How to Instantiate a Template Class in C++
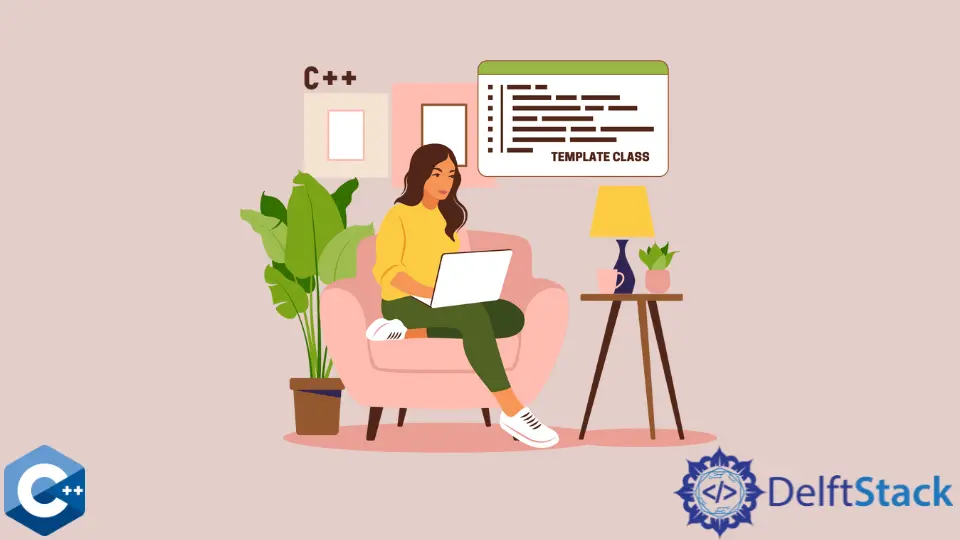
Template classes are used to create new classes that share the same implementation. They are mainly used to create an abstract base class that provides a general implementation of a particular type.
The template class is instantiated by specifying the data types and parameters for all the template class methods. The instantiated class can then be used as any other class in your program without needing to specify or create an object of that type.
Benefits of Using the Templates Classes
There are many advantages to using templates in C++. One advantage is that templates can be used to create generic functions, classes, and data structures.
This means they can be reused in many different programs without code modifications.
Another advantage is that they provide a way of generalizing the programming process, thus making it easier for programmers as they don’t have to spend time writing the same code repeatedly.
Steps to Instantiate a Template Class in C++
The following steps will help you instantiate a template class in C++:
-
Create a header file containing the template class declaration.
-
Create a
.cpp
file containing the template class’s implementation. -
Include the header file in the
.cpp
file and ensure it is placed before any other statements. -
Declare an object of your new type and initialize it with values as required.
-
Call functions on your object to use its features.
Example:
#include <iostream>
using namespace std;
template <class P>
class Demo {
private:
P sam;
public:
Demo(P m) : sam(m) {}
P getSam() { return sam; }
};
int main() {
Demo<int> demoSam(56);
cout << "int Number = " << demoSam.getSam() << endl;
return 0;
}
Click here to check the working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook