How to Initialize a Vector in C++
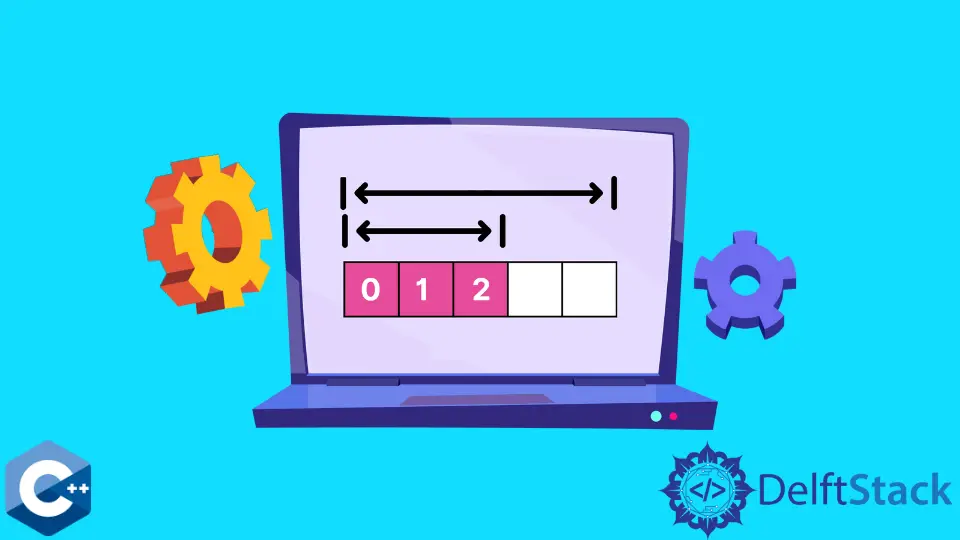
This article will explain how to initialize a vector with constant values in C++.
Use Initializer List Notation to Assign Constant Values to Vector
Elements in C++
This method has been supported since the C++11 style, and it is relatively the most readable way to constant initialize the vector
variable. The values are specified as a braced-init-list
which is preceded with the assignment operator. This notation is often called a copy-list-initialization.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<string> arr = {"one", "two", "three", "four"};
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
Output:
one; two; three; four;
The alternative method is to use the initializer-list constructor call when declaring the vector
variable as shown in the following code block:
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
vector<string> arr{"one", "two", "three", "four"};
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
A preprocessor macro is another way to implement the initializer list notation. In this example, we define constant element values as INIT
object-like macro and then assign it to the vector
variable as follows:
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
#define INIT \
{ "one", "two", "three", "four" }
int main() {
vector<string> arr = INIT;
for (const auto &item : arr) {
cout << item << "; ";
}
return EXIT_SUCCESS;
}
C++11 initializer list syntax is a quite powerful tool and it can be utilized to instanciate vector
of complex structures as demonstrated in the following code sample:
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
struct cpu {
string company;
string uarch;
int year;
} typedef cpu;
int main() {
vector<cpu> arr = {{"Intel", "Willow Cove", 2020},
{"Arm", "Poseidon", 2021},
{"Apple", "Vortex", 2018},
{"Marvell", "Triton", 2020}};
for (const auto &item : arr) {
cout << item.company << " - " << item.uarch << " - " << item.year << endl;
}
return EXIT_SUCCESS;
}
Output:
Intel - Willow Cove - 2020
Arm - Poseidon - 2021
Apple - Vortex - 2018
Marvell - Triton - 2020
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook