How to Pass 2D Array to a Function in C++
-
Use
[]
Notation to Pass 2D Array as a Function Parameter -
Use
&
Notation to Pass 2D Array as a Function Parameter
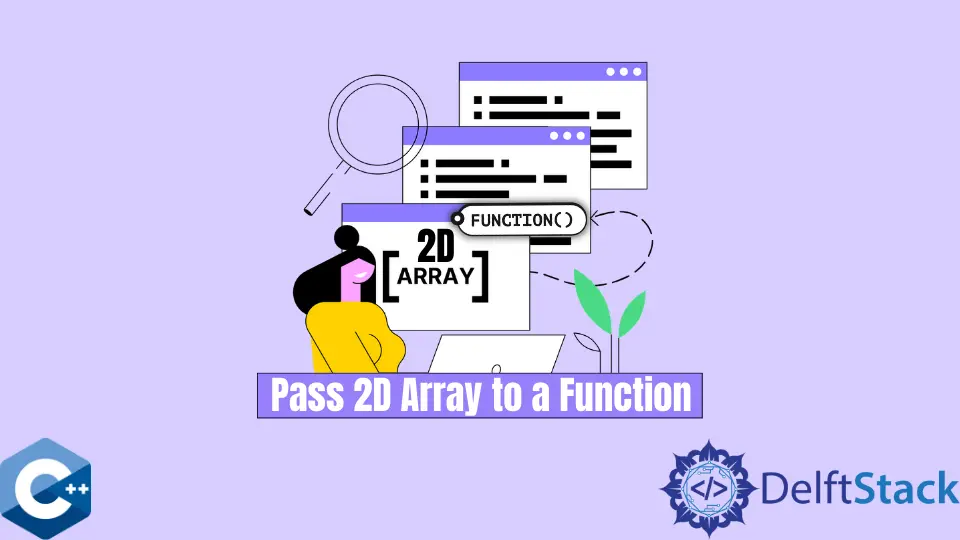
This article will introduce how to pass a 2D array as a function parameter in C++.
Use []
Notation to Pass 2D Array as a Function Parameter
To demonstrate this method, we define a fixed length 2-dimensional array named c_array
and to multiply its every element by 2 we will pass as a parameter to a MultiplyArrayByTwo
function. Notice that this function is a void
type and operates directly on the c_array
object. This way, we’ll directly access the multiplied version of 2D array from the main
routine.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int arr[][size], int len) {
for (int i = 0; i < len; ++i) {
for (int j = 0; j < len; ++j) {
arr[i][j] *= 2;
}
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array, size);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
input array
[ 1, 2, 3, 4, ]
[ 5, 6, 7, 8, ]
[ 9, 10, 11, 12, ]
[ 13, 14, 15, 16, ]
output array
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
Use &
Notation to Pass 2D Array as a Function Parameter
Alternatively, you can use &
reference notation to pass a 2D array as a parameter. Be aware, though, these 2 methods are compatible only with fixed length arrays declared on the stack. Notice that we changed the MultiplyArrayByTwo
function loop with range-based only for readability reasons.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
constexpr int size = 4;
void MultiplyArrayByTwo(int (&arr)[size][size]) {
for (auto& i : arr) {
for (int& j : i) j *= 2;
}
}
int main() {
int c_array[size][size] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
cout << "input array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
MultiplyArrayByTwo(c_array);
cout << "output array\n";
for (int i = 0; i < size; ++i) {
cout << " [ ";
for (int j = 0; j < size; ++j) {
cout << setw(2) << c_array[i][j] << ", ";
}
cout << "]" << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook