How to Reverse a String in C++
- Use String Constructor to Reverse a String
-
Use
std::reverse()
Algorithm to Reverse a String -
Use
std::copy()
Algorithm to Reverse String
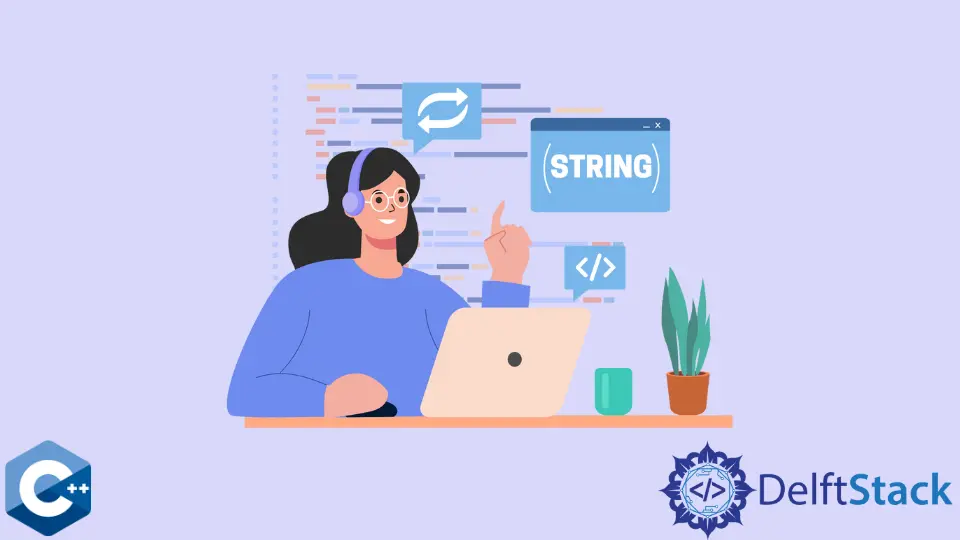
This article will explain how to reverse a string in C++.
Use String Constructor to Reverse a String
std::basic_string
has the constructor, which can build a string with the contents of the range. We can then declare a new string variable and feed its constructor the reverse iterators of the original string variable - tmp_s
. The following example demonstrates this method and outputs both strings for verification.
#include <algorithm>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed(tmp_s.rbegin(), tmp_s.rend());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
Output:
This string will be reversed
desrever eb lliw gnirts sihT
Use std::reverse()
Algorithm to Reverse a String
The std::reverse
method is from the <algorithm>
STL library, and it reverses the order of the elements in the range. The method operates on objects passed as arguments and does not return a new copy of the data, so we need to declare another variable to preserve the original string.
Note that the reverse
function throws std::bad_alloc
exception if the algorithm fails to allocate memory.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed(tmp_s);
reverse(tmp_s_reversed.begin(), tmp_s_reversed.end());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
Use std::copy()
Algorithm to Reverse String
std::copy
is another powerful algorithm, which can be utilized for multiple scenarios. This solution initializes a new string variable and modifies its size using the built-in resize
method. Next, we call the copy
method to fill the declared string with the original string’s data. Note, though, the first two parameters should be reverse iterators of the source range.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed;
tmp_s_reversed.resize(tmp_s.size());
copy(tmp_s.rbegin(), tmp_s.rend(), tmp_s_reversed.begin());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
In case the reversed string data need not be stored, we can use the copy()
algorithm to directly output the string data in reverse order to the console as shown in the following code sample:
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
copy(tmp_s.rbegin(), tmp_s.rend(), std::ostream_iterator<char>(cout, ""));
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++