How to Read File Char by Char in C++
-
Use
ifstream
andget
Method to Read File Char by Char -
Use the
getc
Function to Read File Char by Char -
Use the
fgetc
Function to Read File Char by Char
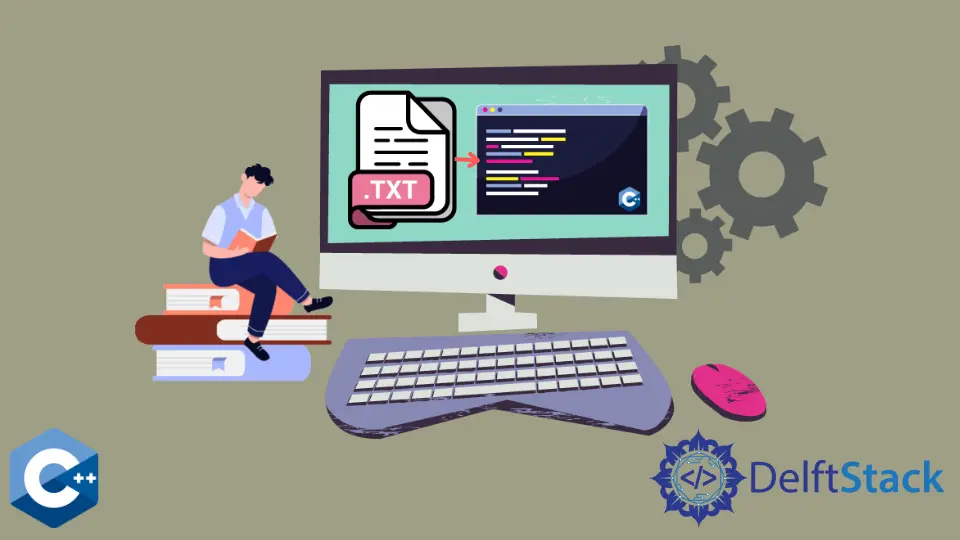
This article will explain several methods of how to read a text file char
by char
in C++.
Use ifstream
and get
Method to Read File Char by Char
The most common way to deal with file I/O the C++ way is to use std::ifstream
. At first, an ifstream
object is initialized with the argument of the filename that needs to be opened. Notice that, if
statement verifies if the opening of a file succeeded. Next, we use the built-in get
function to retrieve the text inside the file char by char and push_back
them in the vector
container. Finally, we output vector elements to the console for demonstration purposes.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
char byte = 0;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file.get(byte)) {
bytes.push_back(byte);
}
for (const auto &i : bytes) {
cout << i << "-";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
Use the getc
Function to Read File Char by Char
Another method to read file char by char is to use getc
function, which takes FILE*
stream as an argument and reads the next character if available. Next, the input file stream should be iterated until the last char
is reached, and that’s implemented with the feof
function, checking if the end of the file has been reached. Note that it’s always recommended to close the open file streams once they are no longer needed.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) {
return EXIT_FAILURE;
}
unsigned char character = 0;
while (!feof(input_file)) {
character = getc(input_file);
cout << character << "-";
}
cout << endl;
fclose(input_file);
return EXIT_SUCCESS;
}
Use the fgetc
Function to Read File Char by Char
fgetc
is an alternative method to the previous function, which implements exactly the same functionality as getc
. In this case, fgetc
return value is used in the if
statement since it returns the EOF
if the end of the file stream is reached. As recommended, before the program exit, we close the stream with a fclose
call.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) {
return EXIT_FAILURE;
}
int c;
while ((c = fgetc(input_file)) != EOF) {
putchar(c);
cout << "-";
}
cout << endl;
fclose(input_file);
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook