How to Use the ignore() Function in C++
-
Use the
ignore()
Function to Discard Unwanted Command Line User Input -
Use the
ignore
Function to Extract Initials of the User Input in C++
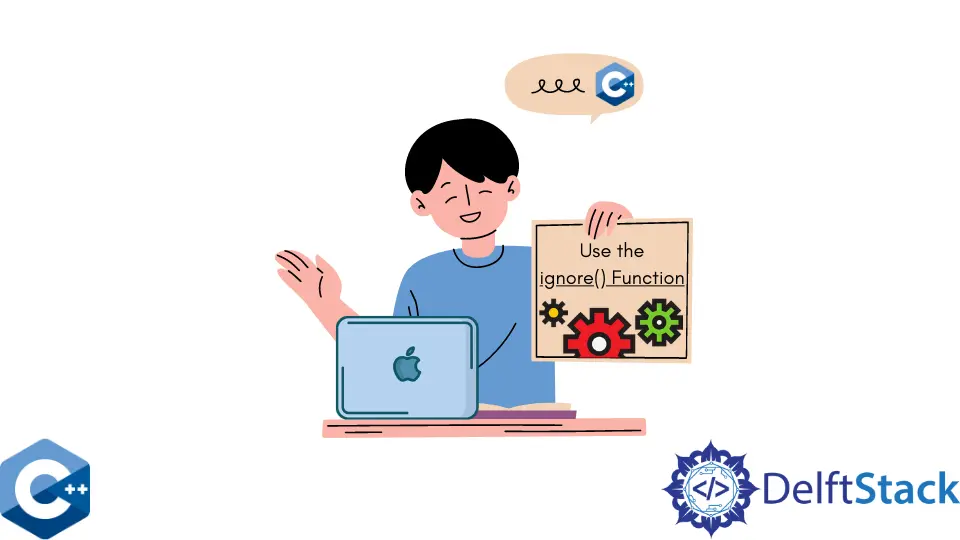
This article will explain several methods of how to use the ignore()
function in C++.
Use the ignore()
Function to Discard Unwanted Command Line User Input
The ignore()
function is a member function of std::basic_istream
and is inherited by different input stream classes. The function discards the characters in the stream until the given delimiter, inclusive, and then extracts the stream’s remainder.
ignore
takes two arguments; the first one is the number of characters to extract, and the second one is the delimiter character.
The following example shows how the ignore
function is called on the cin
object to store only 3 numbers and discard any other user input. Note that we use numeric_limits<std::streamsize>::max()
as the first argument of the ignore
function to force the special case and disable the number of characters parameter.
#include <fstream>
#include <iostream>
#include <limits>
#include <sstream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::numeric_limits;
using std::string;
int main() {
while (true) {
int i1, i2, i3;
cout << "Type space separated numbers: " << endl;
cin >> i1 >> i2 >> i3;
if (i1 == 0) exit(EXIT_SUCCESS);
cin.ignore(numeric_limits<std::streamsize>::max(), '\n');
cout << i1 << "; " << i2 << "; " << i3 << endl;
}
return EXIT_SUCCESS;
}
Output:
Type space separated numbers:
238 389 090 2232 89
238; 389; 90
Use the ignore
Function to Extract Initials of the User Input in C++
A common way of using the ignore
function is to seek the input stream to the desired delimiter and extract only needed characters.
In the following code sample, the user input is parsed so that only initials of the space-separated strings are extracted. Notice that we are using cin.get
twice to extract a single character from the stream, but cin.ignore
statement between them ensures that every character before and including the next space is discarded.
#include <fstream>
#include <iostream>
#include <limits>
#include <sstream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::numeric_limits;
using std::string;
using std::vector;
int main() {
char name, surname;
cout << "Type your name and surname: " << endl;
name = cin.get();
cin.ignore(numeric_limits<std::streamsize>::max(), ' ');
surname = cin.get();
cout << "Your initials are: " << name << surname << endl;
return EXIT_SUCCESS;
}
Output:
Type your name and surname:
Tim Cook
Your initials are: TM
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook