How to Loop Over an Array in C++
-
Use the
for
Loop to Iterate Over an Array - Use Range-based Loop to Iterate Over an Array
-
Use
std::for_each
Algorithm to Iterate Over an Array
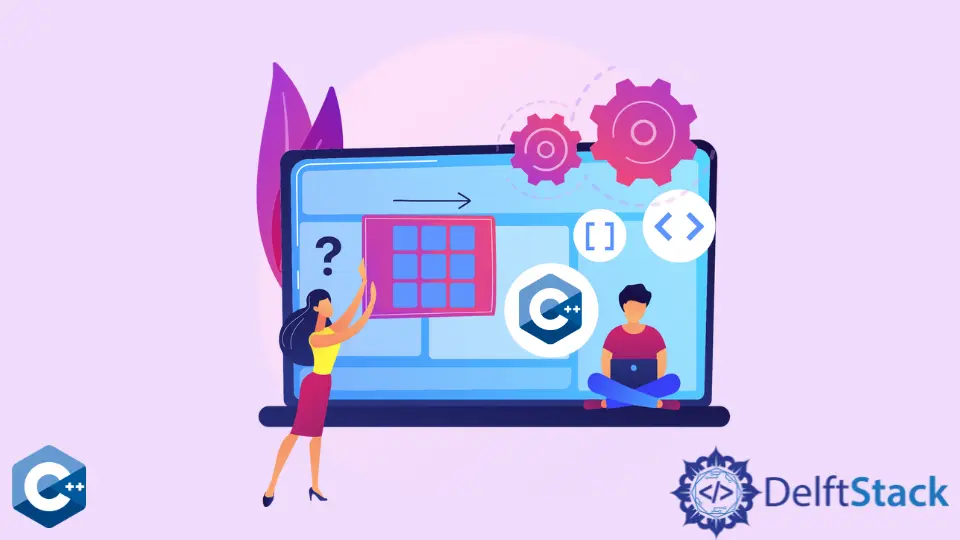
This article will explain how to iterate over an array using different methods in C++.
Use the for
Loop to Iterate Over an Array
The apparent method to iterate over array elements is a for
loop. It consists of a three-part statement, each separated with commas. First, we should initialize the counter variable - i
, which is executed only once by design. The next part declares a condition that will be evaluated on each iteration, and if the false is returned, the loop stops running. In this case, we compare the counter and the size of the array. The last part increments the counter, and it is also executed on each loop cycle.
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
array<string, 10> str_arr = {"Albatross", "Auklet", "Bluebird", "Blackbird",
"Cowbird", "Dove", "Duck", "Godwit",
"Gull", "Hawk"};
for (size_t i = 0; i < str_arr.size(); ++i) {
cout << str_arr[i] << " - ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
Albatross - Auklet - Bluebird - Blackbird - Cowbird - Dove - Duck - Godwit - Gull - Hawk -
Use Range-based Loop to Iterate Over an Array
The range-based loop is the readable version of the traditional for
loop. This method is a powerful alternative as it offers easy iteration over complicated containers and still maintains flexibility for accessing each element. The following code sample is the exact reimplementation of the previous example:
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
array<string, 10> str_arr = {"Albatross", "Auklet", "Bluebird", "Blackbird",
"Cowbird", "Dove", "Duck", "Godwit",
"Gull", "Hawk"};
for (auto &item : str_arr) {
cout << item << " - ";
}
cout << endl;
return EXIT_SUCCESS;
}
Use std::for_each
Algorithm to Iterate Over an Array
for_each
is a powerful STL algorithm to operate on range elements and apply custom defined functions. It takes range starting and last iterator objects as the first two parameters, and the function object as the third one. In this case, we declare a function object as lambda
expression, which directly outputs the calculated results to the console. Lastly, we can pass the custom_func
variable as an argument to for_each
method to operate on array elements.
#include <array>
#include <iostream>
using std::array;
using std::cin;
using std::cout;
using std::endl;
using std::for_each;
using std::string;
int main() {
array<int, 10> int_arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto custom_func = [](auto &i) { cout << i * (i + i) << "; "; };
;
for_each(int_arr.begin(), int_arr.end(), custom_func);
cout << endl;
return EXIT_SUCCESS;
}
Output:
2; 8; 18; 32; 50; 72; 98; 128; 162; 200;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook