How to Iterate Through a String in C++
- Use Range Based Loop to Iterate Through a String in C++
-
Use the
for
Loop to Iterate Through a String in C++
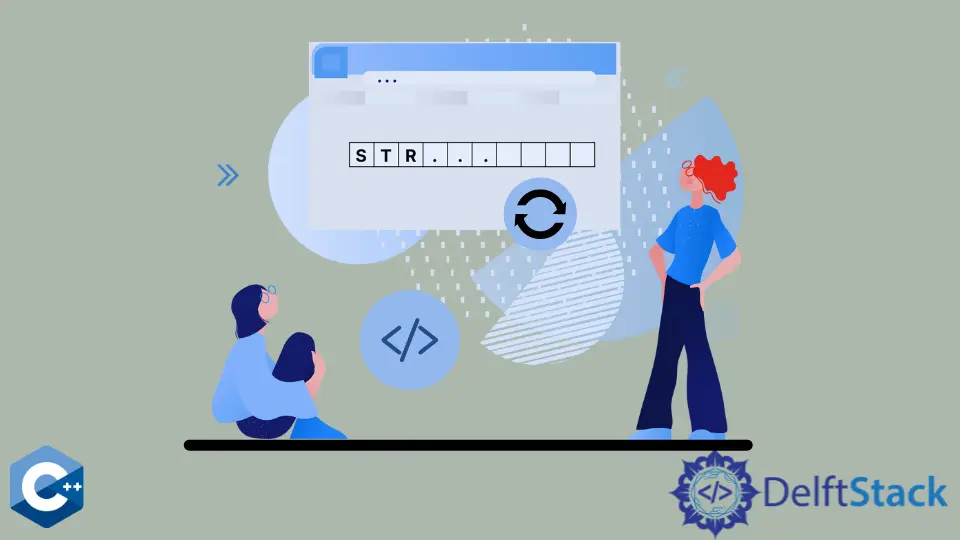
This article will introduce multiple methods of how to iterate through a string while keeping the index count in C++.
Use Range Based Loop to Iterate Through a String in C++
Modern C++ language style recommends the range based iteration for structures that support it. Meanwhile, the current index can be stored in a separate variable of type size_t
that will be incremented each iteration. Note that the increment is specified with the ++
operator at the end of the variable because putting it as a prefix would yield an index that starts with 1. The following example shows only the short part of the program output.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "They talk of days for which they sit and wait";
size_t index = 0;
for (char c : text) {
cout << index++ << " - '" << c << "'" << endl;
}
return EXIT_SUCCESS;
}
Output:
0 - 'T'
1 - 'h'
2 - 'e'
...
43 - 'i'
44 - 't'
Use the for
Loop to Iterate Through a String in C++
It has elegance and power in the conventional for
loop, as it offers flexibility when the inner scope involves matrix / multidimensional array operations. It’s also the preferred iteration syntax when using advanced parallelization techniques like the OpenMP standard.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "They talk of days for which they sit and wait";
for (int i = 0; i < text.length(); ++i) {
cout << i << " - '" << text[i] << "'" << endl;
}
return EXIT_SUCCESS;
}
Output:
0 - 'T'
1 - 'h'
2 - 'e'
...
43 - 'i'
44 - 't'
Alternatively, we can access the string’s individual characters with the at()
member function.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "They talk of days for which they sit and wait";
for (int i = 0; i < text.length(); ++i) {
cout << i << " - '" << text.at(i) << "'" << endl;
}
return EXIT_SUCCESS;
}
Output:
0 - 'T'
1 - 'h'
2 - 'e'
...
43 - 'i'
44 - 't'
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++