How to Use Nested if-else Statements in C++
-
Use Single
if-else
Statement to Implement Conditional Statement in C++ -
Use Nested
if-else
Statements to Implement Multi-Conditional Program Control Flow in C++
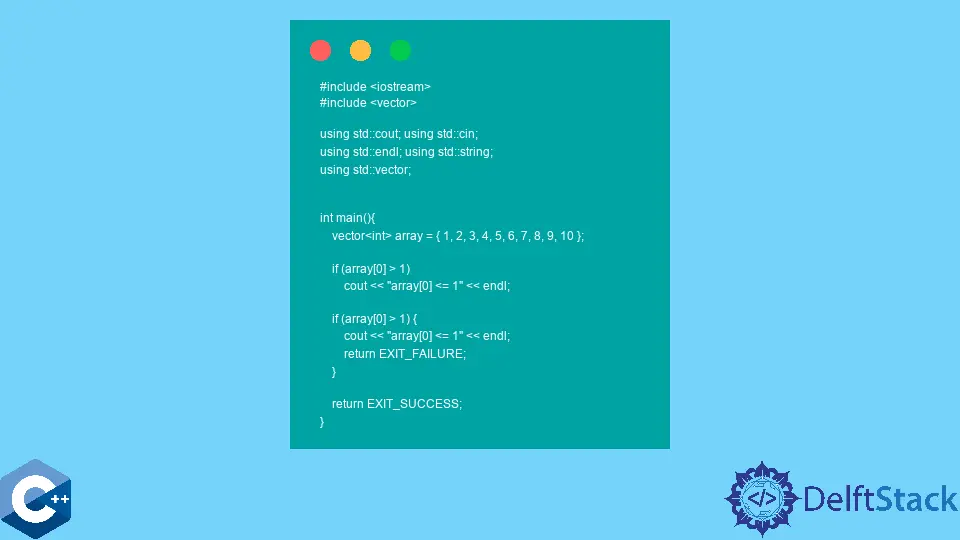
This article will explain several methods of how to use nested if-else statements in C++.
Use Single if-else
Statement to Implement Conditional Statement in C++
There are two kinds of statements provided by the C++ language to implement conditional execution. One is the if
statement - which branches the control flow based on a condition, and the other is the switch
statement that evaluates the expression to choose one of the possible paths of execution. The if
statement can be expressed as a single condition or constructed as multi-step statements that control the different execution paths. Note that when the single statement follows the condition, it can be written without braces {}
to distinguish the block scope.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
if (array[0] > 1) cout << "array[0] <= 1" << endl;
if (array[0] > 1) {
cout << "array[0] <= 1" << endl;
return EXIT_FAILURE;
}
return EXIT_SUCCESS;
}
Use Nested if-else
Statements to Implement Multi-Conditional Program Control Flow in C++
Alternatively, one can chain the nested if-else
statements inside each other to implement complex conditional control flow. Note that missing braces for the given if-else
would mean that the block is executed without condition. The latter scenario is most likely when multiple if
statements are nested and not all if
conditions have the corresponding else
block on the same level. To avoid the problems like this, one should try to enforce braces style or use some IDE-specific tools to detect such issues in the code.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
if (array[4] > array[3]) {
cout << "array[4] > array[3]" << endl;
if (array[4] > array[5])
cout << "array[4] > array[5]" << endl;
else
cout << "array[4] <= array[5]" << endl;
}
if (array[4] > array[3]) {
cout << "array[4] > array[3]" << endl;
if (array[4] > array[5])
cout << "array[4] > array[5]" << endl;
else if (array[4] > array[6])
cout << "array[4] > array[6]" << endl;
else if (array[4] < array[5])
cout << "array[4] < array[5]" << endl;
else
cout << "array[4] == array[5]" << endl;
}
return EXIT_SUCCESS;
}
Output:
array[4] > array[3]
array[4] <= array[5]
array[4] > array[3]
array[4] < array[5]
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook