How to Convert String to Char Array in C++
-
Use
std::basic_string::c_str
Method to Convert String to Char Array -
Use
std::vector
Container to Convert String to Char Array - Use Pointer Manipulation Operations to Convert String to Char Array
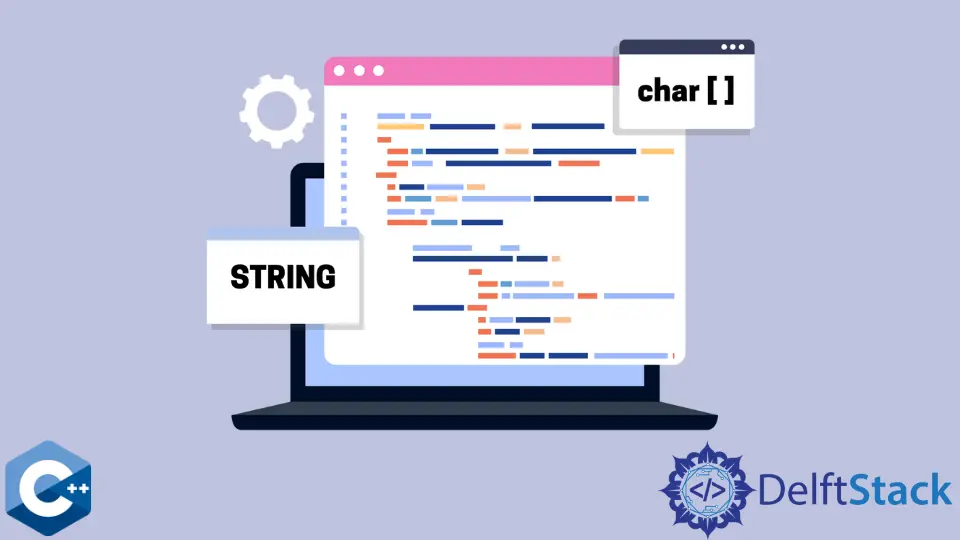
This article introduces multiple methods for converting string
data to char
array.
Use std::basic_string::c_str
Method to Convert String to Char Array
This version is the C++ way of solving the above problem. It uses the string
class built-in method c_str
which returns a pointer to a null-terminated char array.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*";
auto c_string = tmp_string.c_str();
cout << c_string << endl;
return EXIT_SUCCESS;
}
Output:
This will be converted to char*
If you intend to modify data stored at the pointer returned by the c_str()
method, first, you must copy the range to a different location with memmove
function and then operate on the char string as follows:
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*";
char *c_string_copy = new char[tmp_string.length() + 1];
memmove(c_string_copy, tmp_string.c_str(), tmp_string.length());
/* do operations on c_string_copy here */
cout << c_string_copy;
delete[] c_string_copy;
return EXIT_SUCCESS;
}
Note that, you can copy c_string
data using various functions like: memcpy
, memccpy
, mempcpy
, strcpy
or strncpy
, but bear in mind to read the manual pages and carefully consider their edge cases/bugs.
Use std::vector
Container to Convert String to Char Array
Another way is to store characters from string
into vector
container and then use its powerful built-in methods to manipulate data safely.
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string tmp_string = "This will be converted to char*\n";
vector<char> vec_str(tmp_string.begin(), tmp_string.end());
std::copy(vec_str.begin(), vec_str.end(),
std::ostream_iterator<char>(cout, ""));
return EXIT_SUCCESS;
}
Use Pointer Manipulation Operations to Convert String to Char Array
In this version, we define a char
pointer named tmp_ptr
and assign the first character’s address in tmp_string
to it.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*\n";
string mod_string = "I've been overwritten";
char *tmp_ptr = &tmp_string[0];
cout << tmp_ptr;
return EXIT_SUCCESS;
}
Remember though, modifying data at tmp_ptr
would also alter the tmp_string
value, which you can see by executing the following code sample:
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*\n";
string mod_string = "I've been overwritten";
char *tmp_ptr = &tmp_string[0];
memmove(tmp_ptr, mod_string.c_str(), mod_string.length());
cout << tmp_string;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++