How to Print Out the Contents of a Vector in C++
- Method 1: Using a Simple Loop
- Method 2: Using Range-Based For Loop
-
Method 3: Using the
std::copy
Function -
Method 4: Using
std::for_each
- Method 5: Using a Custom Function
- Conclusion
- FAQ
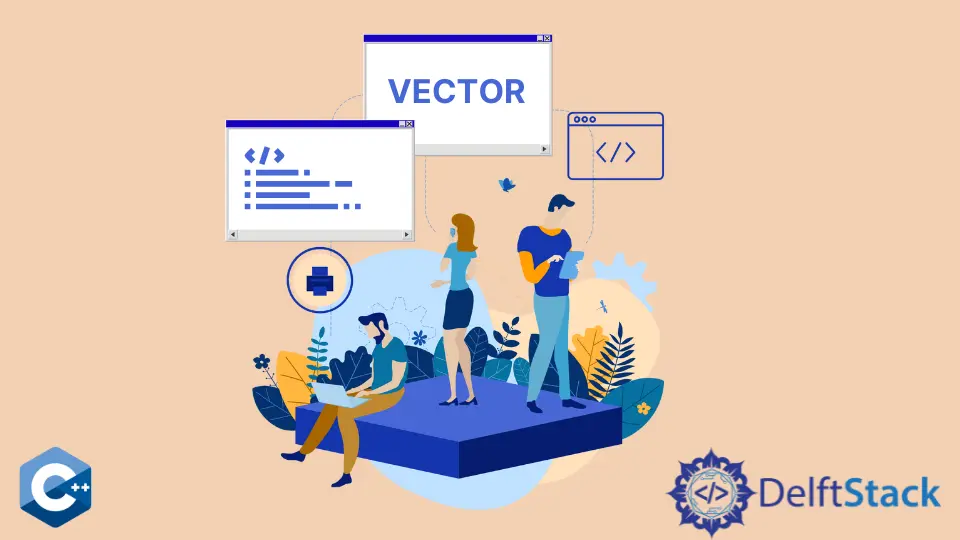
Printing the contents of a vector in C++ is a fundamental skill that every programmer should master. Vectors are dynamic arrays that can grow and shrink in size, making them incredibly versatile for managing collections of data. When you want to visualize or debug the data stored in a vector, printing its contents to standard output (stdout) is essential.
In this article, we will explore various methods to achieve this, providing you with clear examples and explanations to enhance your understanding. Whether you are a beginner or an experienced developer, these techniques will help you effectively display vector contents in your C++ applications.
Method 1: Using a Simple Loop
One of the most straightforward ways to print the contents of a vector in C++ is by using a simple for loop. This method allows you to iterate through each element of the vector and print it individually. Here’s how you can do it:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (size_t i = 0; i < numbers.size(); ++i) {
std::cout << numbers[i] << " ";
}
return 0;
}
Output:
1 2 3 4 5
In this example, we first include the necessary headers and create a vector of integers called numbers
. We then use a for loop to iterate through the vector using its size. The std::cout
statement prints each element followed by a space. This method is simple and effective, especially for beginners learning C++. However, it can become cumbersome for larger vectors or more complex data types.
Method 2: Using Range-Based For Loop
C++11 introduced range-based for loops, which provide a more elegant way to iterate through containers like vectors. This method simplifies the syntax and improves readability. Here’s how you can use it to print a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<std::string> fruits = {"Apple", "Banana", "Cherry", "Date"};
for (const auto& fruit : fruits) {
std::cout << fruit << " ";
}
return 0;
}
Output:
Apple Banana Cherry Date
In this example, we create a vector of strings called fruits
. The range-based for loop iterates through each element in the vector, binding each element to fruit
. The const auto&
syntax ensures that we are not making unnecessary copies of the elements. This method is particularly useful for printing elements of any data type, making it a versatile choice for developers.
Method 3: Using the std::copy
Function
Another efficient way to print vector contents is by using the std::copy
function along with an output stream iterator. This method is less common but can be very powerful. Here’s how you can implement it:
#include <iostream>
#include <vector>
#include <algorithm>
#include <iterator>
int main() {
std::vector<double> decimals = {1.1, 2.2, 3.3, 4.4};
std::copy(decimals.begin(), decimals.end(), std::ostream_iterator<double>(std::cout, " "));
return 0;
}
Output:
1.1 2.2 3.3 4.4
In this code, we include the necessary headers and create a vector of doubles called decimals
. The std::copy
function takes three arguments: the beginning and end of the vector and an output iterator that specifies where to send the output. In this case, we use std::ostream_iterator
, which writes to std::cout
, with a space as a delimiter. This method is efficient and works well for larger datasets, as it leverages the power of the Standard Template Library (STL).
Method 4: Using std::for_each
Another STL algorithm for doing the iteration and output operations in a single statement is for_each
. This method can apply a certain function object to each element of the specified range, which is a powerful tool to have. In this case, we pass the lambda
expression, which prints the elements to the output stream.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::for_each;
using std::vector;
int main() {
vector<int> int_vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
for_each(int_vec.begin(), int_vec.end(),
[](const int& n) { cout << n << "; "; });
cout << endl;
return EXIT_SUCCESS;
}
This code demonstrates the use of std::for_each
, an STL algorithm, to iterate over and print elements of a vector. It creates a vector of integers and uses for_each
with a lambda function to print each element followed by a semicolon. This approach provides a concise way to perform operations on container elements without explicit loops. It showcases how modern C++ features like lambda expressions can be combined with STL algorithms for clean and efficient code, especially useful for simple operations on collections.
Method 5: Using a Custom Function
For those who prefer a more modular approach, creating a custom function to print vector contents can be a great option. This method enhances code reusability and organization. Here’s how you can create such a function:
#include <iostream>
#include <vector>
template<typename T>
void printVector(const std::vector<T>& vec) {
for (const auto& element : vec) {
std::cout << element << " ";
}
}
int main() {
std::vector<char> letters = {'A', 'B', 'C', 'D'};
printVector(letters);
return 0;
}
Output:
A B C D
In this example, we define a template function called printVector
, which accepts a vector of any data type. Inside the function, we use a range-based for loop to print each element. In the main
function, we create a vector of characters called letters
and call printVector
to display its contents. This method not only makes your code cleaner but also allows you to print vectors of different types without rewriting the printing logic.
Conclusion
Printing the contents of a vector in C++ is a straightforward task that can be accomplished in several ways. Whether you choose to use a simple loop, a range-based for loop, the std::copy
function, or a custom function, each method has its advantages. Selecting the right approach depends on your specific needs and coding style. By mastering these techniques, you will enhance your ability to debug and visualize data in your C++ applications effectively.
FAQ
-
What is a vector in C++?
A vector is a dynamic array that can grow and shrink in size, allowing for flexible management of collections of data. -
How do I include vectors in my C++ program?
You can include vectors by adding#include <vector>
at the beginning of your code. -
Can I print vectors containing different data types?
Yes, you can use template functions to create a generic print function that can handle vectors of any data type. -
What is the difference between a for loop and a range-based for loop?
A standard for loop requires you to manage the loop counter and size, while a range-based for loop simplifies iteration without needing to manage indices. -
Are there performance differences between the printing methods?
While all methods are efficient, usingstd::copy
with an iterator may offer performance benefits for larger datasets due to its optimization in the STL.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook