Multiple Inheritance in C++
- Use Multiple Inheritance to Apply Multiple Properties of the Two Given Classes to Another
-
Use the
virtual
Keyword to Fix Multiple Copies of Base Classes
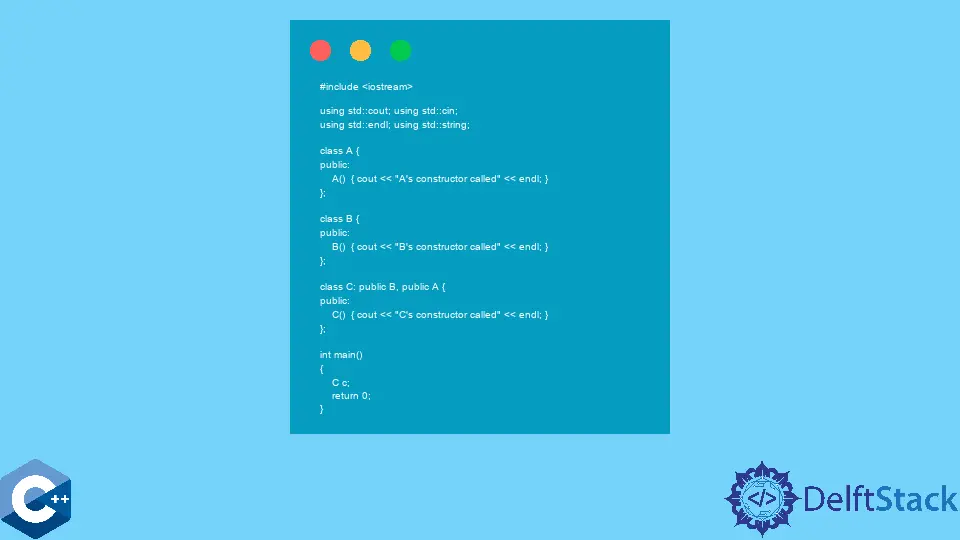
This article will demonstrate multiple methods about how to use multiple inheritances in C++.
Use Multiple Inheritance to Apply Multiple Properties of the Two Given Classes to Another
Classes in C++ can have multiple inheritances, which provides the possibility to derive a class from more than one direct base class.
This implies that there might be particular unusual behavior when the classes are not implemented carefully. E.g., consider the following snippet code, where the class C
is derived from class B
and A
. All of them have the default constructor that outputs the special string. Although, when we declare a C
type object in the mainc
function, three constructors print the output. Notice that constructors get called in the same order they were inherited. On the other hand, the destructors are called in reverse order.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
class A {
public:
A() { cout << "A's constructor called" << endl; }
};
class B {
public:
B() { cout << "B's constructor called" << endl; }
};
class C : public B, public A {
public:
C() { cout << "C's constructor called" << endl; }
};
int main() {
C c;
return 0;
}
Output:
Bs constructor called
As constructor called
Cs constructor called
Use the virtual
Keyword to Fix Multiple Copies of Base Classes
Note that the following program outputs the two instances of the Planet
class constructor, Mars
and Earth
constructors each, and finally the Rock
class constructor. Also, the Planet
class’s destructor will get called twice when the object goes out of scope. Mind though, this issue can be solved by adding the virtual
keyword to the Mars
and Earth
classes. When a class has multiple base classes, there is a possibility that the derived class will inherit a member with the same name from two or more of its base classes.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
class Planet {
public:
Planet(int x) { cout << "Planet::Planet(int ) called" << endl; }
};
class Mars : public Planet {
public:
Mars(int x) : Planet(x) { cout << "Mars::Mars(int ) called" << endl; }
};
class Earth : public Planet {
public:
Earth(int x) : Planet(x) { cout << "Earth::Earth(int ) called" << endl; }
};
class Rock : public Mars, public Earth {
public:
Rock(int x) : Earth(x), Mars(x) { cout << "Rock::Rock(int ) called" << endl; }
};
int main() { Rock tmp(30); }
Output:
Planet::Planet(int ) called
Mars::Mars(int ) called
Planet::Planet(int ) called
Earth::Earth(int ) called
Rock::Rock(int ) called
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook