How to Print Numbers With Specified Decimal Points in C++
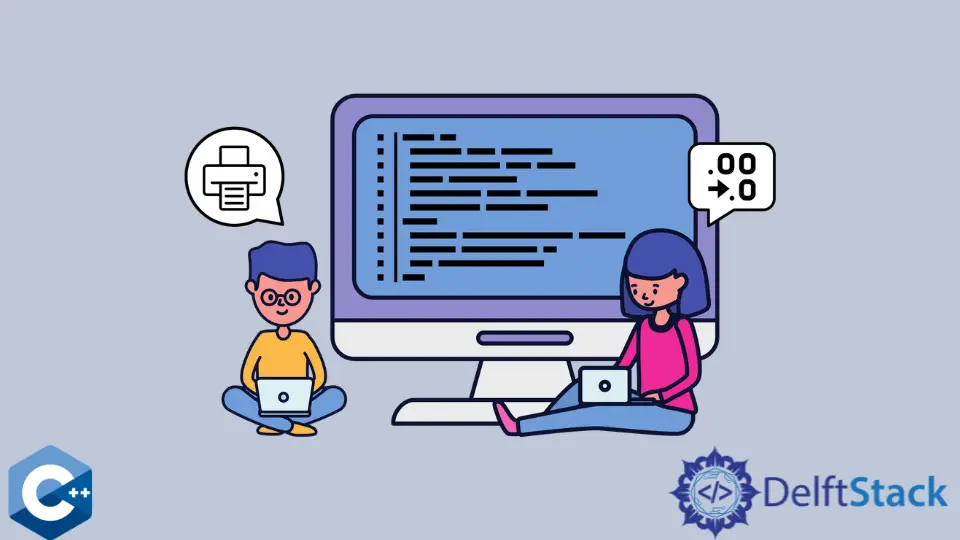
This article will introduce how to print the numbers with specified decimal points in C++.
Use std::fixed
and std::setprecision
Methods to Specify Precision
This method uses so-called I/O manipulators from the standard library defined in <iomanip>
header (see full list). These functions can modify the stream data and are mostly used for I/O formatting operations. In the first example, we only use the fixed
method, which modifies the default formatting so as the floating-point values are written using fixed-point notation. Note, though, the result always has a predefined precision of 6 points.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324,
10.222424, 6.3491092019, 110.12329403024,
92.001112, 0.000000124};
for (auto &d : d_vec) {
cout << fixed << d << endl;
}
return EXIT_SUCCESS;
}
Output:
123.231000
2.234300
0.324000
10.222424
6.349109
110.123294
92.001112
0.000000
Alternatively, we can fixate the decimal points after the comma and specify the precision value simultaneously. In the next code example, the fixed
function is called together with setprecision
, which itself takes int
value as a parameter.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setprecision;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324,
10.222424, 6.3491092019, 110.12329403024,
92.001112, 0.000000124};
for (auto &d : d_vec) {
cout << fixed << setprecision(3) << d << endl;
}
return EXIT_SUCCESS;
}
Output:
123.231
2.234
0.324
10.222
6.349
110.123
92.001
0.000
The previous code sample solves the initial problem, but the output seems quite mixed, and it’s hardly in readable formatting. We can use std::setw
and std::setfill
functions to display float values in a given vector aligned to the same comma position. To achieve this, we should call the setw
function to tell the cout
the maximum length of each output (we choose 8 in this case). Next, the setfill
function is called to specify a char
that will fill the padding places. Finally, we add previously used methods (fixed
and setprecision
) and output to console.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setfill;
using std::setprecision;
using std::setw;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324,
10.222424, 6.3491092019, 110.12329403024,
92.001112, 0.000000124};
for (auto &d : d_vec) {
cout << setw(8) << setfill(' ') << fixed << setprecision(3) << d << endl;
}
return EXIT_SUCCESS;
}
Output:
123.231
2.234
0.324
10.222
6.349
110.123
92.001
0.000
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook