How to Use a PI Constant in C++
-
Use
M_PI
Macro From GNU C Library -
Use
std::numbers::pi
Constant From C++20 - Declare You Own PI Constant Variable
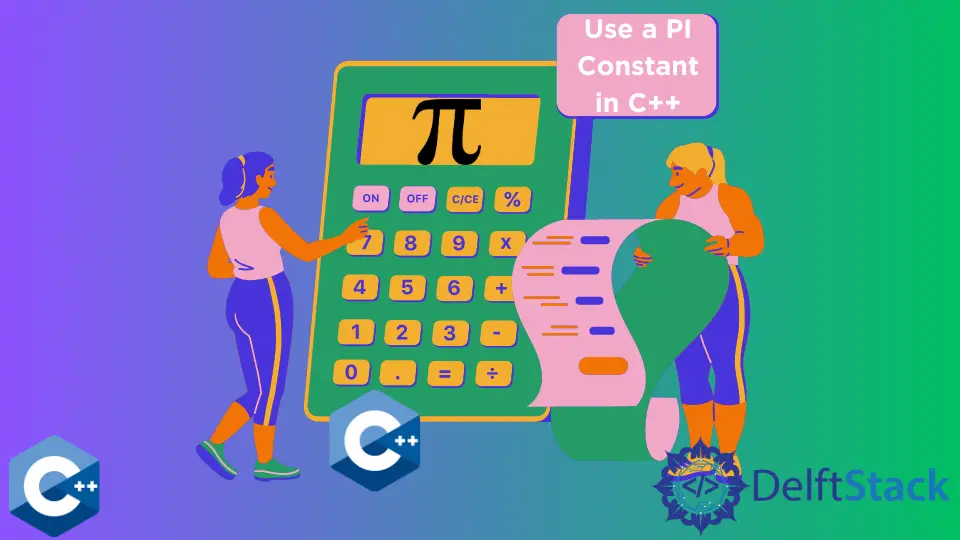
This article will introduce different ways to declare and use PI constant value in C++.
Use M_PI
Macro From GNU C Library
It uses predefined macro expression from C standard math library. The library defines multiple common mathematical constants, which are listed in the following table. M_PI
macro can be assigned to a floating point variable or used as a literal value in calculations. Notice that we are using the setprecision
manipulator function, which can be used to control the precision to which the output number is displayed.
Constant | Definition |
---|---|
M_E |
The base of natural logarithms |
M_LOG2E |
The logarithm to base 2 of M_E |
M_LOG10E |
The logarithm to base 10 of M_E |
M_LN2 |
The natural logarithm of 2 |
M_LN10 |
The natural logarithm of 10 |
M_PI |
Pi, the ratio of a circle’s circumference to its diameter |
M_PI_2 |
Pi divided by two |
M_PI_4 |
Pi divided by four |
M_1_PI |
The reciprocal of pi (1/pi) |
M_2_PI |
Two times the reciprocal of pi |
M_2_SQRTPI |
Two times the reciprocal of the square root of pi |
M_SQRT2 |
The square root of two |
M_SQRT1_2 |
The reciprocal of the square root of two (also the square root of 1/2) |
#include <cmath>
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
int main() {
double pi1 = M_PI;
cout << "pi = " << std::setprecision(16) << M_PI << endl;
cout << "pi * 2 = " << std::setprecision(16) << pi1 * 2 << endl;
cout << "M_PI * 2 = " << std::setprecision(16) << M_PI * 2 << endl;
cout << endl;
return EXIT_SUCCESS;
}
Output:
pi = 3.141592653589793
pi * 2 = 6.283185307179586
M_PI * 2 = 6.283185307179586
Use std::numbers::pi
Constant From C++20
Since the C++20 standard, the language supports the mathematical constants defined in the <numbers>
header. These constants are supposed to offer better cross-platform compliance, but it is still in the early days, and various compilers might not support it yet. The full list of the constants can be seen here.
#include <iomanip>
#include <iostream>
#include <numbers>
using std::cout;
using std::endl;
int main() {
cout << "pi = " << std::setprecision(16) << std::numbers::pi << endl;
cout << "pi * 2 = " << std::setprecision(16) << std::numbers::pi * 2 << endl;
cout << endl;
return EXIT_SUCCESS;
}
pi = 3.141592653589793
pi * 2 = 6.283185307179586
Declare You Own PI Constant Variable
Alternatively, we can declare a custom constant variable with PI value or any other mathematical constant as needed. It can be achieved either using a macro expression or the constexpr
specifier for a variable. The following sample code demonstrates the use of both methods.
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
#define MY_PI 3.14159265358979323846
constexpr double my_pi = 3.141592653589793238462643383279502884L;
int main() {
cout << std::setprecision(16) << MY_PI << endl;
cout << std::setprecision(16) << my_pi << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook