How to Return an Array From a Function in C++
- Use Pointer Manipulation to Return C-Style Array From the Function in C++
-
Use
vector
Container to Return Array From the Function in C++
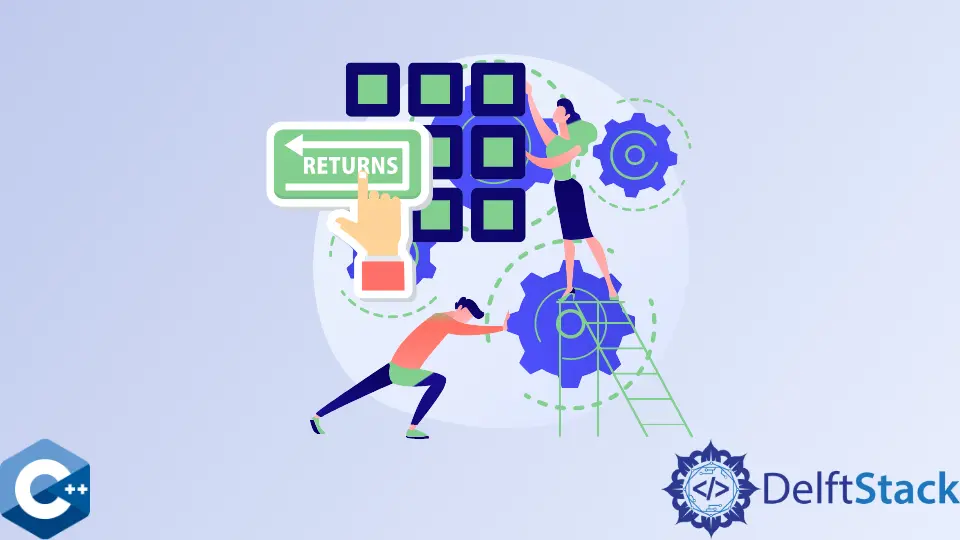
This article will introduce how to return an array from the function in C++.
Use Pointer Manipulation to Return C-Style Array From the Function in C++
In C/C++, when array[]
notation is passed as a function parameter, it’s just a pointer to the first element of the array handed over. Therefore the function prototype we need to construct is the one that returns the pointer to the type stored in the array; in our case it is int
. Once the function returns, we can access its elements with an array notation []
or dereferencing the pointer itself (maybe do some pointer arithmetic as before this).
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int *MultiplyArrayByTwo(int arr[], int size) {
for (int i = 0; i < size; ++i) {
arr[i] *= 2;
}
return arr;
}
int main() {
constexpr int size = 10;
int c_array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int *ptr = MultiplyArrayByTwo(c_array, size);
cout << "array = [ ";
for (int i = 0; i < size; ++i) {
cout << ptr[i] << ", ";
}
cout << "]" << endl;
return EXIT_SUCCESS;
}
Output:
array = [ 2, 4, 6, 8, 10, 12, 14, 16, 18, 20, ]
Notice that the MultiplyArrayByTwo
function could be implemented as void
and we could access modified elements from the c_array
variable since they point to the same address, as shown in the following example:
#include <iostream>
using std::cin;
using std::cout;
using std::endl using std::string;
void MultiplyArrayByTwo(int arr[], int size) {
for (int i = 0; i < size; ++i) {
arr[i] *= 2;
}
}
int main() {
constexpr int size = 10;
int c_array[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
MultiplyArrayByTwo(c_array, size);
cout << "array = [ ";
for (int i = 0; i < size; ++i) {
cout << c_array[i] << ", ";
}
cout << "]" << endl;
return EXIT_SUCCESS;
}
Use vector
Container to Return Array From the Function in C++
In this version, we store our array in a vector
container, which can dynamically scale its elements, so there is no need to pass the size
parameter.
Notice that we return a different object from the function, so we can’t use cpp_array
to access it. We need to create a new variable and assign the function return value to it. Then we can iterate through it and output the result.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::vector;
vector<int> MultiplyArrayByTwo(const vector<int> *arr) {
vector<int> tmp{};
for (const auto &item : *arr) {
tmp.push_back(item * 2);
}
return tmp;
}
int main() {
vector<int> cpp_array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto cpp_array_2x = MultiplyArrayByTwo(&cpp_array);
cout << "array = [ ";
for (int i : cpp_array_2x) {
cout << i << ", ";
}
cout << "]" << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ Function
- C++ Redefinition of Formal Parameter
- The ShellExecute() Function in C++
- The const Keyword in Function Declaration of Classes in C++
- How to Handle Arguments Using getopt in C++
- Time(NULL) Function in C++
- Cotangent Function in C++