamic data collections. Whether you choose to use the erase()
method, the remove()
function, or a custom loop, each method has its strengths and can be applied in various scenarios. By mastering these techniques, you’ll be better equipped to handle data manipulation tasks in your C++ projects. Happy coding!
FAQ
-
What is the difference between erase() and remove() in C++?
erase() directly removes elements from a vector, while remove() shifts elements and marks them for removal, requiring an additional call to erase() to actually remove them from the vector. -
Can I remove elements from a vector while iterating over it?
Yes, but you need to be careful. Use an iterator and update it appropriately after calling erase() to avoid invalidating it. -
Is it possible to remove all elements from a vector?
Yes, you can clear all elements using the clear() method, which removes all elements and reduces the vector size to zero. -
How do I remove duplicates from a vector?
You can use the remove() function followed by erase() to remove duplicates. Alternatively, you can use a set to filter out duplicates before inserting them back into the vector. -
Are there performance considerations when removing elements from a vector?
Yes, removing elements can be costly in terms of performance, especially if you are removing elements from the beginning or middle of the vector, as it requires shifting elements.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook