How to Implement Interfaces Using Abstract Class in C++
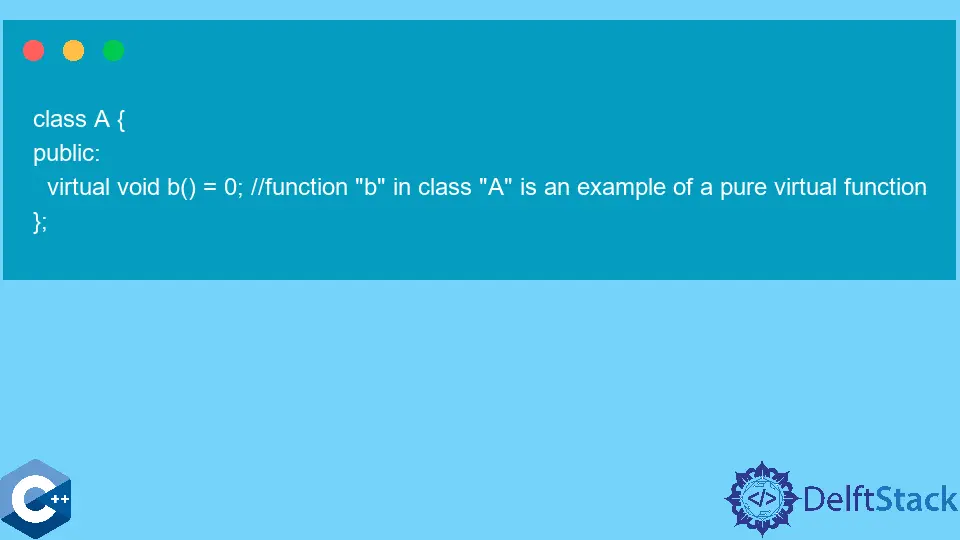
In Java, multiple inheritances are achieved using interface
. interface
is like a template because it contains the necessary layout but is abstract or has no method body.
When it comes to C++, there is no need for interfaces because C++ supports multiple inheritances, unlike Java. In C++, the functionality of interfaces can be achieved using abstract class.
Concept of Abstract Class in C++
An abstract class is a class with at least one pure virtual function. It is only possible to declare a pure virtual function, there can be no definition for it, and it is declared by assigning 0
in the declaration.
Abstract classes are very useful for making a code reusable and extendable. This is because it is possible to create many derived classes which inherit from the parent class but with their unique definitions.
Furthermore, abstract classes cannot be instantiated.
Example:
class A {
public:
virtual void b() = 0; // function "b" in class "A" is an example of a pure
// virtual function
};
During function declaration, trying to make the function pure virtual while defining it would not work.
Implement a Pure Virtual Function in a Program Using C++
Consider an example with shape
as the parent class. When it comes to shapes, there are many different types of them, and when we need to calculate properties, for example, area, the way we calculate it differs from shape to shape.
A rectangle
and triangle
as derived classes inheriting from the parent class of shape
. Then, we will provide the pure virtual function with the needed definition for each shape(rectangle
and triangle
) in their own derived class.
Source Code:
#include <iostream>
using namespace std;
class Shape {
protected:
int length;
int height;
public:
virtual int Area() = 0;
void getLength() { cin >> length; }
void getHeight() { cin >> height; }
};
class Rectangle : public Shape {
public:
int Area() { return (length * height); }
};
class Triangle : public Shape {
public:
int Area() { return (length * height) / 2; }
};
int main() {
Rectangle rectangle;
Triangle triangle;
cout << "Enter the length of the rectangle: ";
rectangle.getLength();
cout << "Enter the height of the rectangle: ";
rectangle.getHeight();
cout << "Area of the rectangle: " << rectangle.Area() << endl;
cout << "Enter the length of the triangle: ";
triangle.getLength();
cout << "Enter the height of the triangle: ";
triangle.getHeight();
cout << "Area of the triangle: " << triangle.Area() << endl;
return 0;
}
Output:
Enter the length of the rectangle: 2
Enter the height of the rectangle: 4
Area of the rectangle: 8
Enter the length of the triangle: 4
Enter the height of the triangle: 6
Area of the triangle: 12
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.