How to Declare 2D Array Using new in C++
-
Declare 2D Array to Access Elements With
arr[x][y]
Notation -
Declare 2D Array to Access Elements With
arr[]
Notation -
Use
vector
Container to Implicitly Allocate Dynamic 2D Array
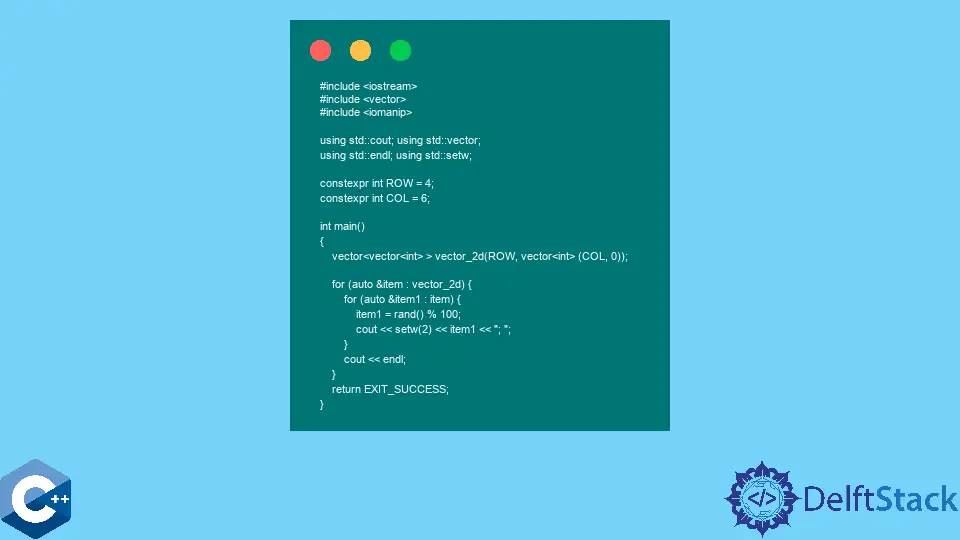
This article introduces multiple C++ methods to declare a 2D array dynamically using new
.
Declare 2D Array to Access Elements With arr[x][y]
Notation
This solution utilizes new
keyword so that the generated matrix structure can be accessed using array notation - [x][y]
. At first, we declare pointer to pointer to integer (int **
) variable and allocate int
pointer array of row size in the array. Next, we loop over this pointer array and allocate the int
array of column size each iteration. Lastly, when we finish the 2D array operation, we need to free up the allocated memory. Notice, that deallocation is done in the reverse order loop.
#include <iomanip>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
constexpr int ROW = 4;
constexpr int COL = 6;
int main() {
int **matrix = new int *[ROW];
for (int i = 0; i < ROW; ++i) {
matrix[i] = new int[COL];
}
for (int i = 0; i < ROW; ++i) {
for (int j = 0; j < COL; ++j) {
matrix[i][j] = rand() % 100;
cout << setw(2) << matrix[i][j] << "; ";
}
cout << endl;
}
for (int i = 0; i < ROW; ++i) {
delete matrix[i];
}
delete[] matrix;
return EXIT_SUCCESS;
}
Output:
83; 86; 77; 15; 93; 35;
86; 92; 49; 21; 62; 27;
90; 59; 63; 26; 40; 26;
72; 36; 11; 68; 67; 29;
Declare 2D Array to Access Elements With arr[]
Notation
This method uses a single array allocation, making it a more efficient method in terms of program execution speed. Consequently, we should use the []
notation and some arithmetic to access the elements. This method is the recommended way when the array size gets bigger, and calculations on its elements are quite intensive. Note also that deallocation becomes easier this way.
#include <iomanip>
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::setw;
constexpr int ROW = 4;
constexpr int COL = 6;
int main() {
int *matrix = new int[ROW * COL];
for (int i = 0; i < ROW; ++i) {
for (int j = 0; j < COL; ++j) {
matrix[j * ROW + i] = rand() % 100;
cout << setw(2) << matrix[j * ROW + i] << "; ";
}
cout << endl;
}
delete[] matrix;
return EXIT_SUCCESS;
}
Use vector
Container to Implicitly Allocate Dynamic 2D Array
Alternatively, we can construct a dynamic 2D array using a vector
container and without manual memory allocation/deallocation. In this example, we initialize a 4x6 vector_2d
array with each element of the value - 0
. The best part of this method is the flexibility of the iteration with the range-based loop.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int ROW = 4;
constexpr int COL = 6;
int main() {
vector<vector<int> > vector_2d(ROW, vector<int>(COL, 0));
for (auto &item : vector_2d) {
for (auto &item1 : item) {
item1 = rand() % 100;
cout << setw(2) << item1 << "; ";
}
cout << endl;
}
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook