How to Convert String to Lower Case in C++
-
Using the Standard Library Function
std::transform
- Manual Iteration with a Loop
-
Using the
std::locale
for Locale-Sensitive Lowercase Conversion - Conclusion
- FAQ
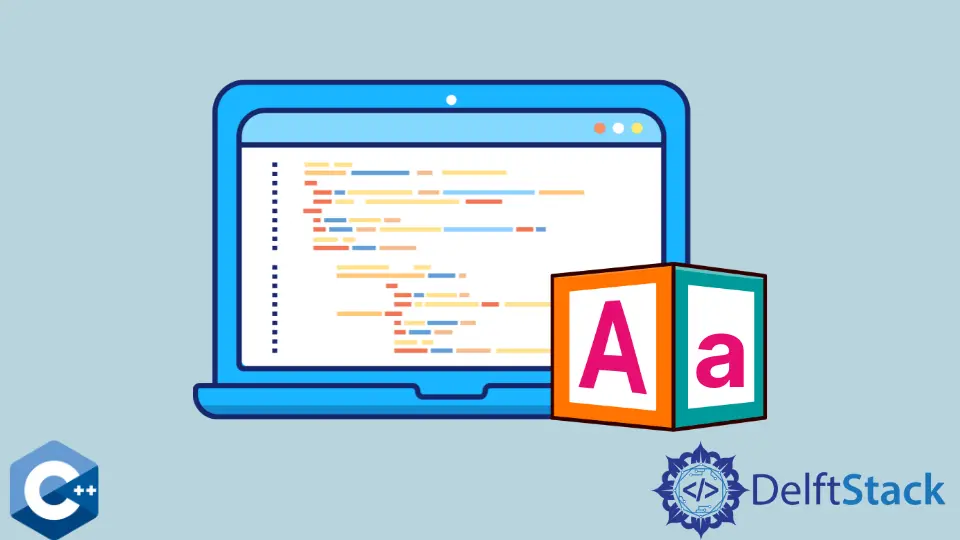
When working with strings in C++, you may often need to manipulate their cases for various reasons, such as ensuring uniformity in user input or preparing data for comparison. Converting a string to lowercase is a common operation that can be achieved using different methods.
In this article, we will explore how to convert a C++ std::string
to lowercase effectively. Whether you’re a beginner or an experienced programmer, you’ll find the methods outlined here straightforward and easy to implement. We will cover various techniques, including the use of standard library functions and manual iteration, to help you understand the best practices for string manipulation in C++. Let’s dive in!
Using the Standard Library Function std::transform
One of the most efficient ways to convert a string to lowercase in C++ is by utilizing the std::transform
function from the <algorithm>
header. This function allows us to apply a transformation to each character in the string, which is perfect for converting to lowercase.
Here’s how you can implement it:
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
std::cout << str << std::endl;
return 0;
}
Output:
hello, world!
In this example, we first include the necessary headers: <iostream>
, <string>
, and <algorithm>
. We define a string str
initialized with “Hello, World!”. The std::transform
function takes four arguments: the beginning and end of the string, the destination (which is the same as the original string in this case), and the transformation function ::tolower
. This function converts each character to lowercase. Finally, we print the modified string. This method is concise and leverages the power of the C++ Standard Library for efficient string manipulation.
Manual Iteration with a Loop
If you prefer a more hands-on approach, you can manually iterate through each character of the string and convert it to lowercase using a simple loop. This method provides a clear view of the conversion process, making it an excellent choice for beginners.
Here’s how you can do it:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
for (size_t i = 0; i < str.length(); ++i) {
str[i] = tolower(str[i]);
}
std::cout << str << std::endl;
return 0;
}
Output:
hello, world!
In this code snippet, we again include the necessary headers and initialize our string. We then use a for
loop to iterate through each character of the string. The tolower
function is called on each character, converting it to lowercase, and we assign the result back to the same position in the string. This method is straightforward and gives you full control over the string manipulation process, making it easy to understand how each character is processed.
Using the std::locale
for Locale-Sensitive Lowercase Conversion
In some cases, you might want to consider locale-sensitive conversions, especially when dealing with internationalization. The std::locale
class can be used to ensure that your string conversion respects the specific rules of a given locale.
Here’s an example:
#include <iostream>
#include <string>
#include <locale>
int main() {
std::string str = "Hello, World!";
std::locale loc;
for (char &c : str) {
c = std::tolower(c, loc);
}
std::cout << str << std::endl;
return 0;
}
Output:
hello, world!
In this example, we include the <locale>
header and define a locale
object. We then iterate through each character of the string using a range-based for loop. The std::tolower
function is called with two arguments: the character to convert and the locale. This ensures that the conversion adheres to the rules of the specified locale, which can be particularly useful for applications that need to support multiple languages or regional settings. This method combines the power of C++’s Standard Library with the flexibility of locale-based processing.
Conclusion
Converting a string to lowercase in C++ is a fundamental skill that can significantly enhance your string manipulation capabilities. Whether you choose to use the std::transform
function for its efficiency, manually iterate through the string for a better understanding, or utilize locale-sensitive conversions for internationalization, each method has its advantages. Understanding these techniques will not only improve your coding skills but also prepare you for more complex string handling tasks. Embrace these methods, and you’ll find string manipulation in C++ to be an enjoyable experience.
FAQ
-
How does
std::transform
work for string conversion?
std::transform
applies a transformation function to each element in a range, allowing for efficient string manipulation. -
Can I convert only part of a string to lowercase?
Yes, you can specify the range in thestd::transform
function to convert only a portion of the string. -
What is the difference between
tolower
andstd::tolower
?
tolower
is a C-style function, whilestd::tolower
is the C++ version that can take a locale as an argument. -
Is manual iteration slower than using
std::transform
?
Generally,std::transform
is optimized for performance, but for small strings, the difference may be negligible. -
Can I use these methods for other character types?
Yes, while the examples usechar
, you can adapt them for other character types, such aswchar_t
, with minor modifications.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++