Overloaded Constructor in C++
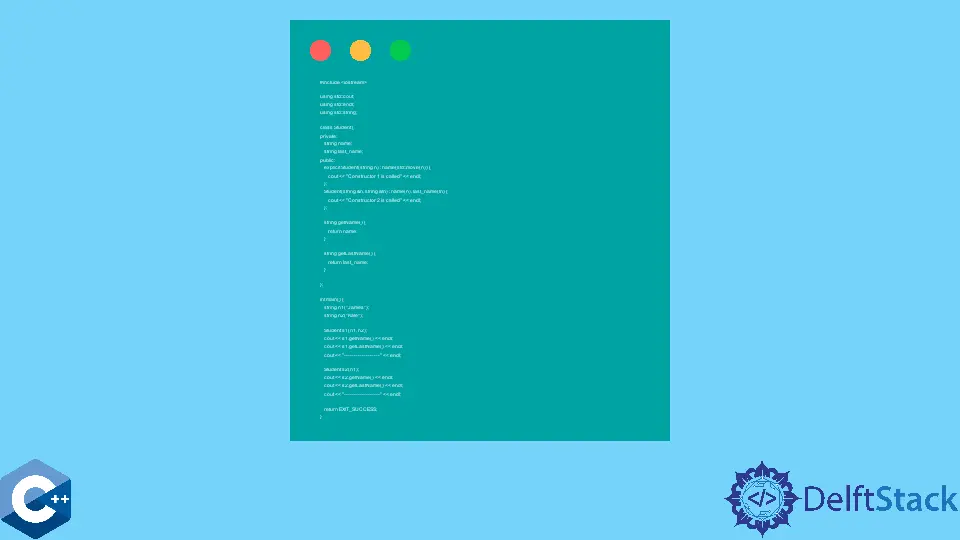
This article will explain how to implement overloaded constructors in C++.
Constructors Basics and Constructor Overloading in C++
Constructor is a special member function in the C++ class tasked to initialize the objects of the class. Constructors don’t have special names and can’t be called directly, but each time a given class is created, a corresponding constructor is usually invoked. Although, we declare constructors with the same name as the class itself. Since C++ provides a way to define multiple functions with the same name in a given scope, we also can define multiple constructors for a single class.
Note that function overloading rules apply similarly to the overloaded constructors, as they need to differ in the number of parameters or types they accept. C++ designates different types of constructors, which often have specific use cases, but we will not delve into these details in this article because of its massive scope.
In the following example code, the Student
class is defined, including two private
data members of type string
. These members are supposed to be initialized when creating a new instance of the Student
class, but let’s assume that only name
member is mandatory. Consequently, we need to have different methods to construct the object of this class, one of which only initializes name
member.
Thus, we define the first constructor that takes only one string
parameter and prints the corresponding message to the console. The latter step is only used to identify the behavior of constructors easily. Additionally, we also define a second constructor that accepts two string
parameters and initializes both data members - name
and last_name
.
#include <iostream>
using std::cout;
using std::endl;
using std::string;
class Student {
private:
string name;
string last_name;
public:
explicit Student(string n) : name(std::move(n)) {
cout << "Constructor 1 is called" << endl;
};
Student(string &n, string &ln) : name(n), last_name(ln) {
cout << "Constructor 2 is called" << endl;
};
string getName() { return name; }
string getLastName() { return last_name; }
};
int main() {
string n1("James");
string n2("Kale");
Student s1(n1, n2);
cout << s1.getName() << endl;
cout << s1.getLastName() << endl;
cout << "------------------" << endl;
Student s2(n1);
cout << s2.getName() << endl;
cout << s2.getLastName() << endl;
cout << "------------------" << endl;
return EXIT_SUCCESS;
}
Output:
Constructor 2 is called
James
Kale
------------------
Constructor 1 is called
James
------------------
Notice that when we create the s1
object in the main
program, the second constructor is invoked since we provided two arguments, and when s2
is initialized, the first constructor gets called. In this case, we had two constructors with a different number of parameters, but in general, we can define two constructors with the same number of parameters that differ in types they accept.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook