Concept of Read/Write Locks in C++
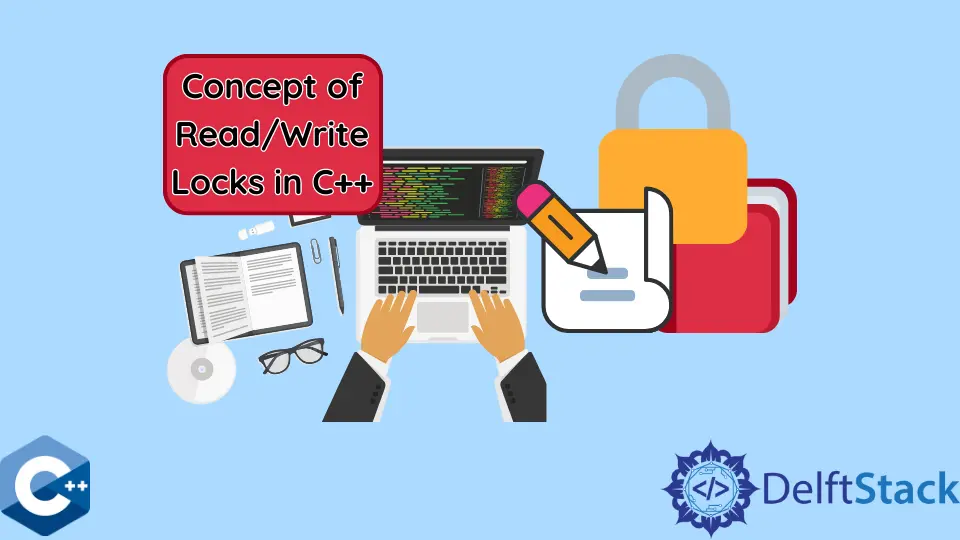
A reader/writer lock is a synchronization mechanism that allows threads to acquire the lock for reading or writing so that one thread does not block another from accessing the resource while it is being modified.
They are often used to implement read-only shared data structures, such as a global cache or lookup table. The reader/writer lock can be implemented using either condition variables or mutexes.
Purpose and Uses of Reader/Writer Locks
Reader locks are used when the reader cannot change the data. The writer lock is used when a writer wants exclusive access to modify data.
Reader locks are also called “read-only” locks and are often found in multi-user systems. They allow one or more readers to access the data without affecting it, while a writer can only change the data if they have exclusive access to it.
On the other hand, writer locks are used when only one person needs to be able to write changes in a file at a time. This prevents simultaneous writing and ensures no conflict between updates made by different people at different times.
Steps to Implement Reader/Writer Locks in C++
In C++, reader/writer locks can be implemented in a few steps.
- First, the lock must be initialized with a
shared_ptr
to the resource protected by the lock. - Second, a function must be created to acquire and release the lock when needed.
- Third,
acquire
andrelease
functions must be called at appropriate times. - Fourth, a function needs to be created that will check if any other threads are waiting for the same resource already holding it and, if so, wait until they have released it before acquiring it again.
- Fifth, call this function from the
acquire
andrelease
functions from step two.
Example:
#include <iostream>
#include <mutex>
#include <shared_mutex>
#include <thread>
using namespace std;
class demo {
public:
void sam() {
unique_lock lock(mutex_);
++b;
}
unsigned int get() {
shared_lock lock(mutex_);
return b;
}
private:
mutable shared_mutex mutex_;
unsigned int b = 0;
};
int main() {
demo tbh;
auto sam_and_roi = [&tbh]() {
for (int x = 0; x < 6; x++) {
tbh.sam();
cout << this_thread::get_id() << tbh.get() << '\n';
}
};
thread start(sam_and_roi);
start.join();
}
Click here to check the working of the example as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook