Pre-Increment VS Post-Increment Operators in C++
- Difference Between Pre-Increment and Post-Increment Operations in C++
- Don’t Use Post-Increment and Pre-Increment Operations With Other Arithmetic Operations of the Same Variable
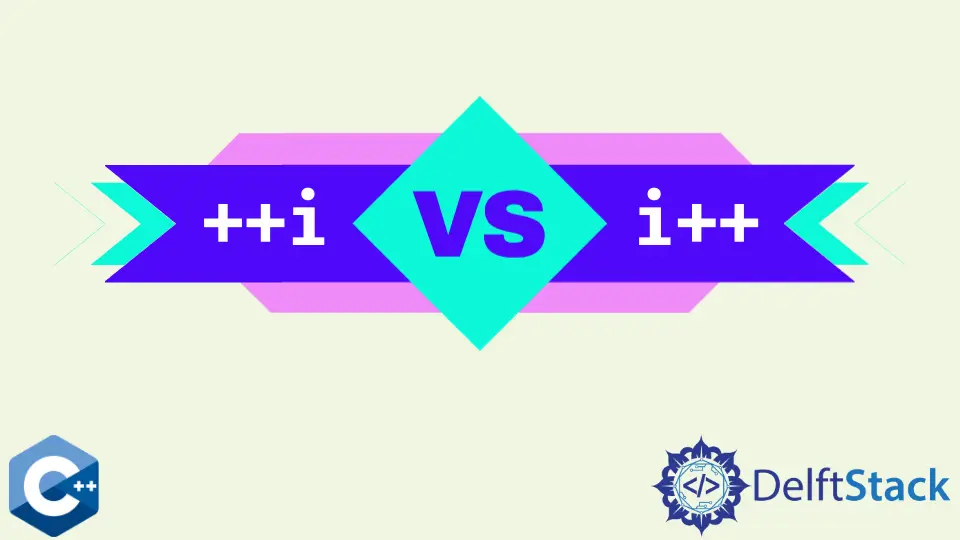
This article will introduce the differences between pre-increment and post-increment in C++.
Difference Between Pre-Increment and Post-Increment Operations in C++
Both operations are based on the unary increment operator - ++
, which has slightly different behavior when used in expressions where the variable’s value is accessed. Generally, a variable can be combined with increment or decrement operator after or before that yields the value bigger/smaller by 1. Note though, pre-increment operation modifies the given variable at first and then accesses it. On the other hand, the post-increment operator accesses the original value and then increments it. Thus, when used in an expression where the value is accessed or stored, the operator can have different effects on the program. E.g., the following example code initializes the two integers to 0
values and then iterates five times while outputting the incremented values for each with different operators. Note that post-increment starts printing with 0
and ends with 4
, while pre-increment starts with 1
and finishes with 5
. Nevertheless, the final value stored in both integers is the same - 5
.
#include <iostream>
using std::cout;
using std::endl;
int main() {
int i = 0, j = 0;
while ((i < 5) && (j < 5)) {
cout << "i: " << i++ << " ";
cout << "j: " << ++j << endl;
}
cout << "End of the loop, both have equal values:" << endl;
cout << "i: " << i << " "
<< "j: " << j << endl;
exit(EXIT_SUCCESS);
}
Output:
i: 0 j: 1
i: 1 j: 2
i: 2 j: 3
i: 3 j: 4
i: 4 j: 5
End of the loop, both have equal values:
i: 5 j: 5
Alternatively, using the different notations within the for
loop’s conditional expression behaves the same. Both loops get executed 5
iterations. Although, it’s usually accepted style to use pre-increment, which can be a more performance efficient variant when the code is not optimized.
#include <iostream>
using std::cout;
using std::endl;
int main() {
int i = 0, j = 0;
while ((i < 5) && (j < 5)) {
cout << "i: " << i++ << " ";
cout << "j: " << ++j << endl;
}
cout << "End of the loop, both have equal values:" << endl;
cout << "i: " << i << " "
<< "j: " << j << endl;
cout << endl;
for (int k = 0; k < 5; ++k) {
cout << k << " ";
}
cout << endl;
for (int k = 0; k < 5; k++) {
cout << k << " ";
}
cout << endl;
exit(EXIT_SUCCESS);
}
Output:
i: 0 j: 1
i: 1 j: 2
i: 2 j: 3
i: 3 j: 4
i: 4 j: 5
End of the loop, both have equal values:
i: 5 j: 5
0 1 2 3 4
0 1 2 3 4
Don’t Use Post-Increment and Pre-Increment Operations With Other Arithmetic Operations of the Same Variable
Note that post-increment and pre-increment operators can make arithmetic calculations entirely erroneous when used with the same variable. For example, combining the post-increment with the multiplication of the same variable makes unintuitive code to read and even error-prone if not treated with enough diligence.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<int> vec1 = {10, 22, 13, 41, 51, 15};
for (int &k : vec1) {
cout << k++ * k << ", ";
}
exit(EXIT_SUCCESS);
}
Output:
110, 506, 182, 1722, 2652, 240,
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook