The std::min_element Algorithm From STL in C++
-
Use the
std::min_element
Algorithm to Find the Smallest Element in Range in C++ -
Use the
std::minmax_element
Algorithm to Find the Smallest Element and the Largest Element in Range
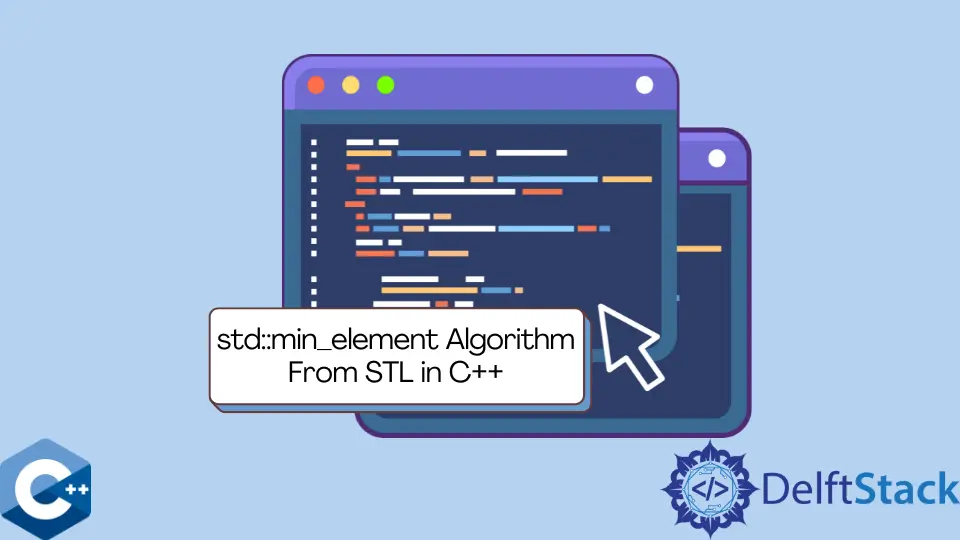
This article will explain how to use std::min_element
algorithm from STL in C++.
Use the std::min_element
Algorithm to Find the Smallest Element in Range in C++
STL algorithms include generic minimum/maximum searching functions that can operate on container ranges. std::min_element
is one of these functions that retrieves the smallest element in the given range. The elements are compared with operator<
when the comparison function is not explicitly specified. So if the container stores the user-defined objects, it must have the corresponding operator defined.
The range should satisfy the requirements of LegacyForwardIterator
. The std::min_element
function accepts two iterators and returns the iterator to the smallest element in the range. If the range includes multiple instances of the smallest element, the algorithm returns the iterator to the first of them. When the given range is empty, the second argument iterator is returned.
The following example shows the simple usage of std::min_element
for the vector
container. Notice that when we want the numeric position of the smallest element, one should utilize std::distance
function to calculate it.
#include <algorithm>
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<string> v1 = {"ztop", "htop", "mtop", "ktop", "ptop"};
vector<int> v2 = {111, 43, 12, 41, 34, 54, 13};
auto ret = std::min_element(v1.begin(), v1.end());
cout << "min_element(v1): " << std::setw(4) << *ret
<< " at position: " << std::distance(v1.begin(), ret) << endl;
auto ret2 = std::min_element(v2.begin(), v2.end());
cout << "min_element(v2): " << std::setw(4) << *ret2
<< " at position: " << std::distance(v2.begin(), ret2) << endl;
return EXIT_SUCCESS;
}
min_element(v1): htop at position: 1
min_element(v2): 12 at position: 2
Use the std::minmax_element
Algorithm to Find the Smallest Element and the Largest Element in Range
Another powerful algorithm included in STL is std::minmax_element
. It retrieves both the smallest and the greatest elements in the given range. std::minmax_element
returns std::pair
value storing the smallest element as the first member. Note that this algorithm returns the last element when there are multiple values qualifying for the greatest value in range. Mind that both of these algorithms also have overloads that can include the third parameter denoting the custom comparison function.
#include <algorithm>
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<string> v1 = {"ztop", "htop", "mtop", "ktop", "ptop"};
vector<int> v2 = {111, 43, 12, 41, 34, 54, 13};
auto ret3 = std::minmax_element(v1.begin(), v1.end());
cout << "min (v1): " << std::setw(4) << *ret3.first
<< "| max (v1): " << std::setw(4) << *ret3.second << endl;
auto ret4 = std::minmax_element(v2.begin(), v2.end());
cout << "min (v2): " << std::setw(4) << *ret4.first
<< "| max (v2): " << std::setw(4) << *ret4.second << endl;
return EXIT_SUCCESS;
}
Output:
min (v1): htop| max (v1): ztop
min (v2): 12| max (v2): 111
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook