How to Declare a Global Variable in C++
- Declare a Global Variable in a Single Source File in C++
- Declare a Global Variable in Multiple Source Files in C++
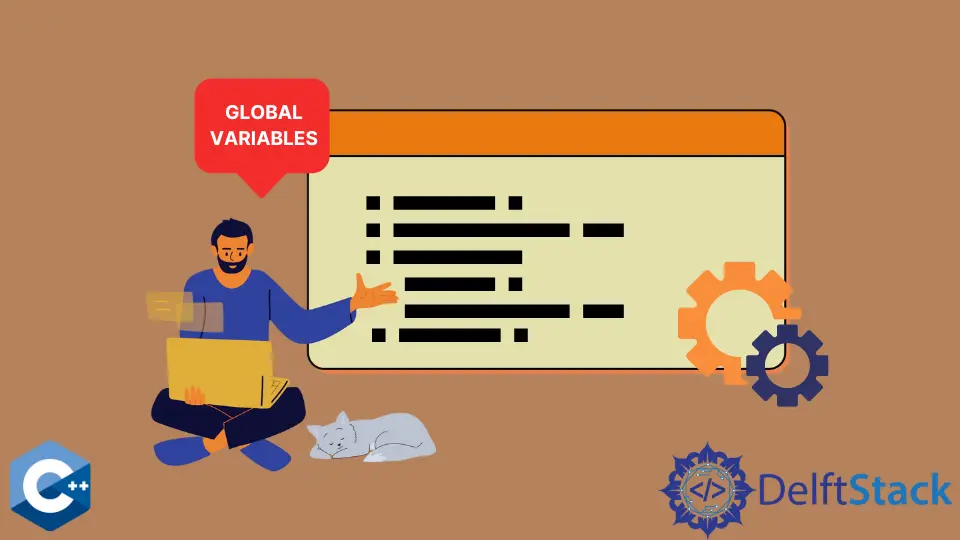
This article will explain several methods of how to declare a global variable in C++.
Declare a Global Variable in a Single Source File in C++
We can declare a global variable with the statement that is placed outside of every function. In this example, we assume the int
type variable and initialize it to an arbitrary 123
value. The global variable can be accessed from the scope of the main
function as well as any inner construct (loop or if statement) inside it. Modifications to the global_var
are also visible to each part of the main
routine, as demonstrated in the following example.
#include <iostream>
using std::cout;
using std::endl;
int global_var = 123;
int main() {
global_var += 1;
cout << global_var << endl;
for (int i = 0; i < 4; ++i) {
global_var += i;
cout << global_var << " ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
124
124 125 127 130
On the other hand, if additional functions are also defined in the same source file, they can directly access the global_var
value and modify it in the function scope. The global variables can also be declared with a const
specifier, which forces them to only be accessible through the current translation unit (source file with all included headers).
#include <iostream>
using std::cout;
using std::endl;
int global_var = 123;
void tmpFunc() {
global_var += 1;
cout << global_var << endl;
}
int main() {
tmpFunc();
return EXIT_SUCCESS;
}
Output:
124
Declare a Global Variable in Multiple Source Files in C++
Alternatively, there may be global variables declared in different source files, and needed to be accessed or modified. In this case, to access the global variable, it needs to be declared with an extern
specifier, which tells the compiler (more precisely the linker) where to look for the glob_var1
definition.
#include <iostream>
using std::cout;
using std::endl;
int global_var = 123;
extern int glob_var1; // Defined - int glob_var1 = 44; in other source file
void tmpFunc() {
glob_var1 += 1;
cout << glob_var1 << endl;
}
int main() {
tmpFunc();
return EXIT_SUCCESS;
}
There might be global variables declared with a static
specifier in the different source files in some cases. These variables are accessible only within the file where they are defined and can’t be reached from other files. If you still try to declare them with extern
in the current file, the compiler error will occur.
#include <iostream>
using std::cout;
using std::endl;
int global_var = 123;
extern int
glob_var2; // Defined - static int glob_var2 = 55; in other source file
void tmpFunc() {
glob_var2 += 1;
cout << glob_var2 << endl;
}
int main() {
tmpFunc();
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook