How to Find Length of a String in C++
-
Use the
length
Function to Find Length of a String in C++ -
Use the
size
Function to Find Length of a String in C++ -
Use the
while
Loop to Find Length of a String in C++ -
Use the
std::strlen
Function to Find Length of a String in C++
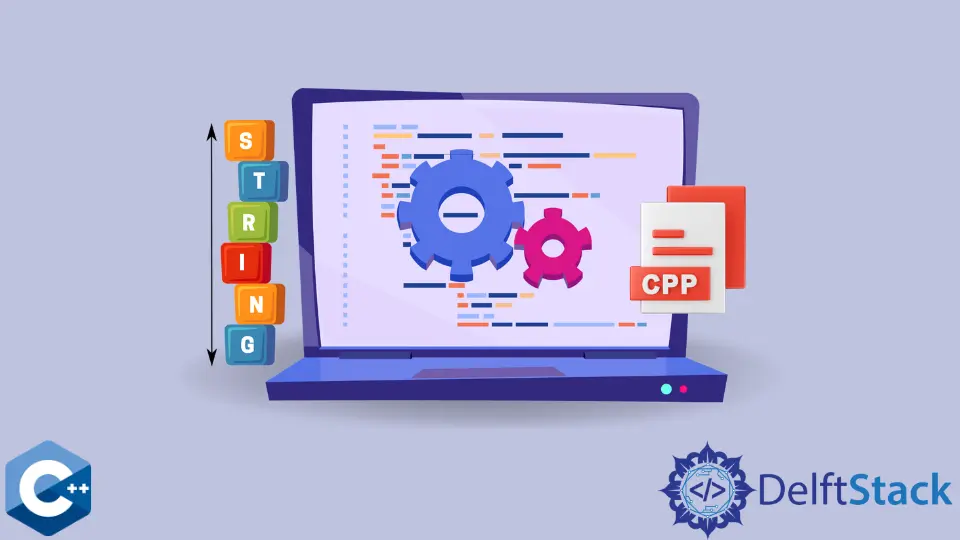
This article will explain several methods of how to find the length of a string in C++.
Use the length
Function to Find Length of a String in C++
The C++ standard library provides the std::basic_string
class to augment char
-like sequences and implement a generic structure for storing and manipulating such data. Although, most people are more familiar with std::string
type which is itself type alias for std::basic_string<char>
. std::string
provides length
built-in function to retrieve the length of the stored char
sequence.
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << str1.length() << endl;
exit(EXIT_SUCCESS);
}
Output:
string: this is random string oiwaoj
length: 28
Use the size
Function to Find Length of a String in C++
Another built-in function included in the std::string
class is size
, which behaves similarly to the previous method. It takes no arguments and returns the number of char
elements in the string object.
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << str1.size() << endl;
exit(EXIT_SUCCESS);
}
Output:
string: this is random string oiwaoj
length: 28
Use the while
Loop to Find Length of a String in C++
Alternatively, one can implement his/her own function to calculate the length of the string. In this case, we utilize the while
loop to traverse the string as a char
sequence and increment the counter by one each iteration. Notice that the function takes char*
as an argument, and the c_str
method is called to retrieve this pointer in the main function. The loop stops when the dereferenced pointer value is equal to 0
, and the null-terminated string implementation guarantees that.
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
size_t lengthOfString(const char *s) {
size_t size = 0;
while (*s) {
size += 1;
s += 1;
}
return size;
}
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << lengthOfString(str1.c_str()) << endl;
exit(EXIT_SUCCESS);
}
Output:
string: this is random string oiwaoj
length: 28
Use the std::strlen
Function to Find Length of a String in C++
Finally, one can resort to the old-school C string library function strlen
, which takes a single const char*
argument as our custom-defined function - lengthOfString
. These last two methods can be faulty when called on char
sequences that don’t end with a null byte, as it could access out-of-range memory during the traversal.
#include <cstring>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main(int argc, char *argv[]) {
string str1 = "this is random string oiwaoj";
cout << "string: " << str1 << endl;
cout << "length: " << std::strlen(str1.c_str()) << endl;
exit(EXIT_SUCCESS);
}
Output:
string: this is random string oiwaoj
length: 28
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++