How to Count Number of Digits in a Number in C++
-
Use
std::to_string
andstd::string::size
Functions to Count Number of Digits in a Number in C++ -
Use
std::string::erase
andstd::remove_if
Methods to Count to Count Number of Digits in a Number in C++
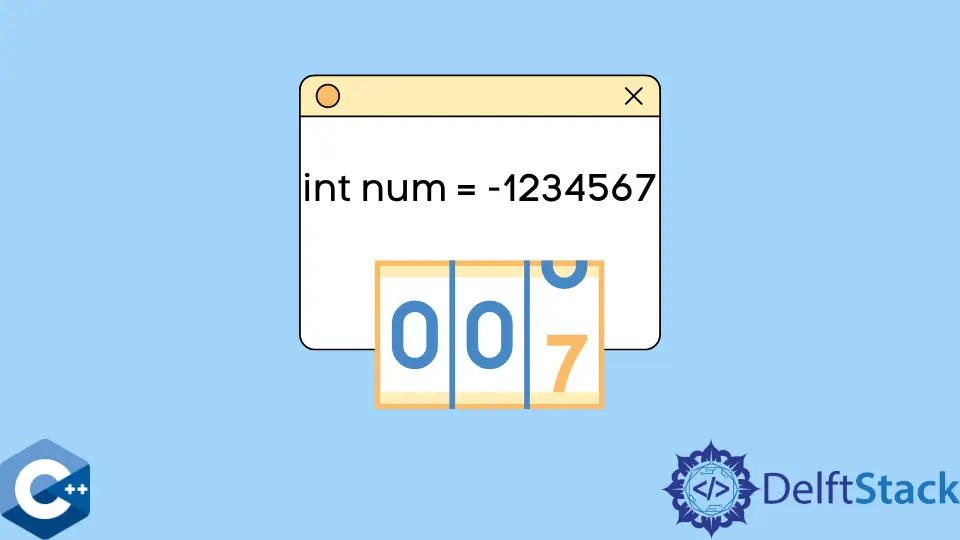
This article will demonstrate multiple methods about how to count the number of digits in a number C++.
Use std::to_string
and std::string::size
Functions to Count Number of Digits in a Number in C++
The most straightforward way to count the number of digits in a number is to convert it to the std::string
object and then call a built-in function of the std::string
to retrieve a count number. In this case, we implemented a separate templated function countDigits
that takes a single argument, which is assumed to be an integer type and returns the size as an integer. Note that the following example outputs incorrect numbers for negative integers since it counts the sign symbol in too.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
template <typename T>
size_t countDigits(T n) {
string tmp;
tmp = to_string(n);
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
Output:
number of digits in 1234567 = 7
number of digits in -1234567 = 8
To remedy the flaw in the previous implementation of function countDigits
, we will add a single if
statement to evaluate if the given number is negative and return the string size that is one less. Notice that if the number is greater than 0
, the original value of string size is returned, as implemented in the following example code.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
template <typename T>
size_t countDigits(T n) {
string tmp;
tmp = to_string(n);
if (n < 0) return tmp.size() - 1;
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
Output:
number of digits in 1234567 = 7
number of digits in -1234567 = 7
Use std::string::erase
and std::remove_if
Methods to Count to Count Number of Digits in a Number in C++
The previous example provides a fully sufficient solution for the above problem, but one can over-engineer the countDigits
using the std::string::erase
and std::remove_if
functions combination to remove any non-number symbols. Note also that this method may be the stepping stone to implement a function that can work with floating-point values. Mind though, the following sample code is not compatible with floating-point values.
#include <iostream>
#include <string>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::string;
using std::to_string;
template <typename T>
size_t countDigits(T n) {
string tmp;
tmp = to_string(n);
tmp.erase(std::remove_if(tmp.begin(), tmp.end(), ispunct), tmp.end());
return tmp.size();
}
int main() {
int num1 = 1234567;
int num2 = -1234567;
cout << "number of digits in " << num1 << " = " << countDigits(num1) << endl;
cout << "number of digits in " << num2 << " = " << countDigits(num2) << endl;
exit(EXIT_SUCCESS);
}
Output:
number of digits in 1234567 = 7
number of digits in -1234567 = 7
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook