Namespaces in C++
- What Are Namespaces?
- How to Define a Namespace
- Using Multiple Namespaces
-
Using the
using
Directive - Best Practices for Using Namespaces
- Conclusion
- FAQ
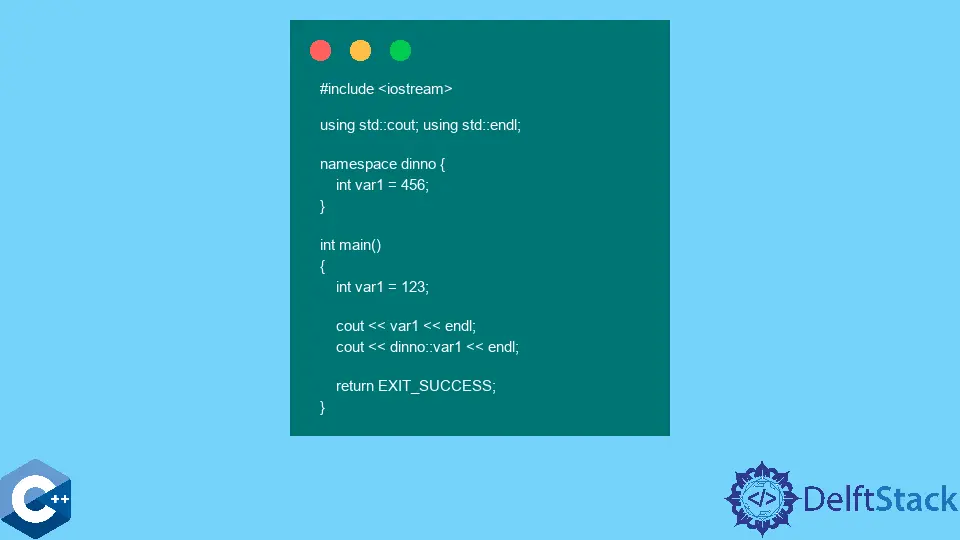
Namespaces in C++ are a powerful feature that helps manage the scope of variables, functions, and other identifiers. They play a crucial role in preventing naming conflicts, especially in large projects or when integrating multiple libraries. By using namespaces, developers can organize their code more clearly and avoid potential issues that arise from name collisions.
In this article, we will explore the concept of namespaces in C++, how to define and use them, and the best practices to follow to maximize their benefits. Whether you are a beginner or an experienced programmer, understanding namespaces is essential for writing clean, maintainable, and efficient C++ code.
What Are Namespaces?
A namespace is essentially a declarative region that provides a scope to identifiers such as variables, functions, and classes. By wrapping your code within a namespace, you create a unique context that allows you to reuse the same names in different parts of your program without causing ambiguity.
For example, consider two libraries that both define a function named calculate
. If both are included in your project without namespaces, the compiler won’t know which calculate
function to use, leading to errors. Namespaces help avoid this by allowing you to specify which calculate
you mean, like this: LibraryA::calculate()
or LibraryB::calculate()
.
How to Define a Namespace
Defining a namespace in C++ is straightforward. You simply use the namespace
keyword followed by the desired name. Here’s a simple example of how to create and use a namespace:
#include <iostream>
namespace MathFunctions {
void calculate() {
std::cout << "Calculating in MathFunctions namespace." << std::endl;
}
}
int main() {
MathFunctions::calculate();
return 0;
}
Output:
Calculating in MathFunctions namespace.
In this example, we defined a namespace called MathFunctions
that contains a function called calculate
. Inside the main()
function, we call this calculate
function by prefixing it with the namespace name. This clearly indicates where the function is defined, thus avoiding any potential conflicts with other functions named calculate
.
Using Multiple Namespaces
C++ allows you to define multiple namespaces, making it easy to organize your code logically. You can even nest namespaces within each other. Here’s how you can work with multiple namespaces:
#include <iostream>
namespace MathFunctions {
void calculate() {
std::cout << "Calculating in MathFunctions namespace." << std::endl;
}
}
namespace StringFunctions {
void calculate() {
std::cout << "Calculating in StringFunctions namespace." << std::endl;
}
}
int main() {
MathFunctions::calculate();
StringFunctions::calculate();
return 0;
}
Output:
Calculating in MathFunctions namespace.
Calculating in StringFunctions namespace.
In this example, we have two separate namespaces: MathFunctions
and StringFunctions
. Each namespace contains its own calculate
function. When we call these functions in the main()
function, we specify the namespace to avoid any ambiguity. This structure not only keeps your code organized but also enhances readability.
Using the using
Directive
While namespaces help avoid naming conflicts, they can also make your code verbose if you have to prefix every function call with the namespace name. To simplify this, C++ provides the using
directive, which allows you to bring an entire namespace or specific functions into the current scope. Here’s how it works:
#include <iostream>
namespace MathFunctions {
void calculate() {
std::cout << "Calculating in MathFunctions namespace." << std::endl;
}
}
using namespace MathFunctions;
int main() {
calculate();
return 0;
}
Output:
Calculating in MathFunctions namespace.
In this example, we used the using namespace MathFunctions;
directive, which allows us to call the calculate
function without the namespace prefix. While this can make the code cleaner, be cautious when using it, especially in larger files or projects, as it can lead to naming conflicts if two namespaces define the same function or variable.
Best Practices for Using Namespaces
When working with namespaces in C++, adhering to best practices can help you write cleaner and more maintainable code. Here are some tips to keep in mind:
-
Use namespaces for logical grouping: Organize your code into namespaces based on functionality or modules. This will help you and others understand the structure of your code more easily.
-
Avoid using directives in headers: If you have a header file, avoid using
using namespace
directives. This can lead to unexpected naming conflicts in the files that include your header. -
Use aliases for long namespaces: If you have a long namespace name, consider using an alias to make your code more readable. You can do this with the
namespace alias_name = namespace_name;
syntax. -
Be specific with
using
declarations: Instead of using the entire namespace, consider using specific functions or variables. For example,using MathFunctions::calculate;
is more precise thanusing namespace MathFunctions;
.
By following these best practices, you can harness the full potential of namespaces in C++ while keeping your code clean and understandable.
Conclusion
Namespaces are a vital feature in C++ that help manage the scope of identifiers and prevent naming conflicts. By understanding how to define and use namespaces effectively, you can write cleaner, more maintainable code. Remember to organize your code logically, avoid using directives in header files, and be specific when bringing names into scope. With these practices in mind, you’ll be well-equipped to leverage namespaces in your C++ projects, enhancing both clarity and functionality.
FAQ
-
What is a namespace in C++?
A namespace is a declarative region that provides a scope to identifiers such as variables, functions, and classes, helping to avoid naming conflicts. -
How do I define a namespace in C++?
You define a namespace using thenamespace
keyword followed by the desired name and enclosing the identifiers within curly braces. -
Can I have multiple namespaces in C++?
Yes, you can define multiple namespaces in C++, and you can even nest namespaces within each other for better organization. -
What is the
using
directive in C++?
Theusing
directive allows you to bring an entire namespace or specific functions into the current scope, making your code less verbose. -
What are best practices for using namespaces?
Organize code logically, avoid using directives in header files, use aliases for long namespaces, and be specific withusing
declarations.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook