Function Overloading VS Overriding in C++
- Use Function Overloading to Define Multiple Functions with Different Parameter Lists in C++
- Use Function Overriding to Redefine Inherited Members in Derived Class in C++
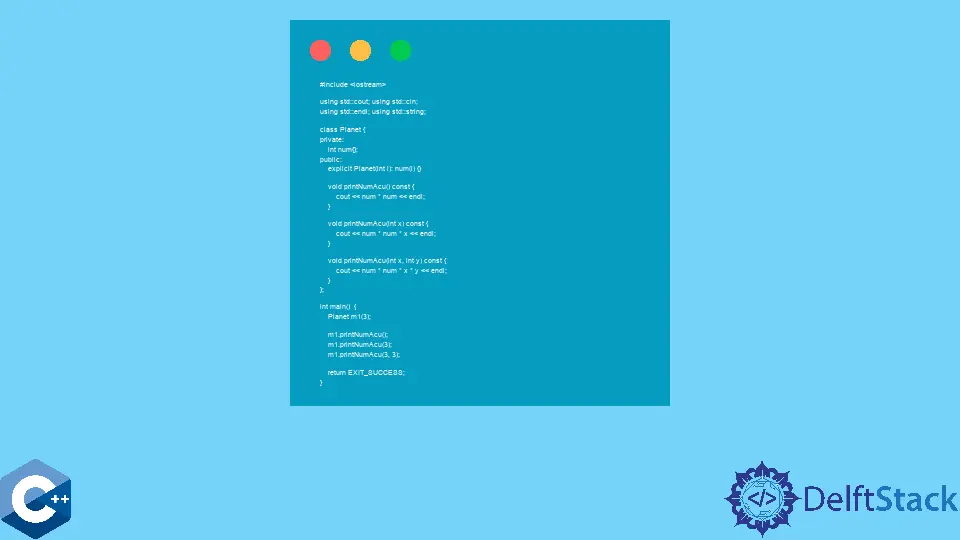
This article will introduce the differences between function overloading VS overriding in C++.
Use Function Overloading to Define Multiple Functions with Different Parameter Lists in C++
Function overloading is the feature of the C++ language to be able to have multiple functions with the same name, which have different parameters and are located in one scope. Generally, overloaded functions conduct very similar operations, and it’s just intuitive to define a single function name for them and expose the interface with multiple parameter sets. In this case, we defined a class named Planet
with three functions named printNumAcu
. All these functions have different parameters, and they are differentiated at the compile time. Note that overloaded functions must have different parameter types or a different number of parameters.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
class Planet {
private:
int num{};
public:
explicit Planet(int i) : num(i) {}
void printNumAcu() const { cout << num * num << endl; }
void printNumAcu(int x) const { cout << num * num * x << endl; }
void printNumAcu(int x, int y) const { cout << num * num * x * y << endl; }
};
int main() {
Planet m1(3);
m1.printNumAcu();
m1.printNumAcu(3);
m1.printNumAcu(3, 3);
return EXIT_SUCCESS;
}
Output:
9
27
81
Use Function Overriding to Redefine Inherited Members in Derived Class in C++
Function overriding is the feature associated with the derived classes and their inheritance. Usually, derived classes inherit all base class members, and some get redefined in the derived class to implement a custom routine. Note, though, the base class needs to specify the member functions that are supposed to be overridden. These member functions are known as virtual functions and include the keyword virtual
in a definition too. There is also a concept of a pure virtual function, which is a type of virtual function that does not need to be defined. Classes that include or inherit (without overriding) a pure virtual function are called abstract base classes, which usually should not be used to create objects, but rather derive other classes.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
class Planet {
protected:
int num{};
public:
explicit Planet(int i) : num(i) {}
virtual void printName() = 0;
};
class Mars : public Planet {
public:
explicit Mars(int i) : Planet(i) {}
void printName() override { cout << "Name's Mars " << num << endl; }
};
class Earth : public Mars {
public:
explicit Earth(int i) : Mars(i) {}
void printName() override { cout << "Name's Earth " << num << endl; }
};
int main() {
Earth e1(3);
e1.printName();
Mars m1 = e1;
m1.printName();
return EXIT_SUCCESS;
}
Output:
Name's Earth 3
Name's Mars 3
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook