How to Print All Permutations of the String in C++
-
Use
std::next_permutation
to Print All Permutations of the String in C++ -
Use
std::prev_permutation
to Print All Permutations of the String in C++
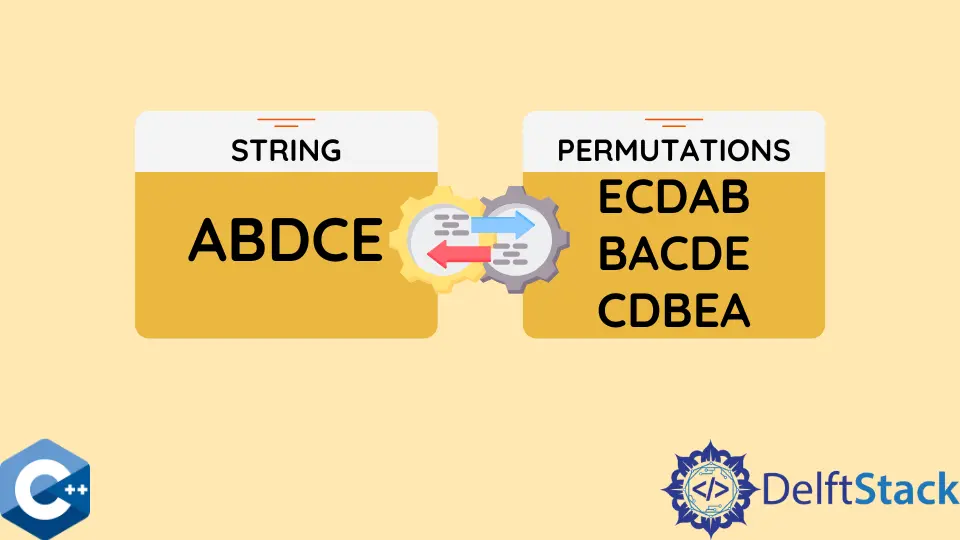
This article will introduce how to print all permutations of the given string in C++.
Use std::next_permutation
to Print All Permutations of the String in C++
The std:next_permutation
algorithm modifies the given range so that the permutation of the elements is ordered lexicographically in ascending order, and a true boolean value is returned if such permutation exists. The function can operate on the std::string
object to generate its permutations if the string characters are sorted in descending order. We can utilize std::sort
algorithm with std::greater
function object to sort the string and then call next_permutation
until it returns false. The latter can be implemented using the do...while
loop that takes the next_permutation
statement as the condition expression and prints the string to the cout
stream each cycle.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::next_permutation(str.begin(), str.end()));
}
int main() {
string input;
cout << "Enter string to print permutations: ";
cin >> input;
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
Output:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
Use std::prev_permutation
to Print All Permutations of the String in C++
Alternatively, we can utilize another algorithm from STL called - std::prev_permutation
that generates the new permutation of the given range with the same lexicographical order but stores the previous permutation when the sequence is provided. The final solution still remains similar to the previous example, except that the prev_permutation
function is called in the while
loop condition.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::prev_permutation(str.begin(), str.end()));
}
int main() {
string input;
cout << "Enter string to print permutations: ";
cin >> input;
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
Output:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
Additionally, one can ensure more robust code by implementing the user string validation function that will print the input prompt until the user supplies the valid string. Note that all listed solutions will parse only the single string argument from the command line input, and multi-word strings will not be read.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
using std::string;
void PrintStringPermutations(string &str) {
std::sort(str.begin(), str.end(), std::greater<>());
do {
cout << str << endl;
} while (std::prev_permutation(str.begin(), str.end()));
}
template <typename T>
T &validateInput(T &val) {
while (true) {
cout << "Enter string to print permutations: ";
if (cin >> val) {
break;
} else {
if (cin.eof()) exit(EXIT_SUCCESS);
cout << "Enter string to print permutations\n";
cin.clear();
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
}
}
return val;
}
int main() {
string input;
validateInput(input);
PrintStringPermutations(input);
return EXIT_SUCCESS;
}
Output:
Enter string to print permutations: nel
nle
nel
lne
len
enl
eln
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++