How to Find Substring in String in C++
-
Use
find
Method to Find Substring in a String in C++ -
Use
rfind
Method to Find Substring in a String in C++
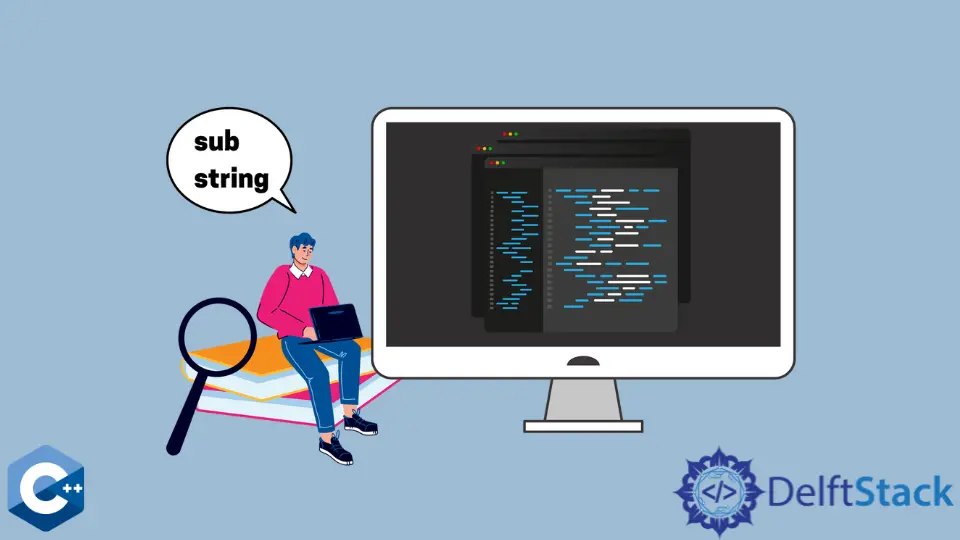
This article demonstrates methods to find a given substring in a string in C++.
Use find
Method to Find Substring in a String in C++
The most straightforward way of solving the issue is the find
function, which is a built-in string
method, and it takes another string
object as an argument.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
string str3 = "random s tring";
str1.find(str2) != string::npos
? cout << "str1 contains str2" << endl
: cout << "str1 does not contain str3" << endl;
str1.find(str3) != string::npos
? cout << "str1 contains str3" << endl
: cout << "str1 does not contain str3" << endl;
return EXIT_SUCCESS;
}
Output:
str1 contains str2
str1 does not contain str3
Alternatively, you can use find
to compare specific character ranges in two strings. To do this, you should pass the starting position and length of the range as arguments to the find
method:
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str3 = "random s tring";
constexpr int length = 6;
constexpr int pos = 0;
str1.find(str3.c_str(), pos, length) != string::npos
? cout << length << " chars match from pos " << pos << endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
Output:
6 chars match from pos 0
Use rfind
Method to Find Substring in a String in C++
rfind
method has a similar structure as find
. We can utilize rfind
to find the last substring or specify a certain range to match it with the given substring.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
str1.rfind(str2) != string::npos
? cout << "last occurrence of str3 starts at pos " << str1.rfind(str2)
<< endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
Output:
last occurrence of str3 starts at pos 22
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++