The Addition Assignment Operator and Increment Operator in C++
- Understanding the Addition Assignment Operator (+=)
- Exploring the Increment Operator (++)
- When to Use These Operators
- Conclusion
- FAQ
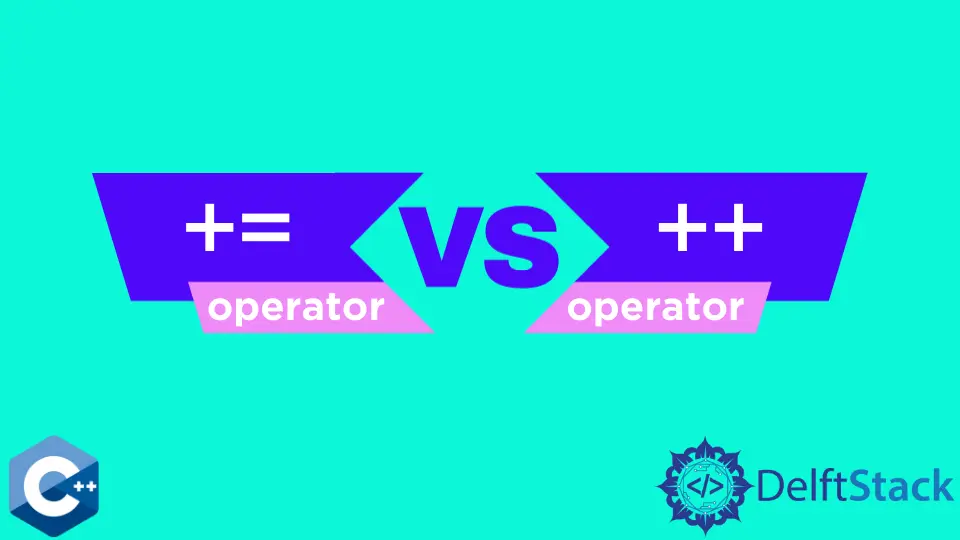
When diving into the world of C++, understanding operators is crucial for mastering the language. Among these operators, the addition assignment operator (+=) and the increment operator (++) play significant roles in simplifying your code and enhancing its readability. These operators not only save time but also make your code cleaner and more efficient.
In this article, we will explore what these operators are, how they function, and when to use them effectively. Whether you’re a beginner or looking to brush up on your C++ skills, this guide will provide you with the insights you need to leverage these powerful tools in your programming toolkit.
Understanding the Addition Assignment Operator (+=)
The addition assignment operator (+=) is a shorthand way of adding a value to a variable and then assigning the result back to that variable. Instead of writing x = x + y
, you can simply write x += y
. This operator is particularly useful in loops or when you want to accumulate values over time. Let’s take a look at how it works in practice.
#include <iostream>
using namespace std;
int main() {
int x = 5;
int y = 10;
x += y;
cout << "The value of x after addition assignment: " << x << endl;
return 0;
}
Output:
The value of x after addition assignment: 15
In this example, we initialized x
with the value of 5 and y
with 10. By using the addition assignment operator, we added y
to x
and assigned the result back to x
. The output shows that x
now holds the value of 15. This operator is not only concise but also improves code readability, making it easier to understand the operations being performed.
Exploring the Increment Operator (++)
The increment operator (++) is another handy tool in C++. It increases the value of a variable by one. There are two forms of the increment operator: prefix and postfix. The prefix version (++x
) increments the variable before its value is used, while the postfix version (x++
) increments the variable after its value is used. Understanding the difference between these two forms is vital for effective programming.
#include <iostream>
using namespace std;
int main() {
int x = 5;
cout << "Value of x before increment: " << x << endl;
cout << "Using prefix increment: " << ++x << endl;
cout << "Value of x after prefix increment: " << x << endl;
cout << "Using postfix increment: " << x++ << endl;
cout << "Value of x after postfix increment: " << x << endl;
return 0;
}
Output:
Value of x before increment: 5
Using prefix increment: 6
Value of x after prefix increment: 6
Using postfix increment: 6
Value of x after postfix increment: 7
In this code snippet, we start with x
set to 5. When we use the prefix increment operator (++x
), x
is incremented to 6 before its value is printed. However, when we use the postfix increment operator (x++
), the original value of x
(which is 6) is printed first, and then x
is incremented to 7. This distinction is essential in scenarios where the order of operations matters, such as in loops or complex expressions.
When to Use These Operators
Knowing when to use the addition assignment and increment operators can greatly enhance your coding efficiency. The addition assignment operator is particularly useful in scenarios where you need to accumulate values, such as in summing elements of an array or in iterative calculations. It helps to keep your code neat and reduces the likelihood of errors associated with repetitive variable assignments.
On the other hand, the increment operator is invaluable in loop control. For example, in a for
loop, using i++
or ++i
allows you to increment the loop counter easily. Choosing between prefix and postfix forms depends on your specific needs. If you need the incremented value immediately, opt for the prefix version. If the original value suffices before incrementing, the postfix version is the way to go.
In summary, both operators simplify your code and make it more readable. They help reduce redundancy and improve the overall efficiency of your programming. By incorporating these operators into your coding practices, you can write cleaner, more maintainable code.
Conclusion
In conclusion, the addition assignment operator (+=) and the increment operator (++) are essential tools in the C++ programming language. They not only streamline your code but also enhance its readability and efficiency. By mastering these operators, you can write more concise and effective programs, making your journey through C++ much smoother. Whether you’re working on simple calculations or complex algorithms, these operators will undoubtedly become invaluable assets in your coding arsenal.
FAQ
-
What is the difference between the addition assignment operator and the increment operator?
The addition assignment operator (+=) adds a value to a variable and assigns it back, while the increment operator (++) simply increases a variable’s value by one. -
Can I use the addition assignment operator with different data types?
Yes, the addition assignment operator can be used with various data types, including integers, floats, and even strings in C++. -
Is there any performance difference between using
x += y
andx = x + y
?
In most cases, usingx += y
is more efficient as it reduces redundancy, making the code cleaner and potentially faster. -
When should I use prefix increment versus postfix increment?
Use prefix increment when you need the incremented value immediately, and use postfix when you want to use the original value before incrementing. -
Are there any scenarios where using these operators might lead to errors?
Yes, misuse of the increment operator, especially in complex expressions, can lead to unexpected results. It’s essential to understand their behavior in different contexts.